How to use Vue to implement WeChat-like voice message effects
How to use Vue to implement WeChat-like voice message effects
Introduction:
With the development of mobile Internet, voice messages have become one of the important ways for people to communicate in daily life. . WeChat is currently one of the most popular social software, and the voice message special effects experience it provides is deeply loved by users. This article will introduce how to use Vue to implement WeChat-like voice message effects and provide specific code examples.
- Preparation
Before we begin, we need to ensure that Vue and related development environments have been installed. You can use the Vue CLI to create a new project or add Vue dependencies to an existing project. - Create component
We first need to create a voice message component named VoiceMessage.vue. This component will be responsible for displaying the icon, duration and special effects of the voice message.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
|
In the above code, we use Vue’s single-file component format, which includes templates, scripts and styles. The voice message component has an icon and a duration label, and the style of the icon can be dynamically changed according to the playback status.
- Implement playback logic
In methodplayAudio
, we will implement the playback logic of the voice. You can use the HTML5<audio>
element to play audio. We add an audio object to the component's data and perform corresponding operations in theplayAudio
method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
|
In the above code, we first determine whether this.audio
already exists. If it does not exist, create a new Audio
object and Pass the path to the audio file. Then determine whether to play the audio or pause the audio based on the status of playing
.
- Add special effects
In order to achieve WeChat-like voice message special effects, we can use the@keyframes
rules in CSS. Add the following code to the style.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
In the above code, we define an animation named pulse
to change the transform
property of the icon from the initial state scale( 1)
changes to scale(1.2)
, and performs an unlimited number of alternating movements back and forth within 1 second. By adding the animation
attribute to the style of .icon.active
, the animation will start running when the icon's active
class is added.
- Using components
Now we can use the voice message component we just created in other Vue components.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
In the above code, we introduced the voice message component just created through import
and registered the component in components
. The component can then be instantiated in the template using the <voice-message></voice-message>
tag.
Summary:
This article introduces how to use Vue to implement WeChat-like voice message effects. By creating a voice message component, implementing playback logic and adding special effects, we can easily implement a WeChat-like voice message experience in the Vue project. I hope this article is helpful to you, thank you for reading.
The above is the detailed content of How to use Vue to implement WeChat-like voice message effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


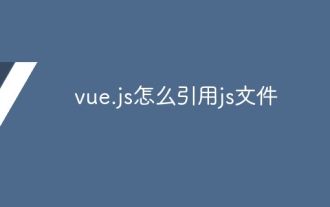
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
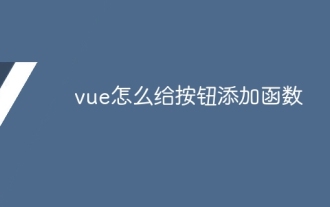
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
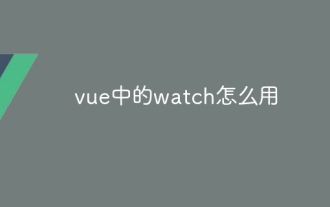
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
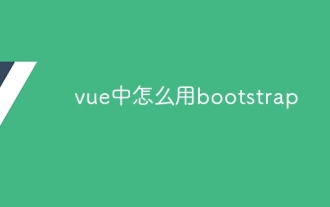
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
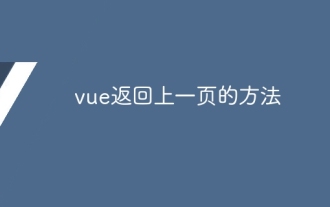
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
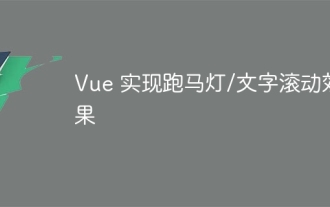
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
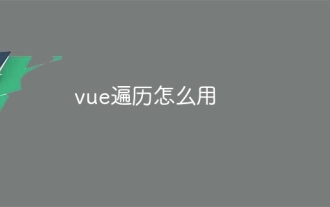
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
