In C programming, working with 2D arrays at runtime
Question
Write a C program that uses runtime compilation to calculate the sum and product of all elements in a two-dimensional array.
Solution
Run-time compilation or initialization is also known as dynamic allocation. Allocating memory at execution time (runtime) is called dynamic memory allocation.
The functions calloc() and malloc() support dynamic memory allocation.
The functions calloc() and malloc() support dynamic memory allocation. p>
In this program, we will calculate the sum of all elements and the product of all elements of a 2D array at runtime.
Logic used to calculate the sum of all elements in a two-dimensional array-
printf("Sum array is : </p><p>"); for(i=0;i<2;i++){ for(j=0;j<3;j++){ sum[i][j]=A[i][j]+B[i][j]; printf("%d\t",sum[i][j]); } printf("</p><p>"); }
Logic used to calculate the product of all elements in a two-dimensional array-
printf("Product array is : </p><p>"); for(i=0;i<2;i++){ for(j=0;j<3;j++){ product[i][j]=A[i][j]*B[i][j]; printf("%d\t",product[i][j]); } printf("</p><p>"); } }
Example
Example demonstration
#include<stdio.h> void main(){ //Declaring the array - run time// int A[2][3],B[2][3],i,j,sum[i][j],product[i][j]; //Reading elements into the array's A and B using for loop// printf("Enter elements into the array A: </p><p>"); for(i=0;i<2;i++){ for(j=0;j<3;j++){ printf("A[%d][%d] :",i,j); scanf("%d",&A[i][j]); } printf("</p><p>"); } for(i=0;i<2;i++){ for(j=0;j<3;j++){ printf("B[%d][%d] :",i,j); scanf("%d",&B[i][j]); } printf("</p><p>"); } //Calculating sum and printing output// printf("Sum array is : </p><p>"); for(i=0;i<2;i++){ for(j=0;j<3;j++){ sum[i][j]=A[i][j]+B[i][j]; printf("%d\t",sum[i][j]); } printf("</p><p>"); } //Calculating product and printing output// printf("Product array is : </p><p>"); for(i=0;i<2;i++){ for(j=0;j<3;j++){ product[i][j]=A[i][j]*B[i][j]; printf("%d\t",product[i][j]); } printf("</p><p>"); } }
Output
Enter elements into the array A: A[0][0] :A[0][1] :A[0][2] : A[1][0] :A[1][1] :A[1][2] : B[0][0] :B[0][1] :B[0][2] : B[1][0] :B[1][1] :B[1][2] : Sum array is : 000 000 Product array is : 000 000
The above is the detailed content of In C programming, working with 2D arrays at runtime. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


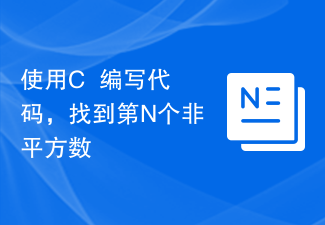
We all know numbers that are not the square of any number, such as 2, 3, 5, 7, 8, etc. There are N non-square numbers, and it is impossible to know every number. So, in this article, we will explain everything about squareless or non-square numbers and ways to find the Nth non-square number in C++. Nth non-square number If a number is the square of an integer, then the number is called a perfect square. Some examples of perfect square numbers are -1issquareof14issquareof29issquareof316issquareof425issquareof5 If a number is not the square of any integer, then the number is called non-square. For example, the first 15 non-square numbers are -2,3,5,6,
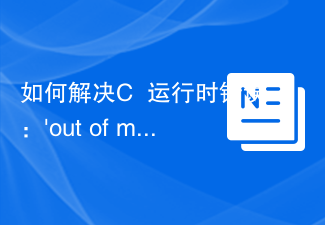
How to solve C++ runtime error: 'outofmemory'? In C++ programming, facing runtime errors is a very common thing. One of them is the "outofmemory" error, which is an out-of-memory error. This error usually occurs when the program needs to allocate more memory space, but the system does not have enough memory to meet the demand. So, how should we solve this problem? This article will provide some solutions to handle this situation. First, the simplest way is to adjust the code

How to convert a php array from two dimensions to a one-dimensional array: 1. Use loop traversal to traverse the two-dimensional array and add each element to the one-dimensional array; 2. Use the "array_merge" function to merge multiple arrays into An array. Pass the two-dimensional array as a parameter to the "array_merge" function to convert it into a one-dimensional array; 3. Using the "array_reduce" function, you can process all the values in the array through a callback function and finally return a result.
