How to use PHP to implement inbox management functions?
How to use PHP to implement inbox management function?
With the rapid development of the Internet, email has become an indispensable and important tool in people's daily life and work. The inbox is an important part of how we receive, manage, and organize emails. In this article, we will introduce how to implement inbox management functions using the PHP programming language and provide some specific code examples.
First, we need to create a database to store email-related data. We can use MySQL or other relational databases to store data. The following is an example of a mailing list:
CREATE TABLE emails ( id INT(11) AUTO_INCREMENT PRIMARY KEY, sender VARCHAR(255), receiver VARCHAR(255), subject VARCHAR(255), content TEXT, sent_at DATETIME );
Next, we can write PHP code to connect to the database and implement inbox management functions. The following is a simple example:
<?php // 连接到数据库 $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } // 获取收件箱中的邮件 $sql = "SELECT * FROM emails WHERE receiver = 'user@example.com'"; $result = $conn->query($sql); if ($result->num_rows > 0) { // 输出每封邮件的信息 while($row = $result->fetch_assoc()) { echo "发件人:" . $row["sender"]. " - 主题:" . $row["subject"]. "<br>"; } } else { echo "收件箱为空"; } $conn->close(); ?>
In the above example, we first create a connection to the database, then query the emails in the inbox and output the results. You can modify the query statement as needed to implement different functions, such as sorting by time, searching for emails with specific topics, etc.
In addition to displaying emails in the inbox, we can also implement other functions, such as deleting emails, marking them as read, etc. The following is a sample code to delete an email:
<?php // 连接到数据库 $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } // 删除指定邮件 $emailId = $_GET["id"]; $sql = "DELETE FROM emails WHERE id = $emailId"; if ($conn->query($sql) === TRUE) { echo "邮件删除成功"; } else { echo "邮件删除失败:" . $conn->error; } $conn->close(); ?>
In the above example, we get the email ID from the URL parameter and use the DELETE statement to delete the corresponding email from the database.
In short, by using the PHP programming language, we can easily implement inbox management functions. We can store and obtain email data by connecting to the database, and implement various functions according to needs. The above examples only provide some basic code examples that you can modify and extend according to your needs. I hope this article will help you use PHP to implement inbox management functions!
The above is the detailed content of How to use PHP to implement inbox management functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


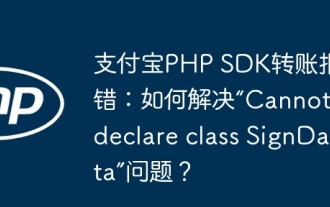
Alipay PHP...
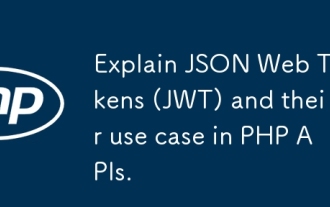
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
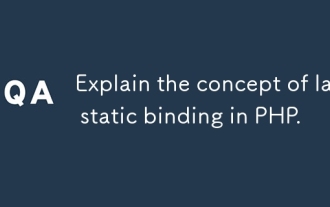
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
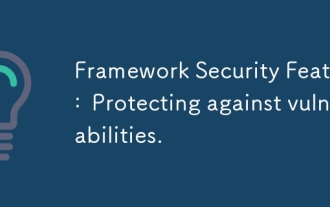
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
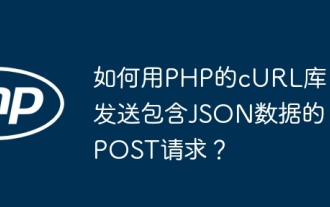
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
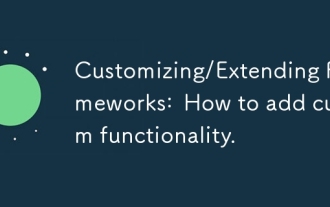
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
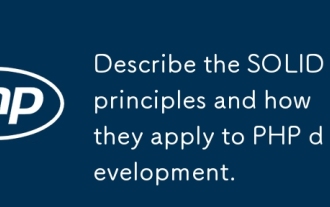
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
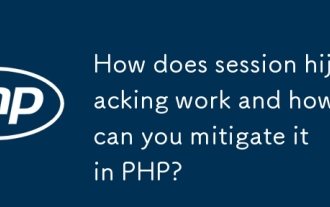
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
