Python script to generate dotted text for any image
In the digital age, manipulating images and creating artistic effects has become a common practice. An interesting effect is to generate dotted text from images. This process involves converting the pixels of an image into a pattern of dots, creating an interesting visual representation of the text.
In this blog post, we will explore how to create a Python script that can generate dotted text from any given image. By leveraging the power of Python and some great libraries, we can automate the process and generate stunning dotted text effects with ease.
Understanding dotted text
Before we get started, let’s take a moment to understand what dotted text is and why it can be an interesting visual effect. Dotted text is a technique that replaces image pixels with dots, forming the shape and outline of the original text.
This effect creates a unique and eye-catching visual representation of text, reminiscent of halftone patterns. It adds a playful and artistic touch to images, making them visually appealing and attractive.
The process of generating dotted text involves converting the image to grayscale, determining the density of dots based on pixel values, and strategically placing the dots to represent the text. The result is a mesmerizing transformation of the image, where the points form the outline and texture of the text.
Implementing Python script
To generate dotted text from images, we will use Python and some libraries that provide image processing functions. Specifically, we will leverage the following libraries -
PIL (Python Image Library) − A powerful image processing and manipulation library.
NumPy− A library for efficient numerical operations, we will use it to perform array operations.
Matplotlib− A plotting library that will help us visualize the generated dotted and line text.
Let's install the necessary libraries first. Open a terminal or command prompt and run the following command−
pip install Pillow numpy matplotlib
After installing the library, we can start implementing the script. Create a new Python file, for example dotted_text_generator.py, then we first import the required modules −< /p>
from PIL import Image, ImageDraw import numpy as np import matplotlib.pyplot as plt
Next, we need to define a function that takes an image file path as input and generates dotted text. We name this function generate_dotted_text −
def generate_dotted_text(image_path): # Load the image using PIL image = Image.open(image_path).convert("L") # Convert the image to a NumPy array image_array = np.array(image) # Perform necessary operations to generate dotted text # Create a new image for the dotted text dotted_text_image = Image.new("L", image.size) # Convert the dotted text image back to PIL format dotted_text_image_pil = Image.fromarray(dotted_text_image) # Save the dotted text image dotted_text_image_pil.save("dotted_text.png") # Display the original image and the generated dotted text fig, axes = plt.subplots(1, 2) axes[0].imshow(image, cmap="gray") axes[0].set_title("Original Image") axes[0].axis("off") axes[1].imshow(dotted_text_image, cmap="gray") axes[1].set_title("Dotted Text") axes[1].axis("off") plt.show()
In this code snippet, we use PIL to load the image and convert it to grayscale using the Convert("L") method. We then convert the image to a NumPy array for efficient processing. The actual implementation of generating dotted text is omitted here for the sake of brevity, but it typically involves analyzing pixel values, determining point locations, and creating an image of dotted text.
After generating the dotted text, we use Image.new() to create a new image and convert it back to PIL format. We save the dotted text image as "dotted_text.png". Finally, we use Matplotlib to display the original image and the generated dotted text side by side for comparison.
To use the generate_dotted_text function, we can call it with the path of the input image file−
generate_dotted_text("input_image.png")
Make sure to replace "input_image.png" with the actual path to the image file. When you run the script, it generates an image of dotted text and uses Matplotlib to display it along with the original image.
In the next section, we will provide some additional tips and ideas to further enhance and customize dotted text generation.
Enhancement and Customization
A basic implementation of a Python script that generates point text from images is a good starting point. However, there are many ways to enhance and customize the script to meet your specific needs. Let's explore some of the possibilities -
Font selection− By default, the script uses simple points as markers for point text. However, you can customize the markup by using different Unicode characters or symbols. PIL's ImageDraw module provides various methods for drawing shapes, lines, and text. You can experiment with different markup and font styles to create visually appealing dotted text.
Coloring− You can add color to dotted text by modifying the script instead of using grayscale. One way is to use the ImageDraw.text method and specify the fill color parameter. You can generate colorful dotted text by selecting a color palette or assigning a random color to each dot.
Point size and density −You can control the size and density of points in the generated text. Adjusting dot size can produce different visual effects, while modifying dot density can make text appear more or less dotted. Experiment with different point sizes and densities to find the look you want.
Background Options − Currently, the script generates dotted text on a transparent background. However, you can change the background color or even use a background image by modifying the code. This allows you to integrate dotted text into a variety of designs or images.
Custom input and output paths − You can modify the generate_dotted_text function to accept these paths as parameters instead of input and output images in the script The path is hardcoded. This provides flexibility and allows you to generate dotted line text from different input images and use custom names or save them in a specific directory.
in conclusion
In this article, we explored how to create a Python script to generate dotted text from an image. We first discuss the motivation behind the script and its potential applications. We then detail the implementation process, which involves using PIL (Python Imaging Library) to load images, convert them to grayscale, and generate dotted text based on pixel intensity.
Throughout this article, we examine key concepts and techniques involved in scripting, such as image processing, text generation, and file processing. We provide detailed explanations and accompanying code examples to ensure a clear understanding of the steps involved.
Additionally, we discuss potential enhancements and customizations that can be made to the script, such as font selection, shading, point size and density adjustments, background options, and custom input/output paths. These options allow you to tailor the script to your specific needs and create visually appealing dotted text effects.
The above is the detailed content of Python script to generate dotted text for any image. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
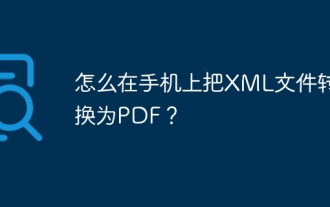
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
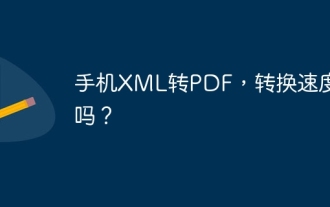
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
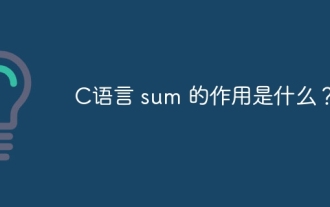
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
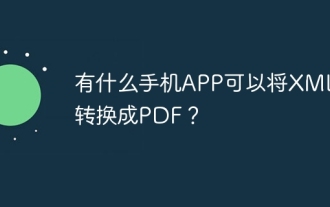
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
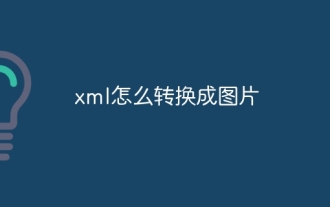
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
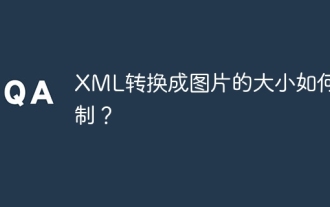
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
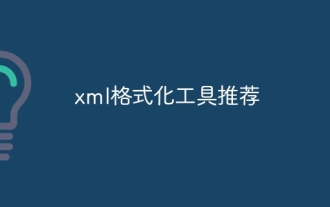
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
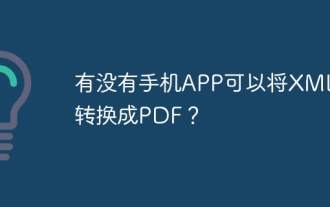
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
