


Understand the application scenarios and implementation steps of Horner's law algorithm in PHP.
Understand the application scenarios and implementation steps of Horner's Rule algorithm in PHP
Introduction:
Horner's Rule algorithm (Horner's Rule) is a method for fast Algorithms for evaluating polynomials. It reduces the computational complexity by converting polynomials into cumulative multiplication and accumulation forms. In PHP programming, Horner's law algorithm is commonly used in polynomial calculations, function evaluation and other fields. This article will introduce the application scenarios of Horner's law algorithm and give specific implementation steps and code examples.
1. Application scenarios of Horner’s law algorithm
Horner’s law algorithm is mainly used for polynomial calculations and function evaluation. It is particularly useful in the following scenarios:
- Polynomial calculation: For a given polynomial, the Horner's rule algorithm can be used to quickly calculate the value of the polynomial at a certain point without having to calculate each term.
- Function evaluation: Some functions can be approximated by polynomials, such as Taylor expansions. Horner's rule algorithm can be used to quickly solve the value of a function at a certain point.
2. Implementation steps of Horner's law algorithm
The following takes the calculation of the value of a polynomial at a certain point as an example to introduce the implementation steps of Horner's law algorithm:
- Determine the coefficients of the polynomial
First, you need to determine the coefficients of the polynomial and put them into an array. The coefficients are arranged from high-order terms to low-order terms. For example, for the polynomial P(x) = 2x^4 3x^3 5x^2 1, the coefficient array is [2, 3, 5, 0, 1]. - Calculate Horner's Law
Use Horner's Law algorithm to perform iterative calculations, starting from higher-order terms and continuing to constant terms. The steps are as follows:
a. Initialize the result variable result to the first element of the coefficient array, that is, result = 2.
b. Starting from the second element of the coefficient array, calculate the result = result * x coefficients in sequence. where x represents the independent variable in the polynomial.
c. Iterative calculation until all coefficients are processed. Finally, the value of the polynomial at the specified point is obtained. - Return the calculated result
Return the calculated result as the value of the polynomial at the specified point.
3. PHP code example
The following is a code example of using PHP to implement Horner's law algorithm:
function hornerAlgorithm($coefficients, $x) { $result = $coefficients[0]; // 初始化结果变量为首个系数 for ($i = 1; $i < count($coefficients); $i++) { $result = $result * $x + $coefficients[$i]; // 迭代计算 } return $result; // 返回计算结果 } // 示例:计算多项式 P(x) = 2x^4 + 3x^3 + 5x^2 + 1,在 x = 2 的值 $coefficients = [2, 3, 5, 0, 1]; $x = 2; $result = hornerAlgorithm($coefficients, $x); echo "多项式在 x = 2 的值为:" . $result;
The above code implements Horner's law algorithm and calculates Polynomial P(x) = 2x^4 3x^3 5x^2 1 at x = 2. The output is that the value of the polynomial at x = 2 is: 55.
Conclusion:
Horner's law algorithm is an effective method for quickly calculating polynomials, which can reduce calculation complexity while increasing calculation speed. In PHP programming, Horner's law algorithm is widely used in scenarios such as polynomial calculations and function evaluation. Through the above steps and code examples, you can understand and master the implementation of Horner's law algorithm, and use it flexibly in practical applications.
The above is the detailed content of Understand the application scenarios and implementation steps of Horner's law algorithm in PHP.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


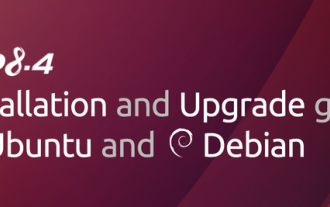
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
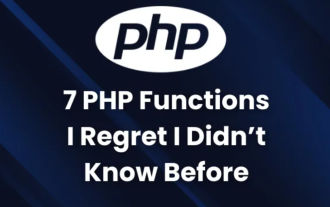
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
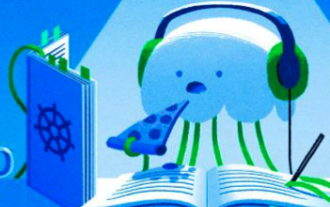
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
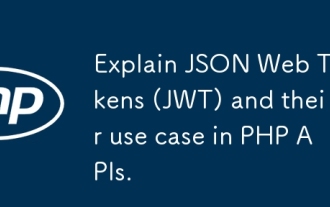
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
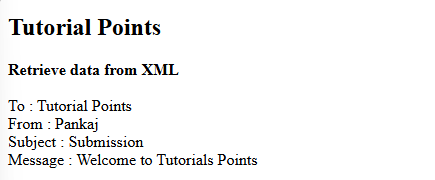
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
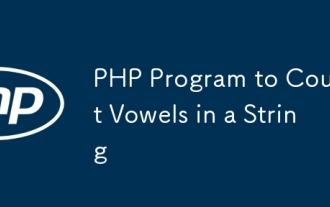
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
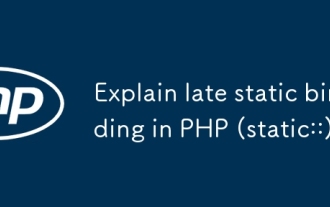
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
