


Learn the design ideas and implementation steps of bitmap sorting algorithm in PHP.
Learn the design ideas and implementation steps of the bitmap sorting algorithm in PHP
Overview
The bitmap sorting algorithm is a sorting algorithm based on bitmaps. By mapping the elements to be sorted to a bitmap, the characteristics of the bitmap are used to achieve efficient sorting. This article will introduce the design idea of the mid-bitmap sorting algorithm and give specific implementation steps and sample code.
Design idea
The design idea of the bitmap sorting algorithm can be summarized into the following steps:
- Create a bitmap: Create a bitmap and initialize all bits as 0.
- Mapping elements: Map the elements to be sorted onto the bitmap, that is, use the elements as subscripts of the bitmap and set the bits at the corresponding positions to 1.
- Bitmap sorting: Traverse the bitmap and output the subscripts with bits 1 in order, which is the sorting result.
Implementation steps
The specific implementation steps and sample codes are given below:
Step 1: Create a bitmap
function createBitmap($maxValue) { $bitmap = []; for ($i = 0; $i <= $maxValue; $i++) { $bitmap[$i] = 0; } return $bitmap; }
This function creates an empty array and initialize all elements to 0 to create a bitmap.
Step 2: Map elements
function mapElement($bitmap, $element) { $bitmap[$element] = 1; return $bitmap; }
This function maps the elements to be sorted onto the bitmap, that is, sets the bit at the corresponding position to 1.
Step 3: Bitmap sorting
function bitmapSort($bitmap) { $result = []; foreach ($bitmap as $key => $value) { if ($value == 1) { $result[] = $key; } } return $result; }
This function traverses the bitmap and outputs the subscripts with bits 1 in order, which is the sorting result.
Sample code
The following is a sample code to demonstrate how to use the median bitmap sorting algorithm:
$unsortedArray = [5, 3, 9, 4, 6, 2, 1, 7, 8]; $maxValue = max($unsortedArray); $bitmap = createBitmap($maxValue); foreach ($unsortedArray as $element) { $bitmap = mapElement($bitmap, $element); } $sortedArray = bitmapSort($bitmap); echo "Sorted Array: "; foreach ($sortedArray as $element) { echo $element . " "; }
In the above sample code, an array to be sorted is first created $ unsortedArray. Then find the maximum value $maxValue in the array and create a bitmap $bitmap. Next, each element in the array is mapped to the bitmap, and finally the bitmapSort function is called to sort the bitmap and output the sorting result.
Summary
The mid-bitmap sorting algorithm is a sorting algorithm based on bitmaps. It maps the elements to be sorted to bitmaps and uses the characteristics of bitmaps to achieve efficient sorting. Through the introduction of this article, we understand the design idea of the median bitmap sorting algorithm, and provide specific implementation steps and sample codes. In actual development, we can choose an appropriate sorting algorithm according to needs, and flexibly use the median bitmap sorting algorithm to improve algorithm efficiency.
The above is the detailed content of Learn the design ideas and implementation steps of bitmap sorting algorithm in PHP.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


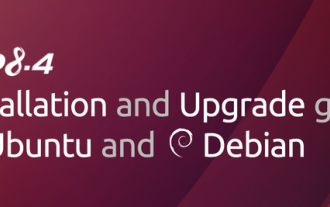
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
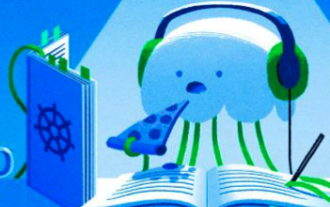
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
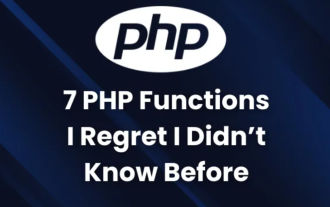
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
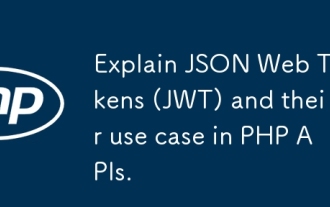
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
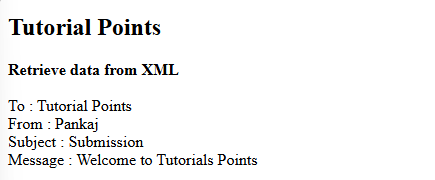
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
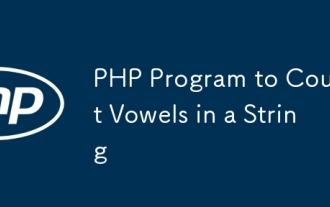
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
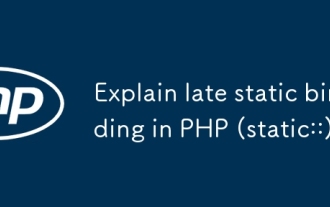
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
