


Java program to extract a string surrounded by single quotes from a larger string using regular expressions
Regular expressions or regular expressions are a language used for pattern matching and string manipulation. It consists of a sequence of characters that defines a search pattern and can be used to perform operations such as searching, replacing, and even validating text input. Regular expressions consist of a sequence of characters and symbols that form a search pattern.
In this article, we will learn how to write a Java program to extract a single-quoted string from a larger string using regular expressions.
Java provides support for regular expressions through the java.util.regex package. Pattern classes represent compiled regular expressions, while matcher classes can be used to match patterns against a given input string.
A single substring enclosed in single quotes
In the following example, we will first define the input string and the regular expression pattern we want to match. The pattern "(_ ?)" matches any sequence of characters enclosed in single quotes and _*? parts. Match any character 0 or more times, but as few times as possible so that the rest of the pattern matches.
We then create a Matcher object based on the pattern to apply to the input string with the help of the find method. If the pattern matches, we extract the matching string using the group() method with parameter 1, which represents the first capturing group in the pattern. The disadvantage of this method is that it does not capture all single-quoted substring groups.
Example
import java.util.regex.Matcher; import java.util.regex.Pattern; public class StringExtractor { public static void main(String[] args) { String input = "This is a 'single quote' enclosed string"; Pattern pattern = Pattern.compile("'(.*?)'"); Matcher matcher = pattern.matcher(input); if (matcher.find()) { String extractedString = matcher.group(1); System.out.println(extractedString); } } }
Output
single quote
Multiple substrings enclosed in single quotes
The above method has a major disadvantage, that is, it is too simple to extract multiple single-quote substrings from the input string, and only extracts the first occurrence. This is an updated and advanced version of the previous method as it is capable of extracting multiple events. We use a while loop to iterate and continue searching for matches until there are no matches left in the input string. A list of matches is used to store all extracted strings and is returned by this method. The main method demonstrates how to use the updated extractStringsWithRegex() method to extract all single-quoted strings.
Example
import java.util.regex.Matcher; import java.util.regex.Pattern; import java.util.ArrayList; import java.util.List; public class StringExtractor { public static List<String> extractStringsWithRegex(String input) { // This function takes string as input, iterates over to search for regex matches // and stores them in a List named matches which is finally returned in the end Pattern pattern = Pattern.compile("'(.*?)'"); Matcher matcher = pattern.matcher(input); List<String> matches = new ArrayList<>(); while (matcher.find()) { matches.add(matcher.group(1)); } return matches; } public static void main(String[] args) { String input = "This is a 'test' string with 'multiple' 'single quote' enclosed 'words'"; List<String> matches = extractStringsWithRegex(input); for (String match : matches) { System.out.println(match); } } }
Output
test multiple single quote words
There are some advantages and disadvantages to the java program for extracting a single quoted string from a larger string using regular expressions as shown below.
advantage
Regular expressions are very powerful and can match strings enclosed in single quotes or even more complex patterns.
The Matcher class provides us with additional methods for processing matching strings, such as finding the starting and ending index of a match.
shortcoming
Regular expressions can be more difficult to write and understand than other methods.
Regular expressions can be slower than other methods, especially for large input strings or complex patterns.
in conclusion
There are several methods for extracting a string enclosed in single quotes, but the most common methods are using regular expressions, split() and substring() methods. Regular expressions are powerful and flexible options because they can handle complex patterns, but can be very time-consuming on very large strings. When using regular expressions, the Pattern class is used to represent the pattern, and the Matcher class is used to apply the pattern to the input string and then extract the matching text. Regular expressions have a variety of use cases, from validating user input data to manipulating text. Whenever working with regular expressions, it is important to carefully design and test the pattern to ensure that it matches the required text and handles all possible edge cases well.
