How to implement a simple data cleaning function using MySQL and Java
How to use MySQL and Java to implement a simple data cleaning function
Overview:
Before conducting data analysis and machine learning, data cleaning is a very important A step of. Data cleaning can help us deal with problems such as missing values, outliers, and duplicate values, thereby improving the accuracy and reliability of our data. This article will introduce how to use MySQL and Java to implement a simple data cleaning function, and provide some specific code examples.
Step 1: Data Import
First, we need to import the original data into the MySQL database. You can use MySQL command line tools or graphical interface tools (such as Navicat) to import data. Suppose we have a data table named "original_data" which contains various incomplete, duplicate and abnormal data.
Step 2: Create a new table to store the cleaned data
Next, we need to create a new table to store the cleaned data. You can use the following SQL statement to create a new table, such as "cleaned_data":
CREATE TABLE cleaned_data (
id INT AUTO_INCREMENT PRIMARY KEY,
column1 VARCHAR(255),
column2 INT ,
column3 DOUBLE,
...
);
Step 3: Write Java code to connect to the MySQL database
Use Java programming language to connect to the MySQL database, and import the required JDBC Driver package.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnector {
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
}
Step 4: Data Cleaning
Next, we can write some code to implement the logic of data cleaning. Below is an example that demonstrates how to handle duplicate records in a data table.
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DataCleaner {
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
}
The above code demonstrates how to use Java to select unique data from the original data table and insert it into the cleaned data table.
You can write more code logic during the cleaning process according to your actual needs, such as handling missing values, outliers, etc.
Conclusion:
By using MySQL and Java, we can implement a simple data cleaning function. This process can help us deal with issues such as duplicate values in the data and improve our accuracy and reliability of the data. I hope the examples and ideas provided in this article will be helpful to you.
The above is the detailed content of How to implement a simple data cleaning function using MySQL and Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










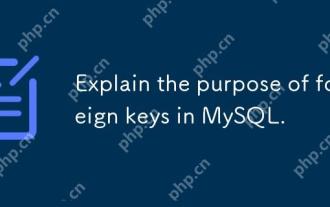
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
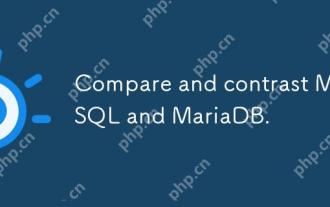
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.

MySQL and phpMyAdmin can be effectively managed through the following steps: 1. Create and delete database: Just click in phpMyAdmin to complete. 2. Manage tables: You can create tables, modify structures, and add indexes. 3. Data operation: Supports inserting, updating, deleting data and executing SQL queries. 4. Import and export data: Supports SQL, CSV, XML and other formats. 5. Optimization and monitoring: Use the OPTIMIZETABLE command to optimize tables and use query analyzers and monitoring tools to solve performance problems.
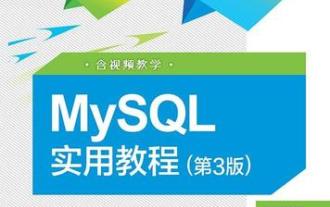
To safely and thoroughly uninstall MySQL and clean all residual files, follow the following steps: 1. Stop MySQL service; 2. Uninstall MySQL packages; 3. Clean configuration files and data directories; 4. Verify that the uninstallation is thorough.
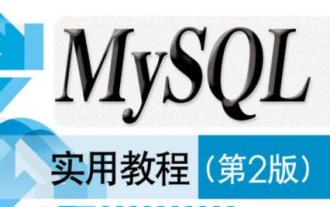
In MySQL, add fields using ALTERTABLEtable_nameADDCOLUMNnew_columnVARCHAR(255)AFTERexisting_column, delete fields using ALTERTABLEtable_nameDROPCOLUMNcolumn_to_drop. When adding fields, you need to specify a location to optimize query performance and data structure; before deleting fields, you need to confirm that the operation is irreversible; modifying table structure using online DDL, backup data, test environment, and low-load time periods is performance optimization and best practice.

HTML5 brings five key improvements: 1. Semantic tags improve code clarity and SEO effects; 2. Multimedia support simplifies video and audio embedding; 3. Form enhancement simplifies verification; 4. Offline and local storage improves user experience; 5. Canvas and graphics functions enhance the visualization of web pages.
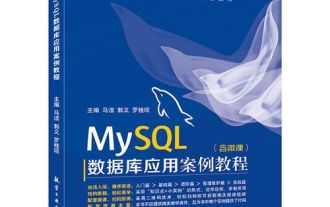
Efficient methods for batch inserting data in MySQL include: 1. Using INSERTINTO...VALUES syntax, 2. Using LOADDATAINFILE command, 3. Using transaction processing, 4. Adjust batch size, 5. Disable indexing, 6. Using INSERTIGNORE or INSERT...ONDUPLICATEKEYUPDATE, these methods can significantly improve database operation efficiency.
