How to use Vue to implement Lenovo search effects
How to use Vue to implement associative search effects
Introduction:
Associative search is a common function in modern websites and applications. It can be based on the user's Enter real-time display of search suggestions related to it. In this article, we will introduce how to use the Vue framework to implement a simple association search function, and provide specific code examples.
Vue framework introduction:
Vue is a popular JavaScript framework for building user interfaces. It's easy to learn and use, and highly scalable. Vue makes building interactive applications easier and more efficient through the ideas of data binding and componentization.
Implementation steps:
-
Create a Vue application:
First, we need to create an instance of Vue to manage the data and interaction of our application . Introduce the Vue library in HTML and create a Vue instance in JavaScript.<html> <head> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <!-- Your HTML code here --> </div> </body> </html>
Copy after login<script> new Vue({ el: '#app', data: { inputValue: '', suggestions: [] }, methods: { // Your methods here } }); </script>
Copy after login Monitor changes in the input box:
We need to monitor what the user inputs in the input box and obtain relevant Lenovo search suggestions in real time when the input changes. This function can be achieved using Vue's v-model directive.<input type="text" v-model="inputValue" @input="getSuggestions" />
Copy after loginmethods: { getSuggestions: function() { // Your code to get suggestions based on inputValue here } }
Copy after loginGet Lenovo search suggestions:
When the user enters content in the input box, we need to send an asynchronous request to obtain related Lenovo search suggestions. In Vue, we can use libraries such as Axios or Vue-resource to send HTTP requests.methods: { getSuggestions: function() { axios.get('/getSuggestions', { params: { keyword: this.inputValue } }) .then(response => { this.suggestions = response.data; }) .catch(error => { console.error(error); }); } }
Copy after loginDisplay search suggestions:
After obtaining the Lenovo search suggestions, we need to display them on the page for users to choose. You can use Vue's v-for directive to iterate through the suggestions array and render each suggestion onto the page.<ul> <li v-for="item in suggestions" :key="item.id"> {{ item.name }} </li> </ul>
Copy after logindata: { suggestions: [] }
Copy after loginNote: This is a simple example. In actual applications, you may need to filter and sort the search results.
Summary:
This article introduces how to use the Vue framework to implement association search effects. By monitoring changes in the input box, sending asynchronous requests to obtain relevant search suggestions, and then displaying the search suggestions on the page, we can implement a real-time association search function. The above is just a simple example, you can expand and optimize it according to actual needs. I hope this article can help you understand and use the Vue framework.
The above is the detailed content of How to use Vue to implement Lenovo search effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


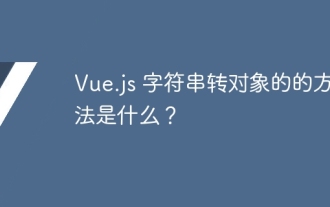
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
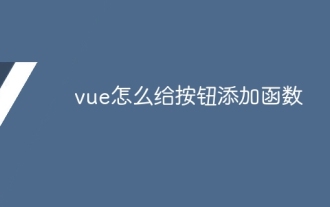
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
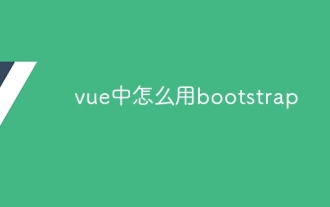
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
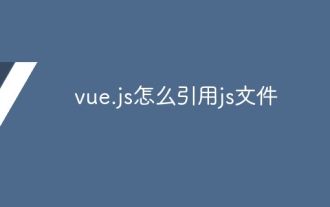
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
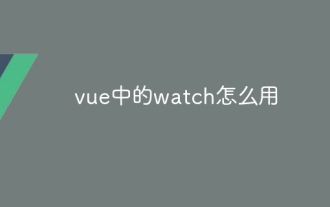
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
