


Python program to convert array to string and concatenate elements using specified characters
数组是一种数据结构,由相同数据类型的元素集合组成,每个元素由索引标识。
[2, 4, 0, 5, 8]
Python中的数组
Python 没有自己的数据结构来表示数组。相反,我们可以使用列表数据结构来表示数组。在这里,我们将使用列出一个数组 -
[10, 4, 11, 76, 99]
在这篇文章中,我们将编写一个Python程序,将一个数组转换为字符串,并使用指定的字符连接元素。
输入输出场景
假设我们有一个包含整数值的输入数组。并且在输出中将有所有数组元素都与指定字符连接的字符串。
Input array: [1, 5, 3, 6] Output: 1a5a3a6
这里将数组元素1、5、3、6与字符a连接起来。让我们考虑另一个包含整数值的数组。
Input array: [1, 2, 3, 10, 20] Output: 1P2P3P10P20
在这里,数组元素1、2、3、10、20与字符P连接在一起。我们将使用内置的字符串join()方法来将数组元素与指定字符连接起来。
Join() 方法
join()方法从可迭代对象中取出元素,并使用一个字符串将它们连接起来。然后通过使用给定的字符串将可迭代对象的所有元素连接起来,并返回一个字符串。
语法
string.join(iterable)
该函数接受一个可迭代对象(可以是列表、元组、字符串、字典或集合)。
用户定义函数
我们将使用 python 中的 def 关键字定义一个用户定义函数,将数组元素转换为字符串。并将每个元素与指定的字符连接起来。
示例
在此示例中,我们将定义一个 toString 函数来将数组元素转换为字符串。在函数中,我们将声明一个空列表,用于存储字符串转换后的元素。通过使用 string.join() 方法,我们将使用指定字符连接数组元素。
def toString(L): r = [] for i in L: r.append(str(i)) return r # creating array arr = [1, 2, 3, 4, 5] print ("The original array is: ", arr) print() specified_char = "a" result = specified_char.join(toString(arr)) print ("The result is: ", result)
输出
The original array is: [1, 2, 3, 4, 5] The result is: 1a2a3a4a5
我们已成功将数组转换为字符串,并使用指定字符(“a”)连接元素。
使用Map()函数
map() 函数采用一个可迭代对象来将该函数应用于可迭代对象的每个项目。并返回一个由应用函数产生的迭代器。
示例
这里,我们将使用map函数将数组元素转换为字符串数据类型。
# creating array arr = [101,102,103,104,105] print ("The original array is: ", arr) print() specified_char = "a" result = specified_char.join(list(map(str, arr))) print ("The result is: ", result)
输出
The original array is: [101, 102, 103, 104, 105] The result is: 101a102a103a104a105
在此示例中,我们将数组转换为字符串,并使用 map() 和 join() 函数将元素与字符“a”连接起来。
使用 Lambda
Lambda表达式用于创建匿名函数,只不过我们可以为函数定义一个未命名的对象。
示例
在此示例中,我们将使用 lambda 创建一个匿名函数,将数组元素转换为字符串。然后使用 join() 方法连接字符串转换后的元素。
# creating array arr = [101,102,103,104,105] print ("The original array is: ", arr) print() specified_char = "a" temp = lambda x : (str(i) for i in x) result = specified_char.join(temp(arr)) print ("The result is: ", result)
输出
The original array is: [101, 102, 103, 104, 105] The result is: 101a102a103a104a105
变量 temp 保存着将数组元素的数据类型转换为字符串数据类型的lambda表达式。
示例
在这个例子中,我们将使用另一个包含浮点数的数组。
# creating array arr = [1.1,1.2,1.3,1.4,1.5] print ("The original array is: ", arr) print() specified_char = "$" temp = lambda x : (str(i) for i in x) result = specified_char.join(temp(arr)) print ("The result is: ", result)
输出
The original array is: [1.1, 1.2, 1.3, 1.4, 1.5] The result is: 1.1$1.2$1.3$1.4$1.5
这些是将数组转换为字符串并使用指定字符连接元素的一些方法。
The above is the detailed content of Python program to convert array to string and concatenate elements using specified characters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


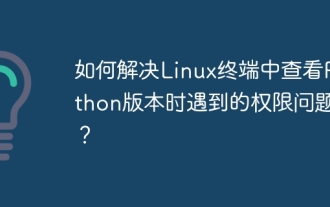
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
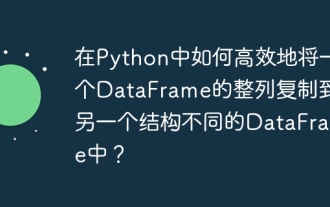
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
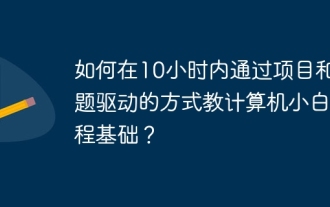
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
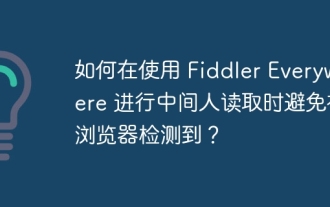
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
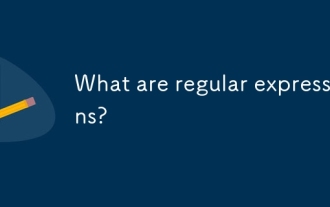
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
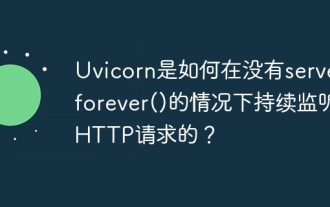
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
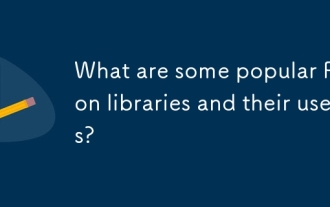
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
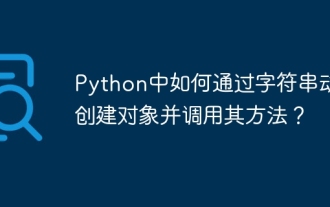
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
