C program for recursive bubble sort
# Bubble sort is one of the simplest sorting algorithms used to sort data by comparing adjacent elements. All elements are compared in stages. The first stage puts the largest value at the end, the second stage puts the second largest element at the second to last position, and so on until the complete list is sorted.
Bubble sort algorithm
- ##int arr[5]= { 5,4,2,1,3 };
- int i, j ;
- Traverse from index i=0 to i
- Traverse from index j=0 to array size - i - 1
- If arr[i]>arr[j] swap arr[i] with arr[j]
- End
- If the array length is 1, return
- Traverse the array once, fixing the largest element at the end
p>
- The rest of the array recursively executes step 2 except the last element
Input − Arr[] = { 5,7,2,3, 1,4}; length=6
Output − Sorted array: 1 2 3 4 5 7
Description −
First Pass 5 7 2 3 1 4 → swap → 5 2 7 3 1 4 5 2 7 3 1 4 → swap → 5 2 3 7 1 4 5 2 3 7 1 4 → swap → 5 2 3 1 7 4 5 2 3 1 7 4 → swap → 5 2 3 1 4 7 Second Pass 5 2 3 1 4 7 → swap → 2 5 3 1 4 7 2 5 3 1 4 7 → swap → 2 3 5 1 4 7 2 3 5 1 4 7 → swap → 2 3 1 5 4 7 2 3 1 5 4 7 → swap → 2 3 1 4 5 7 Third Pass 2 3 1 4 5 7 → swap → 2 1 3 4 5 7 2 1 3 4 5 7 no swap Fourth Pass 2 1 3 4 5 7 → swap → 1 2 3 4 5 7 1 2 3 4 5 7 no swap in further iterations
Input− Arr[] = { 1, 2, 3, 3, 2 };
Output− Sorted array: 1 2 2 3 3
Explanation -
First Pass 1 2 3 3 2 → swap → 1 2 3 2 3 1 2 3 2 3 → swap → 1 2 2 3 3 1 2 2 3 3 no swap in further iterations Second Pass 1 2 2 3 3 no swap in further iterations
< /p>
- Take input array Arr[] and length as the number of elements in it.
- Function recurbublSort(int arr[], int len) gets the array and its length, and uses bubble sort to recursively sort the array.
- Get the variable temp. < li>If the array length is 1, void is returned.
- Otherwise use a single for loop to iterate over the array and, for each element arr[i]>arr[i 1], swap the elements.
- Set temp=arr[i], arr[i]=arr[i 1] and arr[i 1]=temp.
< /p>
- Now reduce the length by 1 since the previous loop placed the largest element at the last position.
- Recursively call recurbublSort(arr,len).
- At the end of all calls, when len becomes 1, we exit the recursion and sort the array. < /li>
- Print the sorted array in main.
#include <stdio.h>
void recurbublSort(int arr[], int len){
int temp;
if (len == 1){
return;
}
for (int i=0; i<len-1; i++){
if (arr[i] > arr[i+1]){
temp=arr[i];
arr[i]=arr[i+1];
arr[i+1]=temp;
}
}
len=len-1;
recurbublSort(arr, len);
}
int main(){
int Arr[] = {21, 34, 20, 31, 78, 43, 66};
int length = sizeof(Arr)/sizeof(Arr[0]);
recurbublSort(Arr, length);
printf("Sorted array : ");
for(int i=0;i<length;i++){
printf("%d ",Arr[i]);
}
return 0;
}
Copy after login
OutputIf we run the above code it will generate the following output#include <stdio.h> void recurbublSort(int arr[], int len){ int temp; if (len == 1){ return; } for (int i=0; i<len-1; i++){ if (arr[i] > arr[i+1]){ temp=arr[i]; arr[i]=arr[i+1]; arr[i+1]=temp; } } len=len-1; recurbublSort(arr, len); } int main(){ int Arr[] = {21, 34, 20, 31, 78, 43, 66}; int length = sizeof(Arr)/sizeof(Arr[0]); recurbublSort(Arr, length); printf("Sorted array : "); for(int i=0;i<length;i++){ printf("%d ",Arr[i]); } return 0; }
Sorted array: 20 21 31 34 43 66 78
The above is the detailed content of C program for recursive bubble sort. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The recursion depth of C++ functions is limited, and exceeding this limit will result in a stack overflow error. The limit value varies between systems and compilers, but is usually between 1,000 and 10,000. Solutions include: 1. Tail recursion optimization; 2. Tail call; 3. Iterative implementation.
