How to use MongoDB to develop a simple smart home system
How to use MongoDB to develop a simple smart home system
Smart home systems have become a part of modern family life. With the help of smart home systems, we can remotely control various devices in the home, such as lights, appliances, door locks, etc., through mobile phones or other devices. This article will introduce how to use MongoDB to develop a simple smart home system and provide specific code examples for readers' reference.
1. System requirements analysis
Before starting development, we first need to clarify the system requirements. A simple smart home system should have the following functions:
- User login and registration: Users can use the system by registering an account and logging in.
- Device management: Users can add, delete and control various devices, such as lights, appliances, door locks, etc.
- Scheduled tasks: Users can set scheduled tasks, such as switching on and off lights or electrical appliances at scheduled times.
- History record: The system should record the user's control history of the device so that the user can view it.
2. Database design
Based on the above requirements, we can design the following database structure:
-
User table (users):
- _id: User ID
- username: Username
- password: Password
-
Device table ( devices):
- _id: device ID
- name: device name
- type: device type
- status: device status (on/off )
- user_id: User ID
-
Scheduled task list (tasks):
- _id: Task ID
- name: task name
- device_id: device ID
- user_id: user ID
- time: task execution time
-
Operation record table (records):
- _id: Record ID
- device_id: Device ID
- user_id: User ID
- action: operation (on/off)
- time: operation time
3. System development
Next, we will use MongoDB and Node.js to develop smart home systems.
- Environment preparation
First, make sure you have installed Node.js and MongoDB and start the MongoDB service.
- Create project and install dependencies
Execute the following commands on the command line to create a new Node.js project and install the corresponding dependencies:
mkdir smart-home-system cd smart-home-system npm init -y npm install express mongodb
- Create database connection
Create a db.js
file in the root directory and add the following content:
const { MongoClient } = require('mongodb'); async function connect() { try { const client = await MongoClient.connect('mongodb://localhost:27017'); const db = client.db('smart-home-system'); console.log('Connected to the database'); return db; } catch (error) { console.log('Failed to connect to the database'); throw error; } } module.exports = { connect };
- Create routes and controllers
Create a routes
folder in the root directory and add the following routing file devices.js
:
const express = require('express'); const { ObjectId } = require('mongodb'); const { connect } = require('../db'); const router = express.Router(); router.get('/', async (req, res) => { try { const db = await connect(); const devices = await db.collection('devices').find().toArray(); res.json(devices); } catch (error) { res.status(500).json({ error: error.message }); } }); router.post('/', async (req, res) => { try { const { name, type, status, user_id } = req.body; const db = await connect(); const result = await db.collection('devices').insertOne({ name, type, status, user_id: ObjectId(user_id), }); res.json(result.ops[0]); } catch (error) { res.status(500).json({ error: error.message }); } }); module.exports = router;
Create a controllers
folder in the root directory and add the following controller file devicesController.js
:
const { connect } = require('../db'); async function getDevices() { try { const db = await connect(); const devices = await db.collection('devices').find().toArray(); return devices; } catch (error) { throw error; } } async function createDevice(device) { try { const db = await connect(); const result = await db.collection('devices').insertOne(device); return result.ops[0]; } catch (error) { throw error; } } module.exports = { getDevices, createDevice, };
- Create entry file
Create a index.js
file in the root directory and add the following content:
const express = require('express'); const devicesRouter = require('./routes/devices'); const app = express(); app.use(express.json()); app.use('/devices', devicesRouter); app.listen(3000, () => { console.log('Server is running on port 3000'); });
At this point, we have completed the development of a simple smart home system. Including user login and registration, device management, scheduled tasks and operation recording functions.
4. Summary
This article introduces how to use MongoDB to develop a simple smart home system. By using the combination of MongoDB and Node.js, we can easily handle data storage and processing. Readers can further expand this system and add more functions according to specific needs.
The code examples provided in this article are for reference only. Readers should modify and improve them according to actual needs during actual development.
The above is the detailed content of How to use MongoDB to develop a simple smart home system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


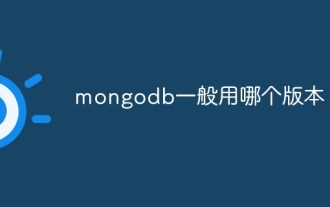
It is recommended to use the latest version of MongoDB (currently 5.0) as it provides the latest features and improvements. When selecting a version, you need to consider functional requirements, compatibility, stability, and community support. For example, the latest version has features such as transactions and aggregation pipeline optimization. Make sure the version is compatible with the application. For production environments, choose the long-term support version. The latest version has more active community support.
