High-performance search algorithms in PHP databases
High-performance search algorithm in PHP database
With the rapid development of the Internet and the increasing amount of data, how to be fast and efficient for a website or application? Searching data locally has become an important issue. To address this problem, this article will introduce a high-performance search algorithm based on PHP database and provide specific code examples.
1. Problem Analysis
In traditional database queries, we usually use fuzzy queries or full-text indexes based on SQL statements to search. However, these methods tend to be less efficient when dealing with large data volumes. Therefore, we need a faster and more efficient search algorithm.
2. High-performance search algorithm
In order to solve the problem of high-performance search, we can use the indexing mechanism of the database and combine it with the data processing capabilities of PHP to design an efficient search algorithm. The specific steps are as follows:
- Data preprocessing
Before the data is stored in the database, we can preprocess the data. For example, for string type data, meaningless characters or symbols can be removed; for numeric type data, data can be normalized. This can reduce storage space and facilitate subsequent searching and sorting. - Database Index
In the database, we can create indexes for the fields that need to be searched. For string type fields, you can use B-tree indexes or full-text indexes; for numeric type fields, you can use B-tree indexes or hash indexes. The creation of indexes can greatly increase the speed of searches. - Search algorithm design
In order to achieve high-performance search, an index-based search algorithm can be designed. The specific steps are as follows:
(1) Receive the search keywords input by the user and process them. Meaningless characters or symbols can be removed and converted to lowercase letters.
(2) Use the database index to match based on the processed search keywords. You can choose to search in a single field or multiple fields according to the actual situation.
(3) Sort according to the matching results. You can design a custom sorting algorithm based on your needs, such as sorting by relevance or sorting by time.
(4) Return search results. You can control the number of results returned, or return results in pages.
- Code Example
The following is a simple example that demonstrates how to use PHP to implement a high-performance search algorithm. Suppose we have a database table user containing user information, which contains the fields name and age. We need to search based on the keywords entered by the user and sort them by relevance.
<?php // 连接数据库 $db = new mysqli('localhost', 'username', 'password', 'database'); // 接收用户输入的搜索关键字 $keyword = $_GET['keyword']; // 去掉无意义的字符或符号,并转换为小写字母 $keyword = strtolower(preg_replace('/[^a-z0-9]+/i', '', $keyword)); // 执行搜索操作 $sql = "SELECT * FROM user WHERE LOWER(name) LIKE '%$keyword%' ORDER BY relevancy DESC"; $result = $db->query($sql); // 输出搜索结果 while ($row = $result->fetch_assoc()) { echo "Name: " . $row['name'] . ", Age: " . $row['age'] . "<br>"; } // 关闭数据库连接 $db->close(); ?>
The above code examples are for demonstration only and need to be adjusted and optimized according to specific circumstances in actual applications.
3. Summary
This article introduces a high-performance search algorithm based on PHP database and provides specific code examples. Through data preprocessing, database indexing and efficient search algorithm design, fast and efficient data search can be achieved when processing large amounts of data. Of course, the algorithm can be further optimized and adjusted for different application scenarios and needs. I hope this article can provide some reference and help for everyone in actual development.
The above is the detailed content of High-performance search algorithms in PHP databases. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


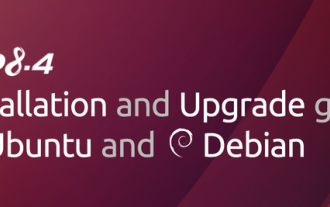
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
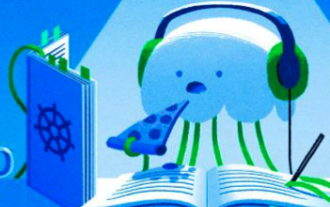
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
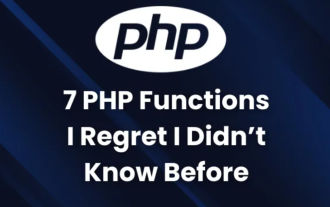
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
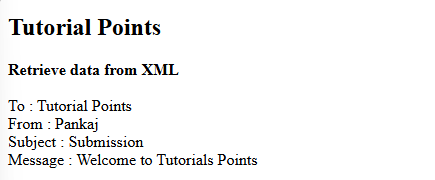
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
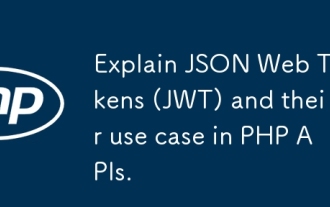
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
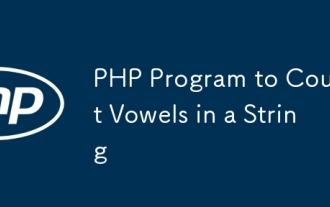
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
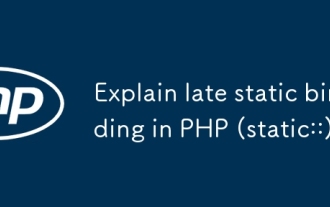
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
