How to implement recursive algorithm using java
How to use Java to implement recursive algorithms
The recursive algorithm is a very powerful and commonly used algorithm. In programming, we often encounter the need to use recursive algorithms to solve problems. Case. Java, as a popular programming language, provides good support for implementing recursive algorithms. This article will introduce you to how to implement recursive algorithms using Java and provide specific code examples.
1. The concept of recursive algorithm
Recursive algorithm refers to an algorithm in which a method calls itself during execution. Generally, recursive algorithms need to satisfy two conditions: the base case and the recursive relationship.
The basic situation refers to the condition under which the recursive algorithm ends execution. If the basic conditions are not met, the recursive algorithm will fall into an infinite loop, causing the program to crash or produce erroneous results.
The recursive relationship means that the recursive algorithm decomposes the problem into smaller sub-problems and solves these sub-problems by calling itself. Recursive algorithms solve problems by making repeated recursive calls until the base case is reached.
2. Steps to implement recursive algorithm using Java
- Define recursive method
First, we need to define a recursive method. Recursive methods must contain base cases, as well as recursive relationships. The base case is the condition at which a recursive algorithm ends, while the recursive relation is the decomposition of the problem into smaller sub-problems and the solution of these sub-problems by calling itself.
The following is an example of a recursive method for calculating factorial:
public int factorial(int n) { // 基本情况 if (n == 0 || n == 1) { return 1; } else { // 递归关系 return n * factorial(n - 1); } }
- Call the recursive method
In practical applications, we need to call the recursive method to Solve the problem. When calling a recursive method, parameters need to be passed in so that the recursive method can execute correctly.
The following is an example of calling the above recursive method for calculating factorial:
public static void main(String[] args) { int n = 5; int result = factorial(n); System.out.println("阶乘结果为:" + result); }
The above code will output "The factorial result is: 120".
3. Precautions for recursive algorithms
In the process of using recursive algorithms, you need to pay attention to the following points:
- Ensure that the recursive method will work under certain conditions End at the bottom to avoid infinite loops.
- Try to avoid using too much recursion, because too many recursive calls may cause stack overflow errors.
- To understand the execution process of the recursive algorithm and the operating mechanism of the recursive relationship.
- Try to use recursive algorithms to solve problems that can be naturally split into sub-problems. Not all problems are suitable for recursive algorithms.
Summary:
The recursive algorithm is a very useful algorithm that can easily solve some problems. This article shows you how to implement recursive algorithms using Java and provides specific code examples. I hope this content is helpful to you and allows you to better understand and apply recursive algorithms.
The above is the detailed content of How to implement recursive algorithm using java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


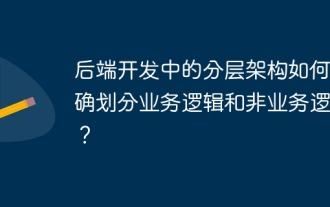
Discussing the hierarchical architecture problem in back-end development. In back-end development, common hierarchical architectures include controller, service and dao...
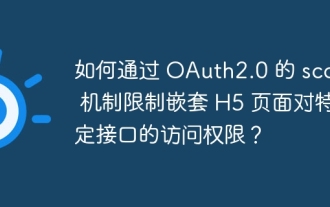
How to use OAuth2.0's access_token to achieve control of interface access permissions? In the application of OAuth2.0, how to ensure that the...
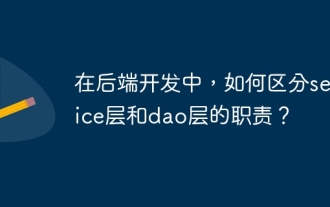
Discussing the hierarchical architecture in back-end development. In back-end development, hierarchical architecture is a common design pattern, usually including controller, service and dao three layers...
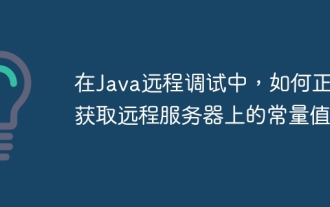
Questions and Answers about constant acquisition in Java Remote Debugging When using Java for remote debugging, many developers may encounter some difficult phenomena. It...
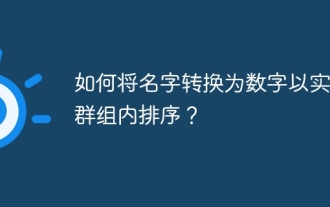
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
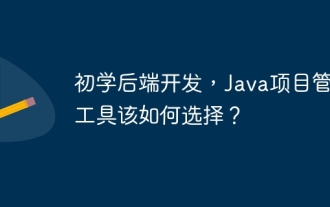
Confused with choosing Java project management tools for beginners. For those who are just beginning to learn backend development, choosing the right project management tools is crucial...
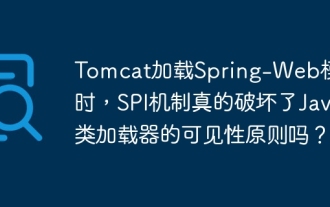
Analysis of class loading behavior of SPI mechanism when Tomcat loads Spring-Web modules. Tomcat is used to discover and use the Servle provided by Spring-Web when loading Spring-Web modules...
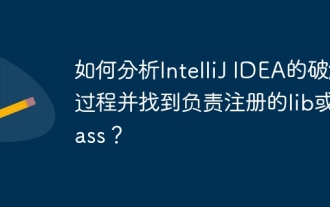
Regarding the analysis method of IntelliJIDEA cracking in the programming world, IntelliJ...
