Different storage classes in C language
Question
What are the different storage classes in C language? Interpret them with programs.
Solution
A storage class is defined as the scope and life cycle of a variable or function that exists in a C program.
Storage classes
The storage classes in C language are as follows:
- auto
- extern
- static
- register
Automatic variables/local variables
- Keywords - auto
- is also called local variable
-
Scope -
The scope of local variables is limited to the block in which they are declared.
These variables are declared inside the block.
- Default Value - Garbage Value
Example
Demonstration
#include<stdio.h> void main (){ auto int i=1;{ auto int i=2;{ auto int i=3; printf ("%d",i); } printf("%d", i); } printf("%d", i); }
Output
3 2 1
Global variables/external variables
- Keywords - extern
These variables are declared outside the block so they are also Global variables are called
#Scope - The scope of a global variable is available throughout the program.
- Default - Zero
Example
Live Demonstration
#include<stdio.h> extern int i =1; /* this ‘i’ is available throughout program */ main (){ int i = 3; /* this ‘i' available only in main */ printf ("%d", i); fun (); } fun (){ printf ("%d", i); }
Output
31
static variable
- Keyword - static
- Scope - The advantage of a static scope variable is that it is used throughout the program as well as Preserves its value between function calls.
- Static variables are initialized only once.
- Default value - zero
- li>
Example
Live demonstration
#include<stdio.h> main (){ inc (); inc (); inc (); } inc (){ static int i =1; printf ("%d", i); i++; }
Output
1 2 3
Register variable
- Keyword − register
The value of the register variable is stored in the CPU register rather than in memory , normal variables are stored in memory.
Register is a temporary storage unit in the CPU.
Example
Demonstration
#include<stdio.h> main (){ register int i; for (i=1; i< =5; i++) printf ("%d",i); }
Output
1 2 3 4 5
The above is the detailed content of Different storage classes in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
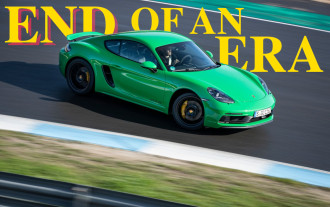
We'vepreviouslyreportedonleaksofanupcomingelectricPorscheBoxster,andPorschehaspreviouslycommittedtoEVsmakingup80%ofsalesby2030andconfirmedthatelectricBoxsterandCaymanmodelswouldbeintroducedalongsideitsregularpetrol-p
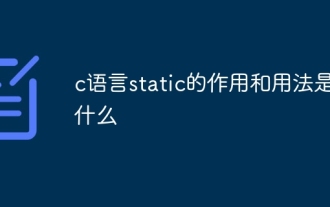
The role and usage of static in C language: 1. Variable scope; 2. Life cycle; 3. Internal function; 4. Modify global variables; 5. Modify function; 6. Other uses; Detailed introduction: 1. Variable scope, when If there is the static keyword before a variable, then the scope of the variable is limited to the file in which it is declared. In other words, the variable is a "file-level scope", which is very useful for preventing the "duplicate definition" problem of variables; 2. Life cycle, static variables are initialized once when the program starts executing, and destroyed when the program ends, etc.
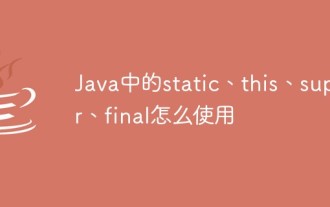
1. static Please look at the following program first: publicclassHello{publicstaticvoidmain(String[]args){//(1)System.out.println("Hello, world!");//(2)}} Have seen this Segment programs are familiar to most people who have studied Java. Even if you have not learned Java but have learned other high-level languages, such as C, you should be able to understand the meaning of this code. It simply outputs "Hello, world" and has no other use. However, it shows the main purpose of the static keyword.
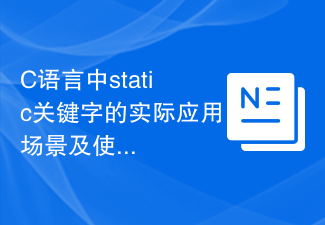
Practical application scenarios and usage skills of the static keyword in C language 1. Overview static is a keyword in C language, used to modify variables and functions. Its function is to change its life cycle and visibility during program running, making variables and functions static. This article will introduce the actual application scenarios and usage techniques of the static keyword, and illustrate it through specific code examples. 2. Static variables extend the life cycle of variables. Using the static keyword to modify local variables can extend their life cycle.
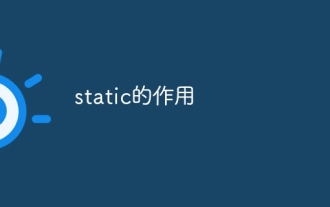
The functions of static: 1. Variables; 2. Methods; 3. Classes; 4. Other uses; 5. Multi-threaded environment; 6. Performance optimization; 7. Singleton mode; 8. Constants; 9. Local variables; 10. Memory Layout optimization; 11. Avoid repeated initialization; 12. Use in functions. Detailed introduction: 1. Variables, static variables. When a variable is declared as static, it belongs to the class level, not the instance level, which means that no matter how many objects are created, only one static variable exists, and all objects share this Static variables and so on.
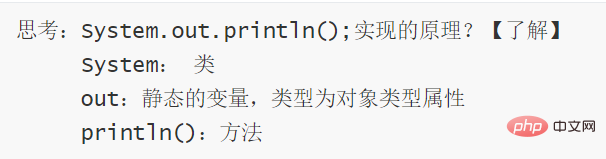
Modifier abstract (abstract) 1. Abstract can modify a class (1) The class modified by abstract is called an abstract class (2) Syntax: abstractclass class name {} (3) Features: Abstract classes cannot create objects separately, but they can be declared Reference the abstract class name reference name; (4) Abstract classes can define member variables and member methods (5) Abstract classes have constructors. When used to create subclass objects, jvm creates a parent class object by default; abstract constructor methods apply Applied when jvm creates parent class object. 2. Abstract can modify methods (1) The method modified by asbtract is called an abstract method (2) Syntax: access modifier abstract return value
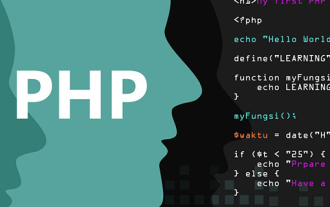
The "static" in php static static methods means that these properties and methods can be called directly without instantiating the class; static is a keyword used to modify the properties and methods of the class, and its usage syntax is such as "class Foo {public static $my_static = 'hello';}".
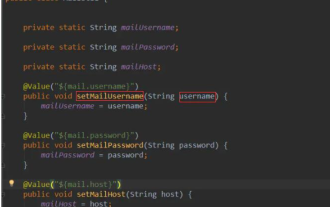
Springboot reads the pro file and injects static static variables mailConfig.properties#Server mail.host=smtp.qq.com#Port number mail.port=587#Email account mail.userName=hzy_daybreak_lc@foxmail.com#Email authorization code mail.passWord =vxbkycyjkceocbdc#Time delay mail.timeout=25000#Sender mail.emailForm=hzy_daybreak_lc@foxmail.com#Sender mai