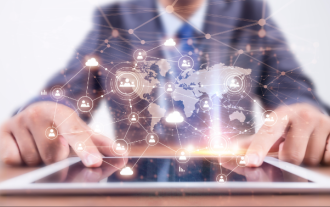
This AI-assisted programming tool has unearthed a large number of useful AI-assisted programming tools in this stage of rapid AI development. AI-assisted programming tools can improve development efficiency, improve code quality, and reduce bug rates. They are important assistants in the modern software development process. Today Dayao will share with you 4 AI-assisted programming tools (and all support C# language). I hope it will be helpful to everyone. https://github.com/YSGStudyHards/DotNetGuide1.GitHubCopilotGitHubCopilot is an AI coding assistant that helps you write code faster and with less effort, so you can focus more on problem solving and collaboration. Git
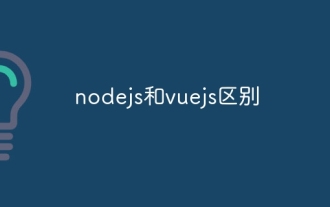
Node.js is a server-side JavaScript runtime, while Vue.js is a client-side JavaScript framework for creating interactive user interfaces. Node.js is used for server-side development, such as back-end service API development and data processing, while Vue.js is used for client-side development, such as single-page applications and responsive user interfaces.
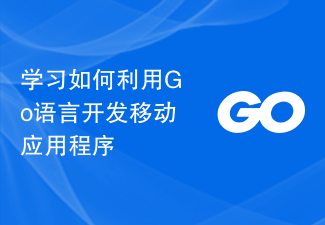
Go language development mobile application tutorial As the mobile application market continues to boom, more and more developers are beginning to explore how to use Go language to develop mobile applications. As a simple and efficient programming language, Go language has also shown strong potential in mobile application development. This article will introduce in detail how to use Go language to develop mobile applications, and attach specific code examples to help readers get started quickly and start developing their own mobile applications. 1. Preparation Before starting, we need to prepare the development environment and tools. head
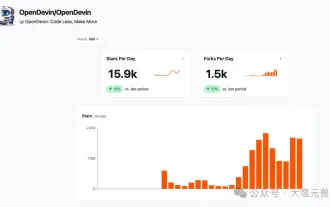
On March 3, 2022, less than a month after the birth of the world's first AI programmer Devin, the NLP team of Princeton University developed an open source AI programmer SWE-agent. It leverages the GPT-4 model to automatically resolve issues in GitHub repositories. SWE-agent's performance on the SWE-bench test set is similar to Devin, taking an average of 93 seconds and solving 12.29% of the problems. By interacting with a dedicated terminal, SWE-agent can open and search file contents, use automatic syntax checking, edit specific lines, and write and execute tests. (Note: The above content is a slight adjustment of the original content, but the key information in the original text is retained and does not exceed the specified word limit.) SWE-A
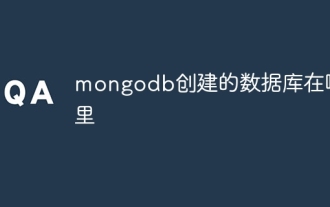
The data of the MongoDB database is stored in the specified data directory, which can be located in the local file system, network file system or cloud storage. The specific location is as follows: Local file system: The default path is Linux/macOS:/data/db, Windows: C:\data\db. Network file system: The path depends on the file system. Cloud Storage: The path is determined by the cloud storage provider.
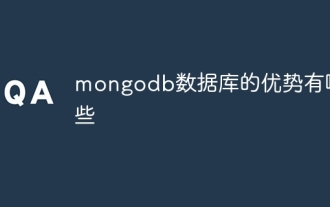
The MongoDB database is known for its flexibility, scalability, and high performance. Its advantages include: a document data model that allows data to be stored in a flexible and unstructured way. Horizontal scalability to multiple servers via sharding. Query flexibility, supporting complex queries and aggregation operations. Data replication and fault tolerance ensure data redundancy and high availability. JSON support for easy integration with front-end applications. High performance for fast response even when processing large amounts of data. Open source, customizable and free to use.
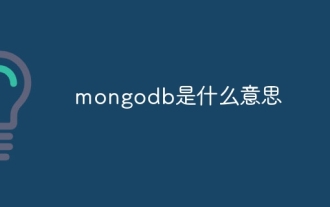
MongoDB is a document-oriented, distributed database system used to store and manage large amounts of structured and unstructured data. Its core concepts include document storage and distribution, and its main features include dynamic schema, indexing, aggregation, map-reduce and replication. It is widely used in content management systems, e-commerce platforms, social media websites, IoT applications, and mobile application development.
