


How does the microservice architecture optimize the code reuse and maintainability of PHP functions?
How does the microservice architecture optimize the code reuse and maintainability of PHP functions?
With the rapid development of the Internet, a single application architecture can no longer meet the development and maintenance of complex requirements. Microservice architecture has received widespread attention due to its architectural flexibility and scalability. In a microservices architecture, applications are split into a series of smaller, independent services, each of which can be deployed and upgraded independently, interacting through a variety of communication protocols and technologies. This article will introduce how to optimize code reuse and maintainability of PHP functions and provide specific code examples.
- Use Composer to manage dependencies
Use Composer to easily manage the dependencies of PHP projects. Dependencies can be easily installed, updated, and upgraded by defining them in your project's composer.json file. This can better realize the reuse and maintenance of functions while reducing code redundancy. Here is an example of a sample composer.json file:
{ "require": { "monolog/monolog": "^2.0" } }
- Using namespaces and class autoloading
Using namespaces in PHP avoids class name conflicts and works better Organize and organize your code. By using the autoloading mechanism, the corresponding file can be automatically loaded based on the namespace and class name of the class. This reduces the effort of manually referencing files and makes the code cleaner and easier to maintain. The following is an example of using namespaces and class autoloading:
namespace AppServices; class UserService { public function getUser($id) { // 查询用户逻辑 } }
- Providing a RESTful API interface
In a microservice architecture, services usually communicate with each other through a RESTful API interface. By providing standardized interfaces, different services can collaborate better and service functions can be reused. The following is an example RESTful API interface:
namespace AppControllers; class UserController { public function getUser($id) { // 调用用户服务的接口获取用户信息 } }
- Using message queue
Using message queue can help decouple the dependencies between services and improve the scalability of the system. By encapsulating tasks into messages, tasks can be processed asynchronously and distributed to different services for processing. This reduces pressure between services and increases system reliability and robustness. The following is an example of using a message queue:
namespace AppServices; class EmailService { public function sendEmail($to, $subject, $content) { // 将邮件任务封装为消息,发送到消息队列 } }
- Using cache
Using cache can improve the performance of the service and reduce the number of calls to the underlying service. By caching frequently used data, you can speed up data access and reading. At the same time, caching can also reduce the pressure on underlying services and improve the scalability of the system. The following is an example of using cache:
namespace AppServices; use PsrSimpleCacheCacheInterface; class UserService { private $cache; public function __construct(CacheInterface $cache) { $this->cache = $cache; } public function getUser($id) { $user = $this->cache->get('user_' . $id); if (!$user) { // 从底层服务获取用户信息 $this->cache->set('user_' . $id, $user); } return $user; } }
Through the above optimization measures, the reusability and maintainability of PHP function code can be improved. Using Composer to manage dependencies, using namespaces and class autoloading, providing RESTful API interfaces, using message queues, and using caching can make PHP code more flexible, reliable, and efficient. The above are some simple examples. The specific implementation and usage will vary according to specific business needs and scenarios. Hope the above content can be helpful to you.
The above is the detailed content of How does the microservice architecture optimize the code reuse and maintainability of PHP functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


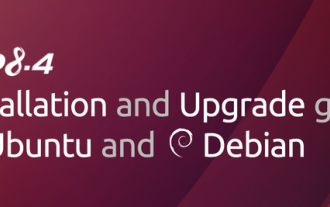
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
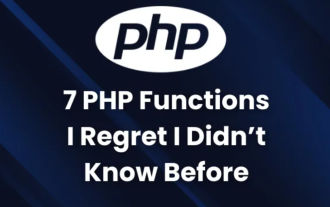
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
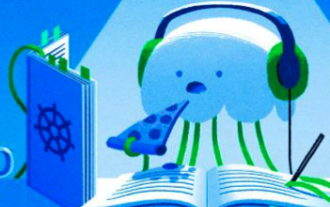
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
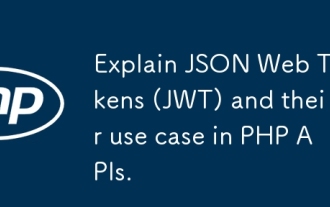
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
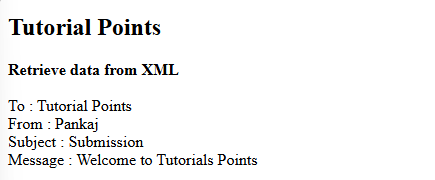
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
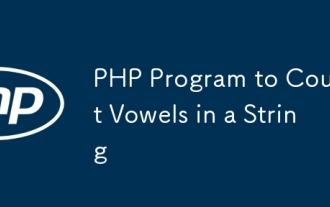
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
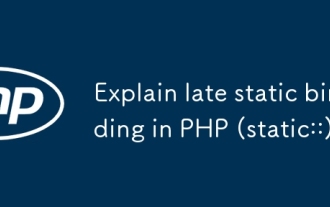
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
