How to use java to implement graph connectivity algorithm
How to use Java to implement the connectivity algorithm of graphs
Introduction:
The graph is one of the common data structures in computer science. It consists of nodes (vertices) and sides. The connectivity of a graph means that all nodes in the graph can be connected to each other through edges. In the fields of algorithms and networks, judging the connectivity of graphs is very important because it can help us solve many problems, such as troubleshooting in networks, relationship analysis in social networks, etc. This article will introduce how to use Java to implement the graph connectivity algorithm and provide specific code examples.
- Representation of graph
In Java, we can use the adjacency matrix or adjacency list of the graph to represent a graph. The adjacency matrix is a two-dimensional array in which the array elements represent the connection relationships between nodes. An adjacency list is an array of linked lists, where each linked list represents the neighbor nodes of each node. - Depth-first search (DFS) algorithm
Depth-first search is an algorithm for traversing a graph. It starts from a starting node and recursively visits its unvisited neighbor nodes until no node is reachable. Through depth-first search, we can traverse the entire graph and determine whether the graph is connected.
The following is the Java code that uses the depth-first search algorithm to determine whether a graph is connected:
import java.util.ArrayList; import java.util.List; public class GraphConnectivity { private int numNodes; private List<List<Integer>> adjList; private boolean[] visited; public GraphConnectivity(int numNodes) { this.numNodes = numNodes; adjList = new ArrayList<>(); for (int i = 0; i < numNodes; i++) { adjList.add(new ArrayList<>()); } visited = new boolean[numNodes]; } public void addEdge(int src, int dest) { adjList.get(src).add(dest); adjList.get(dest).add(src); } private void dfs(int node) { visited[node] = true; for (int neighbor : adjList.get(node)) { if (!visited[neighbor]) { dfs(neighbor); } } } public boolean isGraphConnected() { dfs(0); for (boolean visit : visited) { if (!visit) { return false; } } return true; } public static void main(String[] args) { GraphConnectivity graph = new GraphConnectivity(5); graph.addEdge(0, 1); graph.addEdge(0, 2); graph.addEdge(3, 4); System.out.println("Is the graph connected? " + graph.isGraphConnected()); } }
In the above code, we created a GraphConnectivity
class to represent a graph. Use adjacency lists to store connections between nodes. addEdge
method is used to add edges between nodes. The dfs
method is a recursive method used for depth-first search. The isGraphConnected
method checks the connectivity of the graph by calling dfs(0)
.
Run the above code, the output result is: Is the graph connected? false. This shows that the graph is not connected, because nodes 0, 1, 2 are connected, nodes 3, 4 are connected, but node 0 and node 3 are not connected.
- Breadth-First Search (BFS) Algorithm
Breadth-First Search is also an algorithm for traversing graphs. It starts from a starting node, visits its neighbor nodes, and traverses layer by layer until it finds the target node or traverses the entire graph. Through breadth-first search, we can find the shortest path between two nodes and determine whether the graph is connected.
The following is the Java code that uses the breadth-first search algorithm to determine whether a graph is connected:
import java.util.ArrayList; import java.util.LinkedList; import java.util.List; import java.util.Queue; public class GraphConnectivity { private int numNodes; private List<List<Integer>> adjList; private boolean[] visited; public GraphConnectivity(int numNodes) { this.numNodes = numNodes; adjList = new ArrayList<>(); for (int i = 0; i < numNodes; i++) { adjList.add(new ArrayList<>()); } visited = new boolean[numNodes]; } public void addEdge(int src, int dest) { adjList.get(src).add(dest); adjList.get(dest).add(src); } public boolean isGraphConnected() { Queue<Integer> queue = new LinkedList<>(); int startNode = 0; queue.offer(startNode); visited[startNode] = true; while (!queue.isEmpty()) { int node = queue.poll(); for (int neighbor : adjList.get(node)) { if (!visited[neighbor]) { queue.offer(neighbor); visited[neighbor] = true; } } } for (boolean visit : visited) { if (!visit) { return false; } } return true; } public static void main(String[] args) { GraphConnectivity graph = new GraphConnectivity(5); graph.addEdge(0, 1); graph.addEdge(0, 2); graph.addEdge(3, 4); System.out.println("Is the graph connected? " + graph.isGraphConnected()); } }
In the above code, we call Queue
to implement breadth Prioritize search. We add the starting node to the queue through queue.offer(startNode)
, and then enter the loop until the queue is empty. Compared with depth-first search, breadth-first search traverses the graph layer by layer.
Run the above code, the output result is: Is the graph connected? false. This also shows that the graph is not connected, because nodes 0, 1, and 2 are connected, nodes 3, and 4 are connected, but node 0 and node 3 are not connected.
Conclusion:
This article introduces how to use Java to implement graph connectivity algorithms, including depth-first search and breadth-first search algorithms. These algorithms can help us determine whether a graph is connected and find the shortest path between two nodes. Through these algorithms, we can better understand problems related to computer networks and graph theory and apply them to practical development. Hope this article helps you!
The above is the detailed content of How to use java to implement graph connectivity algorithm. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


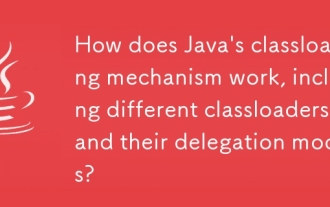
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
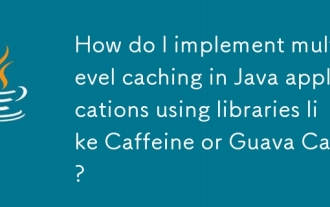
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
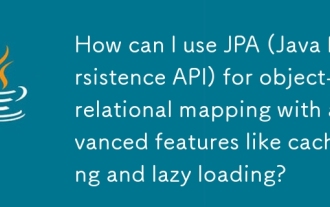
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
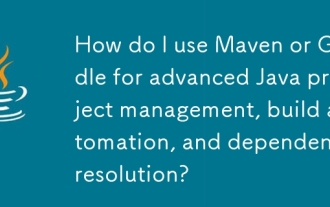
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
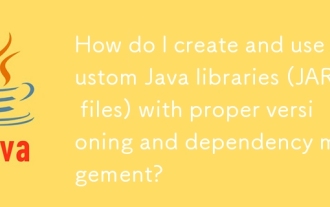
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
