How to convert JavaScript iterator to array?
In JavaScript, an iterator is a collection of elements through which we can iterate and access a single element in each iteration. Collections, maps or objects are iterators in JavaScript and we cannot access the elements of iterators using indexes like we can with arrays.
So, we first need to convert the iterator to an array. Here we will convert iterator to array using different methods like array.from() etc.
Use for-of loop
for-of loops over each element of the iterator lies collection and map. In the for-of loop we can access and add elements to the array and we can use push() method to add elements to the array.
grammar
Users can use the for-of loop to convert the iterator into an array according to the following syntax.
for (let element of iterator) { array.push(element); }
In the above syntax, we access the elements of the iterator in the for-of loop and push it to the array
Example 1
In the example below, we create test_array and initialize it with some numbers. After that, we use Symbol.iterator() to convert the array into an iterator.
Next, we use a for-of loop to iterate over the iterator. We access all the elements of the iterator one by one and push them into the array. Once all iterations of the for loop are completed, we get the complete array of iterators.
<html> <body> <h2>Using the <i> for loop </i> to transform JavaScript iterator into the array</h2> <div id = "output"> </div> </body> <script> let output = document.getElementById('output'); let test_array = [10, 20, 30, 0, 50, 60, 70]; let iterator = test_array[Symbol.iterator](); let array = []; for (let element of iterator) { array.push(element); output.innerHTML += "Array element is - " + element + "<br>"; } output.innerHTML += "The whole array is - " + array; </script> </html>
Use array.from() method
The Array.from() method creates an array from an iterator. We need to pass the iterator object as parameter of array.from() method. After converting the iterator to an array, it returns an array.
grammar
Users can use the array.from() method according to the following syntax to convert the iterator to an array.
let array = Array.from(test_set);
In the above syntax, test_set is an iterator to be converted to an array.
parameter
test_set – It is an iterator to be converted to an array.
Example 2
In the example below, we create a collection using various elements. After that, we convert the collection into an array using the array.from() method. In the output, the user can see the array returned from the array.from() method.
<html> <body> <h2>Using the <i> Array.from() method </i> to transform JavaScript iterator into the array.</h2> <div id = "output"> </div> </body> <script> let output = document.getElementById('output'); let test_set = new Set(["Hello", "Hello", "Hi", 10, 10, 20, 30, 40, 40, true, false, true, true]); let array = Array.from(test_set); output.innerHTML += "The array from the test_set is " + array; </script> </html>
Use spread operator
The spread operator also allows us to convert iterators to arrays like the array.from() method. It copies all elements of the iterator into a new array. Additionally, we can use it to clone arrays.
grammar
Users can use the spread operator to convert the iterator into an array according to the following syntax
let array = [...test_map];
In the above syntax, test_map is an iterator.
Example 3
In the example below, we create a map with unique keys and values. We can access specific values from the map using keys.
We have used the spread operator to convert test_map to an array. In the output, the user can see that each key and value of the map has been added to the array
<html> <body> <h2>Using the <i> Spread operator </i> to transform JavaScript iterator into the array.</h2> <div id = "output"> </div> </body> <script> let output = document.getElementById('output'); var test_map = new Map([["first", true], ["second", false], ["third", false], ["fourth", true]]); let array = [...test_map]; output.innerHTML += "The array from the test_map is " + array; </script> </html>
Example 4
In this example, we convert the collection iterator to an array. The new Set() constructor is used to create sets from numeric, boolean and string elements.
After that, we use the spread operator to convert the collection iterator into an array.
<html> <body> <h2>Using the <i> Spread operator </i> to transform JavaScript iterator into the array.</h2> <div id="output"> </div> </body> <script> let output = document.getElementById('output'); let set = new Set([30, 40, "TypeScript", "JavaScript"]) let array = [...set] output.innerHTML += "The array from the object is " + array; </script> </html>
In this tutorial, we looked at three different ways to convert an iterator into an array. The best way is to use the spread operator as it also provides another functionality like cloning an array.
The above is the detailed content of How to convert JavaScript iterator to array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
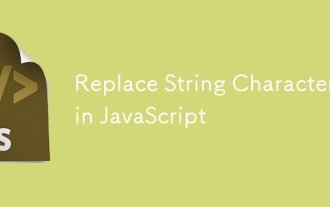
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
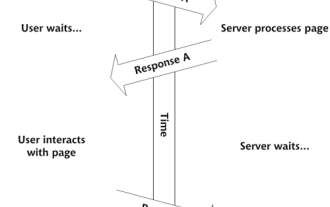
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
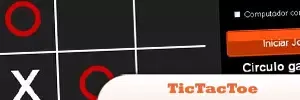
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
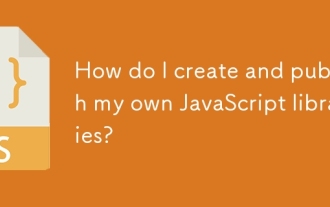
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
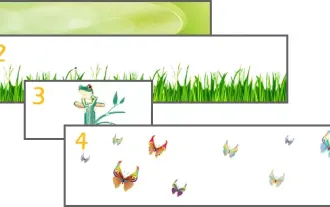
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
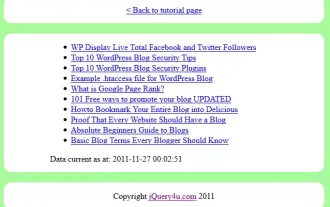
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
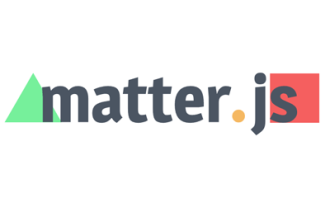
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
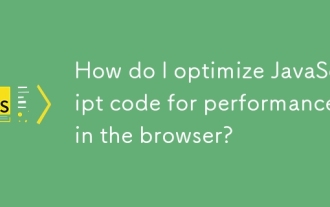
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
