How to develop a simple blockchain system using MongoDB
How to use MongoDB to develop a simple blockchain system
Blockchain technology has attracted much attention in recent years because of its decentralization and high security. It is widely used in fields such as cryptocurrency and contract management. This article will introduce how to use MongoDB to develop a simple blockchain system and provide corresponding code examples.
1. Install and configure MongoDB
First, we need to install MongoDB and configure it accordingly. You can download the latest stable version from MongoDB's official website and install and configure it according to the official documentation.
2. Create databases and collections
In MongoDB, we can store relevant data of the blockchain system by creating databases and collections. Open the MongoDB command line client and enter the following command to create a database and a collection:
use blockchainDB
db.createCollection("blocks")
3. Define the block structure
In the blockchain, each block contains information such as the hash value, transaction data, and timestamp of the previous block. We can use MongoDB's document structure to define the structure of a block. Enter the following command in the command line client:
db.blocks.insertOne({
"previousHash": "0",
"data": "Genisis Block",
" timestamp": new Date()
})
This creates an initial block.
4. Define the blockchain class
Next, we can use Python to define a blockchain class. The following is a simple sample code:
from hashlib import sha256
import json
class Block:
def __init__(self, index, previousHash, data, timestamp): self.index = index self.previousHash = previousHash self.data = data self.timestamp = timestamp self.hash = self.calculateHash() def calculateHash(self): return sha256(str(self.index) + self.previousHash + self.data + str(self.timestamp)).hexdigest()
class Blockchain:
def __init__(self): self.chain = [self.createGenesisBlock()] def createGenesisBlock(self): return Block(0, "0", "Genisis Block", "01/01/2020") def addBlock(self, data): index = len(self.chain) previousHash = self.chain[-1].hash timestamp = datetime.datetime.now().strftime("%d/%m/%Y") newBlock = Block(index, previousHash, data, timestamp) self.chain.append(newBlock) def printChain(self): for block in self.chain: print("Block index:", block.index) print("Previous hash:", block.previousHash) print("Data:", block.data) print("Timestamp:", block.timestamp) print("Hash:", block.hash) print("-" * 20)
Note , the sample code uses Python's hashlib to calculate the hash value of the block, and uses the json module to convert the block information into JSON format.
5. Store blockchain data into MongoDB
In order to store blockchain data into MongoDB, we can use the officially provided Python driver PyMongo. The following is a sample code that transforms the previously defined blockchain class into a form stored in MongoDB:
from pymongo import MongoClient
client = MongoClient()
class Block:
def __init__(self, index, previousHash, data, timestamp): self.index = index self.previousHash = previousHash self.data = data self.timestamp = timestamp self.hash = self.calculateHash() def calculateHash(self): return sha256(str(self.index) + self.previousHash + self.data + str(self.timestamp)).hexdigest() def toDict(self): return { "index": self.index, "previousHash": self.previousHash, "data": self.data, "timestamp": self.timestamp, "hash": self.hash }
class Blockchain:
def __init__(self): self.collection = client.blockchainDB.blocks self.chain = [self.createGenesisBlock()] def createGenesisBlock(self): return Block(0, "0", "Genisis Block", "01/01/2020") def addBlock(self, data): index = len(self.chain) previousHash = self.chain[-1].hash timestamp = datetime.datetime.now().strftime("%d/%m/%Y") newBlock = Block(index, previousHash, data, timestamp) self.collection.insert_one(newBlock.toDict()) self.chain.append(newBlock) def printChain(self): for block in self.collection.find(): print("Block index:", block["index"]) print("Previous hash:", block["previousHash"]) print("Data:", block["data"]) print("Timestamp:", block["timestamp"]) print("Hash:", block["hash"]) print("-" * 20)
In the sample code, we use PyMongo's MongoClient class to connect to MongoDB, which is connected to the local database by default. In the toDict method of the Block class, convert each attribute of the block into dictionary form for storage in MongoDB. In the Blockchain class, we use MongoDB's find method to traverse and print all blocks.
Through the above steps, we developed a simple blockchain system using MongoDB. You can further expand and improve it according to your needs and actual situation. Blockchain technology is not limited to the field of cryptocurrency, but can also be applied to many fields such as contract management and supply chain management to help improve the transparency and security of data.
The above is the detailed content of How to develop a simple blockchain system using MongoDB. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


