


Practical guidance on Java technology optimization to improve database search performance
Title: Java technology optimization practical guide to improve database search performance
Introduction:
In modern application development, database search is a common requirement. However, as the amount of data increases and user access increases, the performance of database searches may become a bottleneck of the system. In order to improve database search performance, application response speed and user experience, this article will introduce some Java technology optimization practical guidance and provide specific code examples.
1. Use indexes
Database indexes can speed up searches, and you can quickly locate specified data by creating indexes. In Java, we can use technologies such as JDBC or JPA to operate the database. By creating appropriate indexes, the performance of database searches can be significantly improved.
For example, the sample code for using JDBC to create an index is as follows:
// 创建索引 Connection conn = DriverManager.getConnection(url, username, password); Statement stmt = conn.createStatement(); stmt.execute("CREATE INDEX idx_name ON users (name)"); stmt.close(); conn.close(); // 执行查询 conn = DriverManager.getConnection(url, username, password); stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM users WHERE name = 'John'"); while(rs.next()) { System.out.println(rs.getString("name")); } rs.close(); stmt.close(); conn.close();
2. Using a connection pool
Database connections are an expensive operation, so frequently creating and closing connections will Resulting in performance degradation. To avoid this situation, we can use connection pooling technology to manage database connections. Connection pooling can reuse existing connections, reducing the overhead of creating and closing connections.
The following is a sample code for using HikariCP connection pool:
HikariConfig config = new HikariConfig(); config.setJdbcUrl(url); config.setUsername(username); config.setPassword(password); HikariDataSource dataSource = new HikariDataSource(config); try (Connection conn = dataSource.getConnection(); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM users WHERE name = 'John'")) { while (rs.next()) { System.out.println(rs.getString("name")); } } catch (SQLException e) { e.printStackTrace(); } dataSource.close();
3. Using paging query
When the amount of data in the database is large, returning all results at once may cause memory problems Overflow or network transmission performance degradation. To mitigate this, we can use paginated queries to reduce the number of results returned. By setting appropriate paging parameters, query operations can be made more efficient.
The following is a sample code for paging query using MyBatis paging plug-in:
@Mapper public interface UserMapper { List<User> getUsers(@Param("name") String name, @Param("startIndex") int startIndex, @Param("pageSize") int pageSize); } // 查询数据,并设置分页参数 int pageSize = 10; int pageNum = 1; int startIndex = (pageNum - 1) * pageSize; List<User> users = userMapper.getUsers("John", startIndex, pageSize); for (User user : users) { System.out.println(user.getName()); }
Conclusion:
By using Java technology optimization practical guidance such as indexing, connection pooling and paging query, it can be effective Improve database search performance. However, specific optimization strategies need to be adjusted and evaluated based on actual conditions. In actual application development, we need to comprehensively consider the system's hardware configuration, database optimization, network transmission and other factors to find the most suitable optimization method. Through continuous optimization and adjustment, the high performance and stability of the system can be guaranteed.
The above is the detailed content of Practical guidance on Java technology optimization to improve database search performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


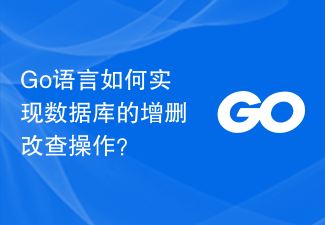
Go language is an efficient, concise and easy-to-learn programming language. It is favored by developers because of its advantages in concurrent programming and network programming. In actual development, database operations are an indispensable part. This article will introduce how to use Go language to implement database addition, deletion, modification and query operations. In Go language, we usually use third-party libraries to operate databases, such as commonly used sql packages, gorm, etc. Here we take the sql package as an example to introduce how to implement the addition, deletion, modification and query operations of the database. Assume we are using a MySQL database.
