How to convert JSON string to Bean using JSON-lib API in Java?
JSON-lib API is a Java library for serializing and deserializing java beans, maps, arrays and collections in JSON format. We need to convert the JSON string to a bean by first converting the string to a JSON object and then converting it to a java bean.
Syntax
public static Object toBean(JSONObject jsonObject, Class beanClass)
In the following program we can convert JSON string to bean.
Example
import net.sf.json.JSONObject; import net.sf.json.JSONSerializer; public class ConvertJSONStringToBeanTest { public static void main(String[] args) { String jsonStr = "{\"firstName\": \"Adithya\", \"lastName\": \"Sai\", \"age\": 30, \"technology\": \"Java\"}"; JSONObject jsonObj = (JSONObject)JSONSerializer.toJSON(jsonStr); // convert String to JSON System.out.println(jsonObj); Student student = (Student)JSONObject.toBean(jsonObj, Student.class); // convert JSON to Bean System.out.println(student.toString()); } public static class Student { private String firstName; private String lastName; private int age; private String technology; public Student() { } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getTechnology () { return technology; } public void setTechnology(String technology) { this.technology = technology; } public String toString() { return "Student[ " + "firstName = " + firstName + ", lastName = " + lastName + ", age = " + age + ", technology = " + technology + " ]"; } } }
Output
{"firstName":"Adithya","lastName":"Sai","age":30,"technology":"Java"} Student[ firstName = Adithya, lastName = Sai, age = 30, technology = Java ]
The above is the detailed content of How to convert JSON string to Bean using JSON-lib API in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
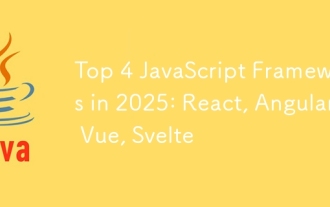
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
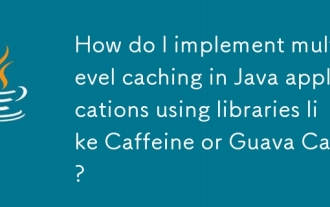
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
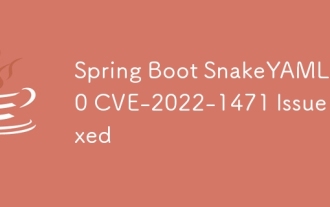
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
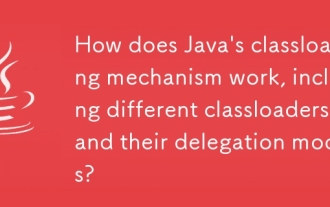
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
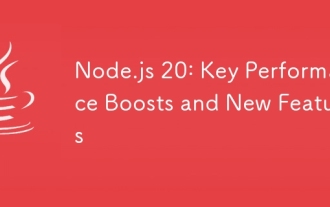
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
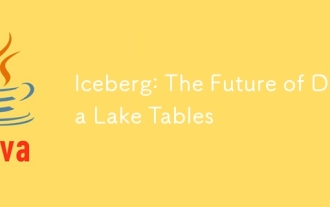
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
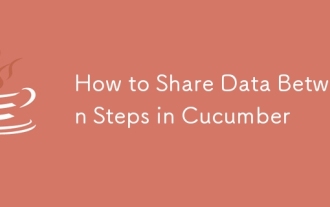
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
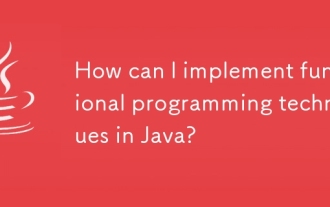
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