The above is the detailed content of Java program to extract a string surrounded by single quotes from a larger string using regular expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
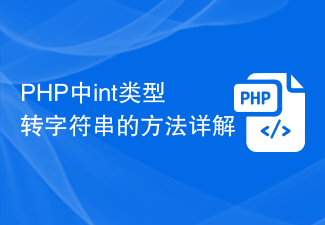
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
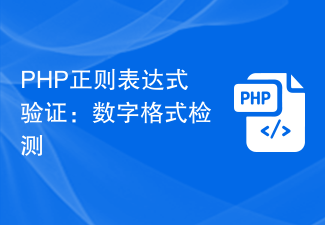
PHP regular expression verification: Number format detection When writing PHP programs, it is often necessary to verify the data entered by the user. One of the common verifications is to check whether the data conforms to the specified number format. In PHP, you can use regular expressions to achieve this kind of validation. This article will introduce how to use PHP regular expressions to verify number formats and provide specific code examples. First, let’s look at common number format validation requirements: Integers: only contain numbers 0-9, can start with a plus or minus sign, and do not contain decimal points. floating point
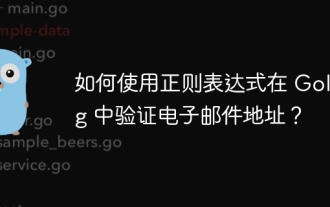
To validate email addresses in Golang using regular expressions, follow these steps: Use regexp.MustCompile to create a regular expression pattern that matches valid email address formats. Use the MatchString function to check whether a string matches a pattern. This pattern covers most valid email address formats, including: Local usernames can contain letters, numbers, and special characters: !.#$%&'*+/=?^_{|}~-`Domain names must contain at least One letter, followed by letters, numbers, or hyphens. The top-level domain (TLD) cannot be longer than 63 characters.
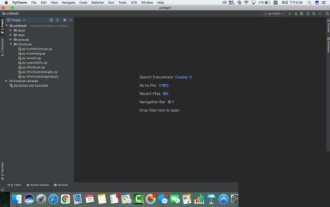
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
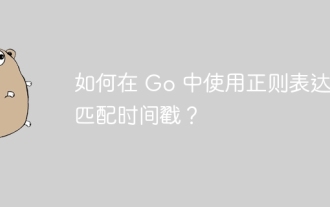
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
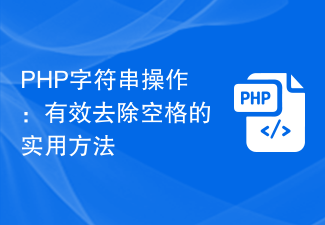
PHP String Operation: A Practical Method to Effectively Remove Spaces In PHP development, you often encounter situations where you need to remove spaces from a string. Removing spaces can make the string cleaner and facilitate subsequent data processing and display. This article will introduce several effective and practical methods for removing spaces, and attach specific code examples. Method 1: Use the PHP built-in function trim(). The PHP built-in function trim() can remove spaces at both ends of the string (including spaces, tabs, newlines, etc.). It is very convenient and easy to use.
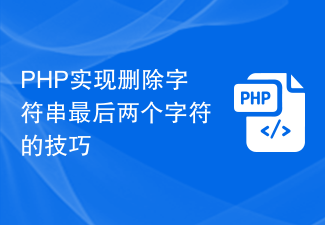
As a scripting language widely used to develop web applications, PHP has very powerful string processing functions. In daily development, we often encounter operations that require deleting a string, especially the last two characters of the string. This article will introduce two PHP techniques for deleting the last two characters of a string and provide specific code examples. Tip 1: Use the substr function The substr function in PHP is used to return a part of a string. We can easily remove characters by specifying the string and starting position
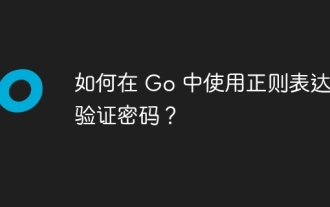
The method of using regular expressions to verify passwords in Go is as follows: Define a regular expression pattern that meets the minimum password requirements: at least 8 characters, including lowercase letters, uppercase letters, numbers, and special characters. Compile regular expression patterns using the MustCompile function from the regexp package. Use the MatchString method to test whether the input string matches a regular expression pattern.