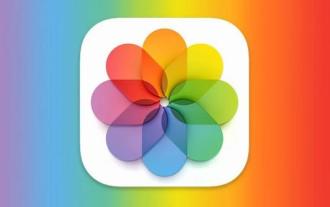
Apple's latest releases of iOS18, iPadOS18 and macOS Sequoia systems have added an important feature to the Photos application, designed to help users easily recover photos and videos lost or damaged due to various reasons. The new feature introduces an album called "Recovered" in the Tools section of the Photos app that will automatically appear when a user has pictures or videos on their device that are not part of their photo library. The emergence of the "Recovered" album provides a solution for photos and videos lost due to database corruption, the camera application not saving to the photo library correctly, or a third-party application managing the photo library. Users only need a few simple steps
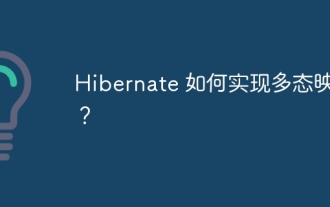
Hibernate polymorphic mapping can map inherited classes to the database and provides the following mapping types: joined-subclass: Create a separate table for the subclass, including all columns of the parent class. table-per-class: Create a separate table for subclasses, containing only subclass-specific columns. union-subclass: similar to joined-subclass, but the parent class table unions all subclass columns.
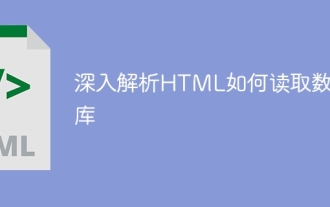
HTML cannot read the database directly, but it can be achieved through JavaScript and AJAX. The steps include establishing a database connection, sending a query, processing the response, and updating the page. This article provides a practical example of using JavaScript, AJAX and PHP to read data from a MySQL database, showing how to dynamically display query results in an HTML page. This example uses XMLHttpRequest to establish a database connection, send a query and process the response, thereby filling data into page elements and realizing the function of HTML reading the database.
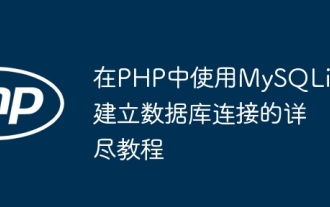
How to use MySQLi to establish a database connection in PHP: Include MySQLi extension (require_once) Create connection function (functionconnect_to_db) Call connection function ($conn=connect_to_db()) Execute query ($result=$conn->query()) Close connection ( $conn->close())
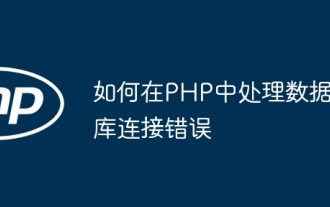
To handle database connection errors in PHP, you can use the following steps: Use mysqli_connect_errno() to obtain the error code. Use mysqli_connect_error() to get the error message. By capturing and logging these error messages, database connection issues can be easily identified and resolved, ensuring the smooth running of your application.
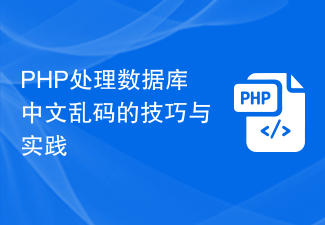
PHP is a back-end programming language widely used in website development. It has powerful database operation functions and is often used to interact with databases such as MySQL. However, due to the complexity of Chinese character encoding, problems often arise when dealing with Chinese garbled characters in the database. This article will introduce the skills and practices of PHP in handling Chinese garbled characters in databases, including common causes of garbled characters, solutions and specific code examples. Common reasons for garbled characters are incorrect database character set settings: the correct character set needs to be selected when creating the database, such as utf8 or u
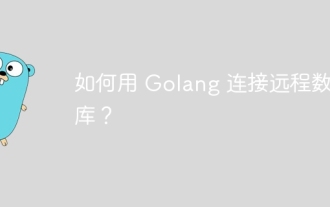
Through the Go standard library database/sql package, you can connect to remote databases such as MySQL, PostgreSQL or SQLite: create a connection string containing database connection information. Use the sql.Open() function to open a database connection. Perform database operations such as SQL queries and insert operations. Use defer to close the database connection to release resources.