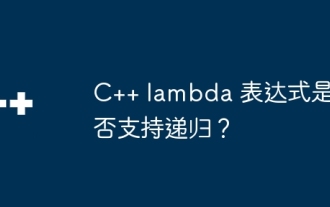
Yes, C++ Lambda expressions can support recursion by using std::function: Use std::function to capture a reference to a Lambda expression. With a captured reference, a Lambda expression can call itself recursively.

The recursive algorithm solves structured problems through function self-calling. The advantage is that it is simple and easy to understand, but the disadvantage is that it is less efficient and may cause stack overflow. The non-recursive algorithm avoids recursion by explicitly managing the stack data structure. The advantage is that it is more efficient and avoids the stack. Overflow, the disadvantage is that the code may be more complex. The choice of recursive or non-recursive depends on the problem and the specific constraints of the implementation.
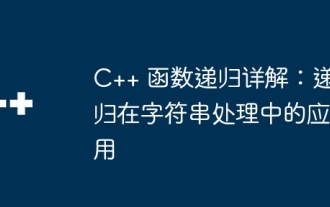
A recursive function is a technique that calls itself repeatedly to solve a problem in string processing. It requires a termination condition to prevent infinite recursion. Recursion is widely used in operations such as string reversal and palindrome checking.
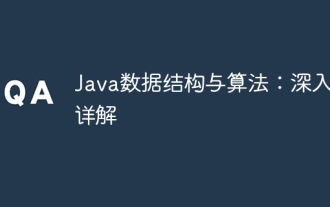
Data structures and algorithms are the basis of Java development. This article deeply explores the key data structures (such as arrays, linked lists, trees, etc.) and algorithms (such as sorting, search, graph algorithms, etc.) in Java. These structures are illustrated through practical examples, including using arrays to store scores, linked lists to manage shopping lists, stacks to implement recursion, queues to synchronize threads, and trees and hash tables for fast search and authentication. Understanding these concepts allows you to write efficient and maintainable Java code.
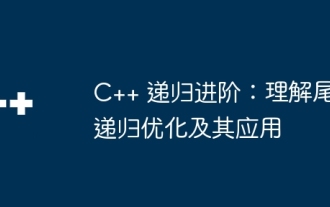
Tail recursion optimization (TRO) improves the efficiency of certain recursive calls. It converts tail-recursive calls into jump instructions and saves the context state in registers instead of on the stack, thereby eliminating extra calls and return operations to the stack and improving algorithm efficiency. Using TRO, we can optimize tail recursive functions (such as factorial calculations). By replacing the tail recursive call with a goto statement, the compiler will convert the goto jump into TRO and optimize the execution of the recursive algorithm.
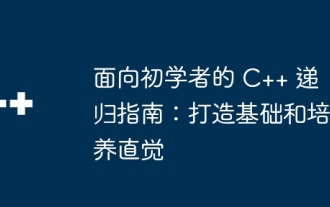
Recursion is a powerful technique that allows a function to call itself to solve a problem. In C++, a recursive function consists of two key elements: the base case (which determines when the recursion stops) and the recursive call (which breaks the problem into smaller sub-problems ). By understanding the basics and practicing practical examples such as factorial calculations, Fibonacci sequences, and binary tree traversals, you can build your recursive intuition and use it in your code with confidence.
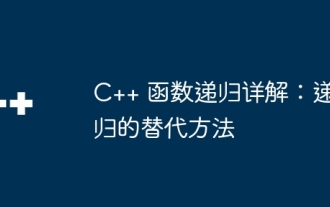
Recursion is a technique where a function calls itself, but has the disadvantages of stack overflow and inefficiency. Alternatives include: tail-recursion optimization, where the compiler optimizes recursive calls into loops; iteration, which uses loops instead of recursion; and coroutines, which allow execution to be paused and resumed, simulating recursive behavior.
