Java Development: How to Optimize Your Code Performance
Java Development: How to Optimize Your Code Performance
In daily software development, we often encounter situations where we need to optimize code performance. Optimizing code performance can not only improve program execution efficiency, but also reduce resource consumption and improve user experience. This article will introduce some common optimization techniques, combined with specific code examples, to help readers better understand and apply them.
- Use appropriate data structures
Choosing the appropriate data structure is the key to improving code performance. Different data structures have different advantages and disadvantages in different scenarios. For example, ArrayList is suitable for scenarios where random access is frequent, while LinkedList is suitable for scenarios where elements are frequently inserted and deleted. Therefore, when using data structures, you must choose an appropriate data structure based on actual needs to avoid unnecessary performance losses.
Sample code:
//Use ArrayList to store data
List
for (int i = 0; i < ; 10000; i ) {
arrayList.add(i);
}
// Use LinkedList to store data
List
for (int i = 0; i < 10000; i ) {
linkedList.add(i);
}
- Avoid using loop nesting
Loop nesting can cause performance problems One of the common reasons. When there are multiple levels of nested loops, the execution time of the program increases exponentially. Therefore, nested loops should be avoided whenever possible, especially when dealing with large data volumes.
Sample code:
// Loop nesting example
for (int i = 0; i < 1000; i ) {
for (int j = 0; j < 1000; j++) { // 执行一些操作 }
}
- Reasonable use of cache
Cache can effectively reduce the number of accesses to underlying resources and improve code execution efficiency. In Java development, you can use some caching frameworks, such as Guava, Ehcache, etc., to improve program performance. At the same time, you can also use some local caches, such as HashMap, ConcurrentHashMap, etc., to cache some calculation results to avoid repeated calculations.
Sample code:
// Using Guava caching framework
LoadingCache
.maximumSize(1000) .expireAfterWrite(10, TimeUnit.MINUTES) .build( new CacheLoader<Integer, String>() { public String load(Integer key) { // 执行一些复杂的计算 return "result"; } });
// Get from cache Data
String result = cache.get(1);
- Multi-threaded concurrent processing
Multi-threaded concurrency can improve the parallelism and execution efficiency of the program. Some independent tasks can be assigned to different threads for execution, thereby achieving parallel processing of tasks. In Java development, multi-thread concurrency can be achieved by using thread pool, multi-threading and other technologies. At the same time, it is important to note that in multi-thread concurrency scenarios, access to shared resources must be synchronized to avoid concurrency security issues.
Sample code:
// Create a thread pool
ExecutorService executorService = Executors.newFixedThreadPool(10);
// Submit a task to the thread pool
executorService .submit(new Runnable() {
public void run() { // 执行任务 }
});
- Avoid excessive IO operations
IO operations are usually the most efficient in program execution One of the bottlenecks. When performing IO operations, try to reduce the number of accesses to underlying resources and use buffers to read or write data in batches. At the same time, caching technology should be rationally used to cache some frequently read data to reduce the frequency of IO operations.
Sample code:
// Read files using buffer
BufferedReader reader = new BufferedReader(new FileReader("test.txt"));
char[] buffer = new char[1024];
int length;
while ((length = reader.read(buffer)) != -1) {
// 执行一些操作
}
reader.close() ;
Conclusion
By optimizing code performance, the execution efficiency and user experience of the program can be improved. This article introduces some common optimization techniques and gives specific code examples. I hope readers can benefit from them and apply them in actual development. At the same time, optimizing code performance is not a one-time task and requires continuous testing and adjustment to achieve the best performance results. I wish readers success in optimizing code performance!
The above is the detailed content of Java Development: How to Optimize Your Code Performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
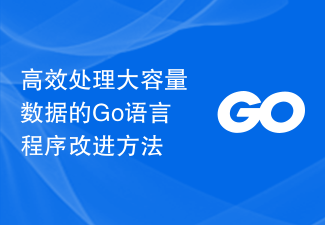
The method of optimizing Go language programs to process large-capacity data requires specific code examples. Overview: As the size of data continues to grow, large-scale data processing has become an important topic in modern software development. As an efficient and easy-to-use programming language, Go language can also well meet the needs of large-capacity data processing. This article will introduce some methods to optimize Go language programs to handle large volumes of data, and provide specific code examples. 1. Batch processing of data When processing large-capacity data, one of the common optimization methods is to use batch processing of data.
