How to use Vue to implement sliding unlock effects
How to use Vue to implement sliding unlock effects
In modern web applications, we often see various sliding unlock effects. The slide to unlock special effect is a way to achieve user interaction by sliding pages or elements to achieve specific purposes, such as unlocking and dragging the slider, switching pages, etc. In this article, we will discuss how to use the Vue framework to implement the slide to unlock effect and provide specific code examples.
- Create Vue project
First, we need to create a Vue project. Vue.js provides a scaffolding tool vue-cli, which can help us quickly build Vue projects. Use the following command to create a new Vue project:
$ npm install -g @vue/cli $ vue create slider-unlock
During the installation process, we need to select some options to configure our project. Let’s just choose the default option.
- Create a sliding unlock component
In the Vue project, we can create a separate component to implement the sliding unlocking effect. Create a file named SliderUnlock.vue in the src/components directory and add the following code:
<template> <div class="slider-unlock"> <div class="slider-bar" ref="sliderBar"></div> <div class="slider-button" :style="buttonStyle" ref="sliderButton"> <div></div> </div> </div> </template> <script> export default { data() { return { buttonLeft: 0, dragging: false, startOffset: 0, }; }, computed: { buttonStyle() { return { left: this.buttonLeft + "px", }; }, }, mounted() { this.$refs.sliderButton.addEventListener("mousedown", this.handleMouseDown); window.addEventListener("mousemove", this.handleMouseMove); window.addEventListener("mouseup", this.handleMouseUp); }, beforeDestroy() { this.$refs.sliderButton.removeEventListener("mousedown", this.handleMouseDown); window.removeEventListener("mousemove", this.handleMouseMove); window.removeEventListener("mouseup", this.handleMouseUp); }, methods: { handleMouseDown(event) { this.dragging = true; this.startOffset = event.pageX - this.buttonLeft; }, handleMouseMove(event) { if (this.dragging) { const offsetX = event.pageX - this.startOffset; this.buttonLeft = Math.max(0, Math.min(offsetX, this.$refs.sliderBar.offsetWidth - this.$refs.sliderButton.offsetWidth)); } }, handleMouseUp() { this.dragging = false; if (this.buttonLeft === this.$refs.sliderBar.offsetWidth - this.$refs.sliderButton.offsetWidth) { // 滑动成功,触发解锁事件 this.$emit("unlock"); } else { // 滑动失败,重置滑块位置 this.buttonLeft = 0; } }, }, }; </script> <style scoped> .slider-unlock { position: relative; width: 300px; height: 40px; border: 1px solid #ccc; border-radius: 20px; overflow: hidden; } .slider-bar { position: absolute; top: 50%; transform: translateY(-50%); width: 100%; height: 4px; background-color: #ccc; } .slider-button { position: absolute; top: 50%; transform: translateY(-50%); width: 40px; height: 40px; background-color: #2196f3; border-radius: 50%; cursor: pointer; transition: left 0.3s; } .slider-button div { position: relative; left: 50%; top: 50%; transform: translate(-50%, -50%); width: 20px; height: 20px; background-color: #fff; border-radius: 50%; } </style>
In this component, we created a sliding unlock bar and a slider. By listening to mouse events, when the slider is dragged, we change the position of the slider based on the mouse offset. At the same time, we will monitor the position of the slider and trigger the unlock event when the slider reaches the end position of the sliding unlock bar.
- Using the slide to unlock component
In the App.vue file, we can use the slide to unlock component we just created. Add the following code in the template paragraph:
<template> <div class="app"> <SliderUnlock @unlock="handleUnlock"></SliderUnlock> </div> </template>
In the script paragraph, we add the handleUnlock method to handle the unlock event:
<script> import SliderUnlock from "./components/SliderUnlock.vue"; export default { components: { SliderUnlock, }, methods: { handleUnlock() { alert("解锁成功!"); }, }, }; </script>
- Run the code
Finally, we can run the Vue project to see the effect. Run the following command in the terminal to start the local development server:
$ npm run serve
Then open the browser and visit http://localhost:8080 to view the sliding unlock effect.
Summary
In this article, we explored how to use the Vue framework to implement the slide to unlock effect and provided specific code examples. By creating a slide to unlock component, we can trigger corresponding events based on the user's sliding action. This approach can enhance the user interaction experience and increase the attractiveness of the application. I hope this article will help you understand how to use Vue to implement the slide to unlock effect.
The above is the detailed content of How to use Vue to implement sliding unlock effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


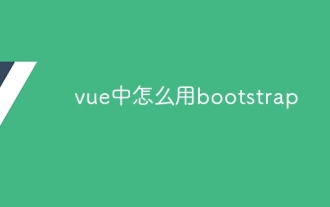
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
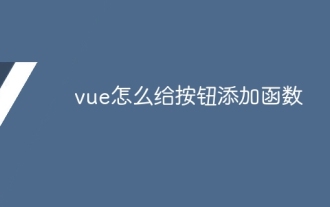
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
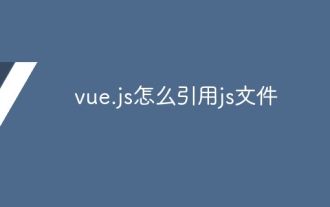
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
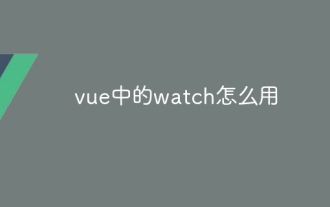
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
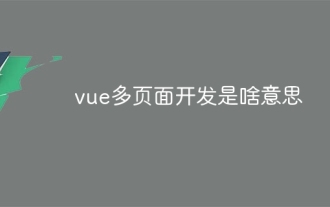
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
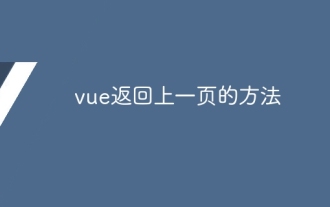
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
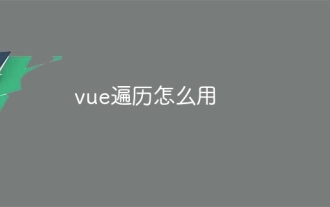
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
