Java Development: How to Code Security and Vulnerability Protection
Java Development: Code Security and Vulnerability Protection
Abstract:
In the current Internet era, code security and vulnerability protection are crucial to Java development. This article will introduce some common code security risks and vulnerabilities and provide corresponding solutions. At the same time, concrete code examples demonstrate how to prevent these security issues.
- Password security
Passwords are common security risks and are vulnerable to attacks such as brute force cracking and credential stuffing. In order to ensure the security of passwords, here are a few suggestions:
(1) Use complex password algorithms: such as SHA-256, BCrypt, etc., and avoid using the one-way encryption algorithm MD5.
(2) Add salt to store passwords: Improve the security of password storage by adding a random string (salt) to the password and then encrypting it.
(3) Use verification code: When users log in and register, use verification code to protect user accounts from malicious attacks.
Sample code:
import org.apache.commons.codec.digest.DigestUtils; import org.apache.commons.lang3.RandomStringUtils; public class PasswordUtils { private static final int SALT_LENGTH = 16; public static String encryptPassword(String password) { String salt = RandomStringUtils.randomAlphanumeric(SALT_LENGTH); String encryptedPassword = DigestUtils.sha256Hex(password + salt); return salt + encryptedPassword; } public static boolean checkPassword(String inputPassword, String storedPassword) { String salt = storedPassword.substring(0, SALT_LENGTH); String encryptedInputPassword = DigestUtils.sha256Hex(inputPassword + salt); return storedPassword.equals(salt + encryptedInputPassword); } }
- SQL injection attack
SQL injection attack refers to destroying the security of the database by users entering malicious SQL code. Here are several ways to avoid SQL injection attacks:
(1) Never splice SQL statements directly, but use parameterized queries or prepared statements.
(2) Input verification and filtering: Verify, filter and escape user input to ensure that there is no malicious code in user input.
Sample code:
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class DatabaseUtils { public static void main(String[] args) { String username = "admin'; DROP TABLE users; --"; String password = "pass123"; Connection conn = null; PreparedStatement pstmt = null; ResultSet rs = null; try { conn = getConnection(); String sql = "SELECT * FROM users WHERE username = ? AND password = ?"; pstmt = conn.prepareStatement(sql); pstmt.setString(1, username); pstmt.setString(2, password); rs = pstmt.executeQuery(); } catch (SQLException e) { e.printStackTrace(); } finally { closeResources(conn, pstmt, rs); } } private static Connection getConnection() throws SQLException { // 获取数据库连接 return null; } private static void closeResources(Connection conn, PreparedStatement pstmt, ResultSet rs) { // 关闭数据库连接和其他资源 } }
- Cross-site scripting attack (XSS)
XSS attack means that the attacker obtains users by injecting malicious script code on the website of sensitive information. The following are several methods to prevent XSS attacks:
(1) Validate and filter user input, especially escaping special characters.
(2) When outputting data to the page, use appropriate encoding methods for processing.
Sample code:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>XSS Prevention</title> </head> <body> <h1 id="Welcome-StringEscapeUtils-escapeHtml-request-getParameter-name">Welcome <%= StringEscapeUtils.escapeHtml4(request.getParameter("name")) %></h1> </body> </html>
- File upload vulnerability
The file upload vulnerability means that an attacker can execute remote commands by uploading a file containing malicious code. The following are several methods to prevent file upload vulnerabilities:
(1) Strictly limit the type and size of uploaded files.
(2) Use random file names and secure storage paths.
Sample code:
import java.io.File; import java.io.IOException; import java.util.UUID; public class FileUploadUtils { public static void main(String[] args) { String fileName = "evil_script.jsp"; File file = new File("/uploads/" + UUID.randomUUID().toString() + ".jpg"); try { file.createNewFile(); // 处理上传文件的逻辑 } catch (IOException e) { e.printStackTrace(); } } }
Conclusion:
Code security and vulnerability protection are crucial to Java development. This article introduces solutions to password security, SQL injection attacks, cross-site scripting attacks, and file upload vulnerabilities, and provides corresponding code examples. Developers should always be vigilant and take appropriate security measures to prevent code security issues and vulnerabilities.
The above is the detailed content of Java Development: How to Code Security and Vulnerability Protection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


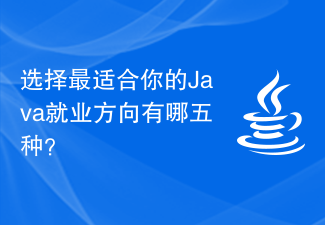
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
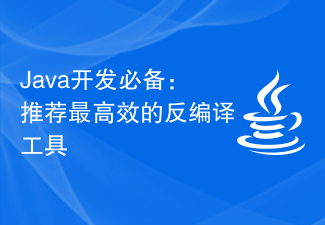
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source
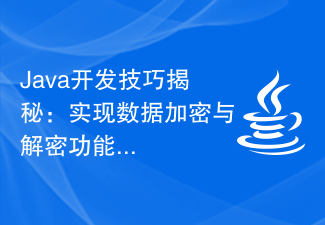
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
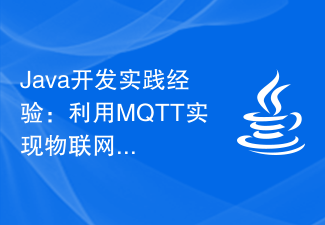
With the development of IoT technology, more and more devices are able to connect to the Internet and communicate and interact through the Internet. In the development of IoT applications, the Message Queuing Telemetry Transport Protocol (MQTT) is widely used as a lightweight communication protocol. This article will introduce how to use Java development practical experience to implement IoT functions through MQTT. 1. What is MQT? QTT is a message transmission protocol based on the publish/subscribe model. It has a simple design and low overhead, and is suitable for application scenarios that quickly transmit small amounts of data.

As a very popular programming language, Java has always been favored by everyone. When I first started learning Java development, I once encountered a problem-how to build a message subscription system. In this article, I will share my experience in building a message subscription system from scratch, hoping to be helpful to other Java beginners. Step 1: Choose a suitable message queue To build a message subscription system, you first need to choose a suitable message queue. The more popular message queues currently on the market include ActiveMQ,
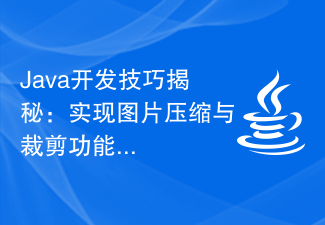
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
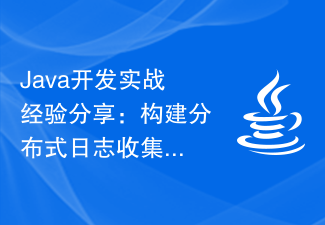
Sharing practical experience in Java development: Building a distributed log collection function Introduction: With the rapid development of the Internet and the emergence of large-scale data, the application of distributed systems is becoming more and more widespread. In distributed systems, log collection and analysis are very important. This article will share the experience of building distributed log collection function in Java development, hoping to be helpful to readers. 1. Background introduction In a distributed system, each node generates a large amount of log information. These log information are useful for system performance monitoring, troubleshooting and data analysis.
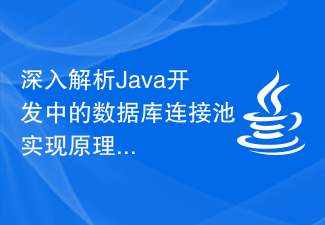
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