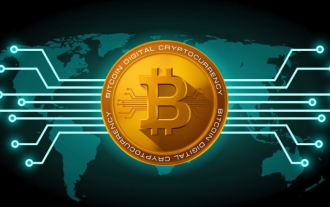
Digital currency rolling positions is an investment strategy that uses lending to amplify trading leverage to increase returns. This article explains the digital currency rolling process in detail, including key steps such as selecting trading platforms that support rolling (such as Binance, OKEx, gate.io, Huobi, Bybit, etc.), opening a leverage account, setting a leverage multiple, borrowing funds for trading, and real-time monitoring of the market and adjusting positions or adding margin to avoid liquidation. However, rolling position trading is extremely risky, and investors need to operate with caution and formulate complete risk management strategies. To learn more about digital currency rolling tips, please continue reading.
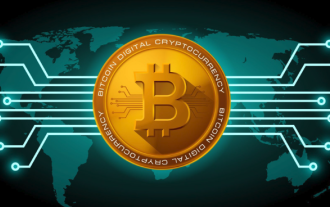
This article recommends ten well-known virtual currency-related APP recommendation websites, including Binance Academy, OKX Learn, CoinGecko, CryptoSlate, CoinDesk, Investopedia, CoinMarketCap, Huobi University, Coinbase Learn and CryptoCompare. These websites not only provide information such as virtual currency market data, price trend analysis, etc., but also provide rich learning resources, including basic blockchain knowledge, trading strategies, and tutorials and reviews of various trading platform APPs, helping users better understand and make use of them
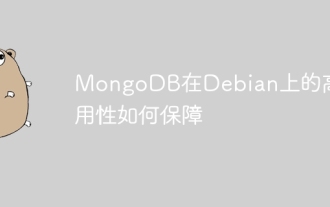
This article describes how to build a highly available MongoDB database on a Debian system. We will explore multiple ways to ensure data security and services continue to operate. Key strategy: ReplicaSet: ReplicaSet: Use replicasets to achieve data redundancy and automatic failover. When a master node fails, the replica set will automatically elect a new master node to ensure the continuous availability of the service. Data backup and recovery: Regularly use the mongodump command to backup the database and formulate effective recovery strategies to deal with the risk of data loss. Monitoring and Alarms: Deploy monitoring tools (such as Prometheus, Grafana) to monitor the running status of MongoDB in real time, and

The handling fees of the Gate.io trading platform vary according to factors such as transaction type, transaction pair, and user VIP level. The default fee rate for spot trading is 0.15% (VIP0 level, Maker and Taker), but the VIP level will be adjusted based on the user's 30-day trading volume and GT position. The higher the level, the lower the fee rate will be. It supports GT platform coin deduction, and you can enjoy a minimum discount of 55% off. The default rate for contract transactions is Maker 0.02%, Taker 0.05% (VIP0 level), which is also affected by VIP level, and different contract types and leverages
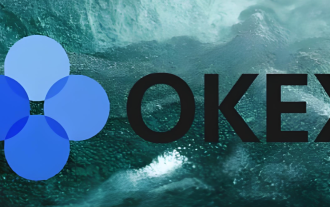
This article introduces in detail the registration, use and cancellation procedures of Ouyi OKEx account. To register, you need to download the APP, enter your mobile phone number or email address to register, and complete real-name authentication. The usage covers the operation steps such as login, recharge and withdrawal, transaction and security settings. To cancel an account, you need to contact Ouyi OKEx customer service, provide necessary information and wait for processing, and finally obtain the account cancellation confirmation. Through this article, users can easily master the complete life cycle management of Ouyi OKEx account and conduct digital asset transactions safely and conveniently.
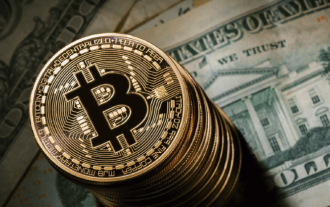
This article lists the top ten well-known Web3 trading platforms, including Binance, OKX, Gate.io, Kraken, Bybit, Coinbase, KuCoin, Bitget, Gemini and Bitstamp. The article compares the characteristics of each platform in detail, such as the number of currencies, trading types (spot, futures, options, NFT, etc.), handling fees, security, compliance, user groups, etc., aiming to help investors choose the most suitable trading platform. Whether it is high-frequency traders, contract trading enthusiasts, or investors who focus on compliance and security, they can find reference information from it.
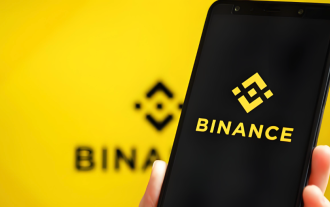
This article provides a complete guide to login and registration on Binance PC version. First, we explained in detail the steps for logging in Binance PC version: search for "Binance Official Website" in the browser, click the login button, enter the email and password (enable 2FA to enter the verification code) to log in. Secondly, the article explains the registration process: click the "Register" button, fill in the email address, set a strong password, and verify the email address to complete the registration. Finally, the article also emphasizes account security, reminding users to pay attention to the official domain name, network environment, and regularly updating passwords to ensure account security and better use of various functions provided by Binance PC version, such as viewing market conditions, conducting transactions and managing assets.

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
