


How to use Redis and PowerShell to develop distributed task scheduling functions
How to use Redis and PowerShell to develop distributed task scheduling functions
Nowadays, with the development of cloud computing and big data technology, distributed systems have become daily development an integral part of. In distributed systems, a common requirement is to implement task distribution and scheduling. This article will introduce how to use Redis and PowerShell to develop distributed task scheduling functions and provide specific code examples.
1. Introduction to Redis
Redis is an open source in-memory data storage system that is commonly used in scenarios such as caching, queuing, and distributed scheduling. It supports a variety of data structures such as strings, lists, hash tables, sets and ordered sets, etc. Redis provides powerful data operation functions, making it an ideal choice for distributed task scheduling.
2. Introduction to PowerShell
PowerShell is a cross-platform scripting language and command line tool that is widely used in Windows system management and automation tasks. PowerShell provides a rich set of commands and APIs to support interaction with various external systems, including Redis.
3. Use Redis to implement distributed task scheduling
In Redis, we can use ordered collections and publish/subscribe mechanisms to implement distributed task scheduling.
- Create task queue
First, we need to create an ordered collection to store tasks to be executed. Each task has a unique identifier and an execution timestamp. We can add tasks to an ordered collection using Redis’ ZADD command.
Sample code:
$timestamp = [DateTime]::Now.Ticks $taskId = "task1" $redisCmd = "ZADD task_queue $timestamp $taskId" Invoke-Expression -Command $redisCmd
- Listening to the task queue
Next, we need to create a subscriber to listen to the task queue. When a new task is added to the queue, the subscriber will be notified and perform corresponding operations.
Sample code:
$redisCmd = "SUBSCRIBE task_channel" Invoke-Expression -Command $redisCmd
- Execute tasks
In the task executor, we can execute the corresponding tasks by consuming the task queue. After executing the task, we can use Redis's ZREM command to remove the task from the queue.
Sample code:
$taskId = "task1" $redisCmd = "ZREM task_queue $taskId" Invoke-Expression -Command $redisCmd
4. Use PowerShell to interact with Redis
In PowerShell, we can use the StackExchange.Redis module to interact with Redis.
- Install StackExchange.Redis module
Use PowerShell Gallery to install the StackExchange.Redis module.
Command:
Install-Module -Name StackExchange.Redis -AllowPrerelease
- Connect to the Redis server
In the PowerShell script, we can use the Client object of the StackExchange.Redis module to connect to the Redis server.
Sample code:
$redis = [StackExchange.Redis.ConnectionMultiplexer]::Connect("localhost:6379")
- Execute Redis commands
Use the Database object of the StackExchange.Redis module to execute various Redis commands, such as SET, GET, ZADD and ZREM et al.
Sample code:
$redisDb = $redis.GetDatabase() $redisDb.StringSet("key", "value") $value = $redisDb.StringGet("key") $redisDb.SortedSetAdd("task_queue", $timestamp, $taskId) $redisDb.SortedSetRemove("task_queue", $taskId)
5. Complete task scheduling example
The following is a complete example code for Redis and PowerShell distributed task scheduling:
$redis = [StackExchange.Redis.ConnectionMultiplexer]::Connect("localhost:6379") $redisDb = $redis.GetDatabase() function AddTaskToQueue($taskId) { $timestamp = [DateTime]::Now.Ticks $redisDb.SortedSetAdd("task_queue", $timestamp, $taskId) } $taskChannel = $redis.GetSubscriber().Subscribe("task_channel") $taskChannel.OnMessage({ $taskId = $_.Message # 执行任务操作 Write-Host "Task $taskId is executing..." Start-Sleep -Seconds 5 # 移除任务 $redisDb.SortedSetRemove("task_queue", $taskId) Write-Host "Task $taskId is completed." }) # 添加任务到队列 AddTaskToQueue("task1") AddTaskToQueue("task2")
In the above example, we created a channel named task_channel and listened to the channel through a subscriber. When a new task is added to the queue, the subscriber will be notified and perform corresponding operations. At the same time, we also provide an AddTaskToQueue function to add tasks to the queue.
Summary
By using Redis and PowerShell, we can easily implement distributed task scheduling functions. With the ordered collection and publish/subscribe mechanism of Redis, we can manage task queues and execute tasks through the power of PowerShell. I hope this article can help readers understand and master the development skills of distributed task scheduling.
The above is the detailed content of How to use Redis and PowerShell to develop distributed task scheduling functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
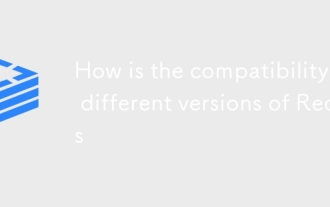
This article addresses Redis version compatibility challenges. Major version upgrades pose significant compatibility risks due to changes in commands, data structures, and configuration. The article emphasizes thorough testing, utilizing redis-cli

The article discusses choosing shard keys in Redis Cluster, emphasizing their impact on performance, scalability, and data distribution. Key issues include ensuring even data distribution, aligning with access patterns, and avoiding common mistakes l
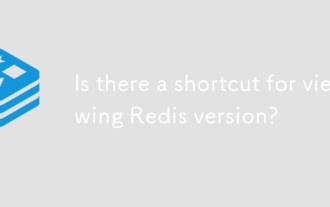
This article details methods for checking Redis server versions. It compares using redis-cli --version and INFO server, examining configuration files, process lists, and package managers. The INFO server command within redis-cli is identified as t

This article details methods to check Redis server versions via the command line. The primary method, redis-cli INFO SERVER, efficiently retrieves version information from the server's output, providing a direct and reliable solution. Alternative a
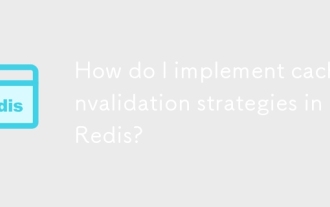
The article discusses strategies for implementing and managing cache invalidation in Redis, including time-based expiration, event-driven methods, and versioning. It also covers best practices for cache expiration and tools for monitoring and automat
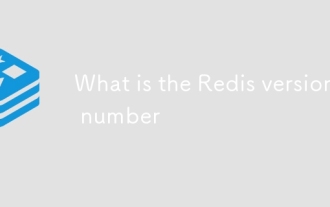
This article details Redis version numbers, their string data type, and methods for checking versions (using redis-cli, configuration files, or programmatically). It also explores differences between major versions, focusing on performance enhanceme
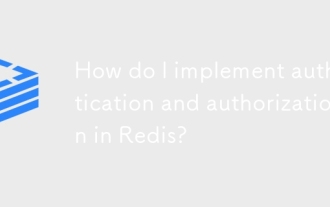
The article discusses implementing authentication and authorization in Redis, focusing on enabling authentication, using ACLs, and best practices for securing Redis. It also covers managing user permissions and tools to enhance Redis security.
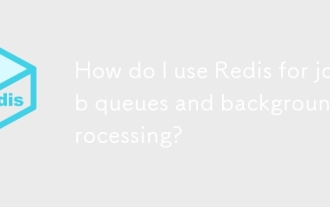
The article discusses using Redis for job queues and background processing, detailing setup, job definition, and execution. It covers best practices like atomic operations and job prioritization, and explains how Redis enhances processing efficiency.