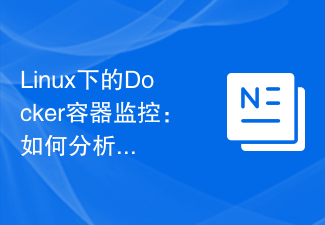
Docker container monitoring under Linux: How to analyze and optimize the running efficiency of containers? Introduction: With the rapid development of container technology, more and more enterprises are beginning to use Docker to build and deploy applications. However, due to the characteristics of containers, container monitoring and performance optimization have become an important task. This article will introduce how to monitor and optimize the performance of Docker containers under Linux to improve the running efficiency of the containers. 1. Docker container monitoring tools: Under Linux, there are many tools

Java Development: How to Optimize Your Code Performance In daily software development, we often encounter situations where we need to optimize code performance. Optimizing code performance can not only improve program execution efficiency, but also reduce resource consumption and improve user experience. This article will introduce some common optimization techniques, combined with specific code examples, to help readers better understand and apply them. Use the right data structures Choosing the right data structures is key to improving the performance of your code. Different data structures have different advantages and disadvantages in different scenarios. For example, Arra
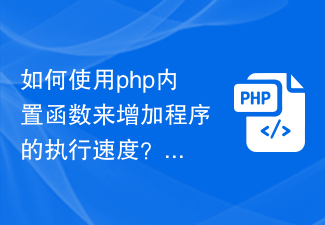
How to use PHP built-in functions to increase program execution speed? As the complexity of network applications increases, program execution speed becomes a very important consideration. As a widely used server-side scripting language, PHP is particularly critical for improving program execution speed. This article will introduce some techniques for using PHP's built-in functions to increase program execution speed, and provide specific code examples. Using String Processing Functions String processing is one of the operations that is often required in developing web applications. Use within PHP
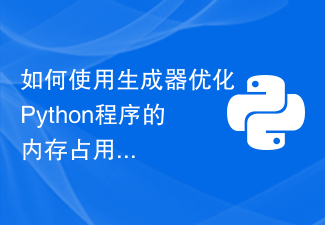
How to use generators to optimize the memory footprint of Python programs. As the amount of data continues to grow, memory footprint has become an important aspect of optimizing the performance of Python programs. The generator is a powerful tool in Python that can significantly reduce the memory footprint of the program and improve the efficiency of the program. This article will introduce how to use generators to optimize the memory footprint of Python programs and illustrate it with code examples. A generator is a special type of iterator that can generate results sequentially through a function.
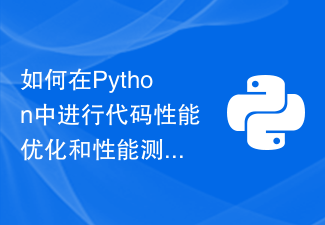
How to perform code performance optimization and performance testing in Python Introduction: When we write code, we often face the problem of slow code execution. For a complex program, efficiency improvements can bring significant performance improvements. This article will introduce how to perform code performance optimization and performance testing in Python, and give specific code examples. 1. Basic principles of code performance optimization: Algorithm optimization: Choose more efficient algorithms to reduce program complexity. Data structure optimization: Choose a data structure that is more suitable for the current problem.
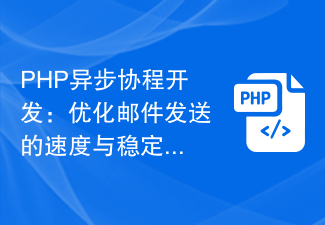
PHP asynchronous coroutine development: optimizing the speed and stability of email sending Introduction: In modern Internet applications, email sending is a very important function, whether it is user registration verification, order confirmation, password reset, etc., it is inseparable. Turn on email sending. However, traditional synchronous email sending methods are often inefficient and unstable when handling large amounts of email sending. In order to solve this problem, we can use PHP's asynchronous coroutine development to improve sending speed and stability by sending emails concurrently. This article will introduce in detail the use of PHP asynchronous
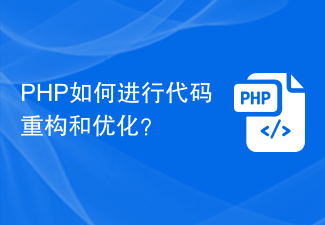
PHP is a programming language widely used in website development. However, as projects continue to iterate and develop, the code tends to become more complex and lengthy, which requires code reconstruction and optimization. This article will introduce methods and techniques for refactoring and optimizing PHP code. 1. The concept and purpose of code refactoring Code refactoring refers to the process of optimizing and improving existing code. The purpose of refactoring mainly includes the following points: Improve the readability and maintainability of the code; Optimize the performance and efficiency of the code; Reduce the duplication and redundancy of the code; Increase the scalability and flexibility of the code.