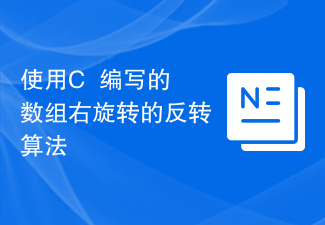
In this article, we will learn about the reversal algorithm to rotate a given array to the right by k elements, for example −Input:arr[]={4,6,2,6,43,7,3,7},k= 4Output:{43,7,3,7,4,6,2,6}Explanation:Rotatingeachelementofarrayby4-elementtotherightgives{43,7,3,7,4,6,2,6}.Input:arr[]={8 ,5,8,2,1,4,9,3},k=3Output:{4,9,3,8,5,8,2,1} Find the solution
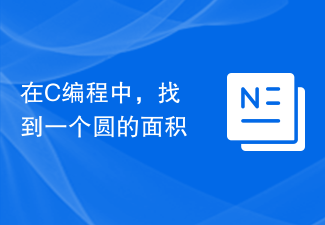
A circle is a closed figure. All points on a circle are equidistant from a point inside the circle. The center point is called the center of the circle. The distance from a point to the center of a circle is called the radius. Area is a quantitative representation of the span of dimensions of a closed figure. The area of a circle is the area enclosed within the dimensions of the circle. The formula to calculate the area of a circle, Area=π*r*r To calculate the area, we give the radius of the circle as input, we will use the formula to calculate the area, algorithm STEP1: Takeradiusasinputfromtheuserusingstdinput.STEP2: Calculatetheareaofcircleusing, area=(
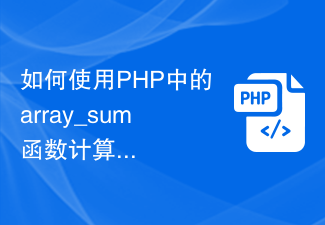
In PHP programming, the array_sum function is a very practical function that can calculate the sum of all elements in an array. However, when we need to calculate the sum of a column of elements in a two-dimensional array, we may encounter some trouble. This article will introduce how to use the array_sum function in PHP to calculate the sum of elements in a column of a two-dimensional array. First, we need to understand the concept of two-dimensional arrays. A two-dimensional array is an array containing multiple arrays, which can be regarded as a table. Each array represents a table
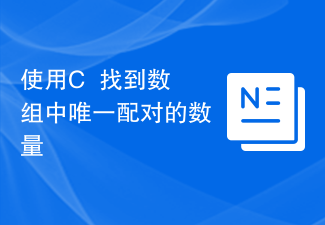
We need proper knowledge to create several unique pairs in array syntax of C++. While finding the number of unique pairs, we count all the unique pairs in the given array i.e. all possible pairs can be formed where each pair should be unique. For example -Input:array[]={5,5,9}Output:4Explanation:Thenumberoffalluniquepairsare(5,5),(5,9),(9,5)and(9,9).Input:array[]= {5,4,3,2,2}Output:16 Ways to Find Solution There are two ways to solve this problem, they are −
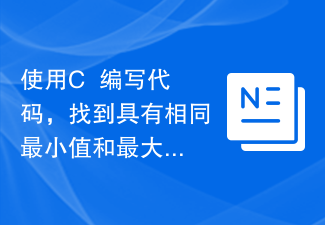
In this article, we will use C++ to solve the problem of finding the number of subarrays whose maximum and minimum values are the same. The following is an example of the problem −Input:array={2,3,6,6,2,4,4,4}Output:12Explanation:{2},{3},{6},{6},{2 },{4},{4},{4},{6,6},{4,4},{4,4}and{4,4,4}arethesubarrayswhichcanbeformedwithmaximumandminimumelementsame.Input:array={3,3, 1,5,
