How to write a radix sort algorithm using C#
How to use C# to write a radix sorting algorithm
Introduction:
Radix Sort (Radix Sort) is a non-comparative sorting algorithm suitable for integers Sort. Its basic idea is to sort the elements to be sorted from low to high to obtain an ordered sequence. Compared with other sorting algorithms, radix sorting has lower time complexity and stability.
Implementation steps:
- Find the largest number in the array to be sorted and determine its number of digits.
- According to the maximum number of digits, proceed to the next step from low to high.
- Perform counting sorting on the array to be sorted, and group according to the current number.
- Recombine the grouped array into a new array to be sorted.
- Repeat steps 3 and 4 until all digits have been compared.
Code sample:
The following is a sample code for the radix sorting algorithm written in C#:
using System; public class RadixSort { public static void Sort(int[] array) { int max = GetMaxValue(array); int digits = GetDigits(max); for (int i = 0; i < digits; i++) { CountingSort(array, i); } } private static int GetMaxValue(int[] array) { int max = array[0]; for (int i = 1; i < array.Length; i++) { if (array[i] > max) { max = array[i]; } } return max; } private static int GetDigits(int number) { int digits = 0; while (number > 0) { number /= 10; digits++; } return digits; } private static void CountingSort(int[] array, int digit) { int[] count = new int[10]; int[] sortedArray = new int[array.Length]; for (int i = 0; i < array.Length; i++) { int digitValue = GetDigitValue(array[i], digit); count[digitValue]++; } for (int i = 1; i < count.Length; i++) { count[i] += count[i - 1]; } for (int i = array.Length - 1; i >= 0; i--) { int digitValue = GetDigitValue(array[i], digit); int index = count[digitValue] - 1; sortedArray[index] = array[i]; count[digitValue]--; } for (int i = 0; i < array.Length; i++) { array[i] = sortedArray[i]; } } private static int GetDigitValue(int number, int digit) { for (int i = 0; i < digit; i++) { number /= 10; } return number % 10; } } public class Program { public static void Main(string[] args) { int[] array = { 170, 45, 75, 90, 802, 24, 2, 66 }; Console.WriteLine("Before sorting:"); foreach (int num in array) { Console.Write(num + " "); } RadixSort.Sort(array); Console.WriteLine(" After sorting:"); foreach (int num in array) { Console.Write(num + " "); } } }
Running results:
Before sorting: 170 45 75 90 802 24 2 66 After sorting: 2 24 45 66 75 90 170 802
Summary:
The radix sort algorithm is a relatively efficient sorting algorithm that can quickly sort integer arrays. By sorting the array to be sorted from low to high, an ordered array is finally obtained. When using C# to write a radix sorting algorithm, we need to first find the maximum value and number of digits of the array to be sorted, then count and sort each digit, and finally recombine the sorted array to obtain an ordered result. As can be seen from the running results of the sample code, the radix sort algorithm can correctly sort the array.
The above is the detailed content of How to write a radix sort algorithm using C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


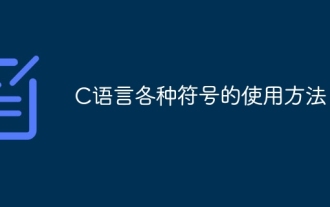
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
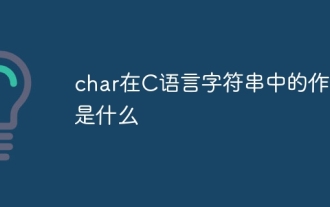
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
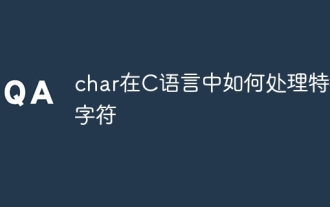
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
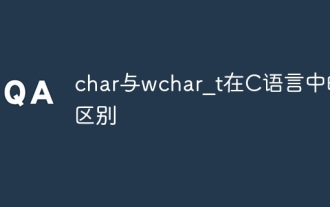
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
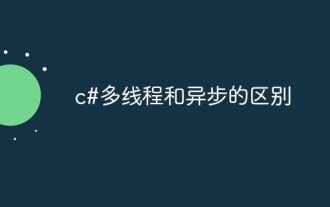
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
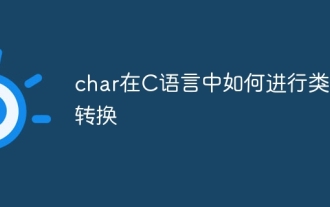
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
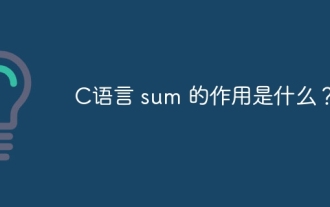
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
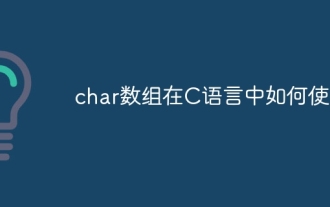
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
