Java development: How to use JVM tuning and memory optimization
Java Development: How to use JVM tuning and memory optimization
Introduction:
In the Java development process, the performance optimization of JVM (Java Virtual Machine) and Memory management is a very important link. Optimizing the use of JVM and memory can not only improve the execution efficiency and stability of the application, but also reduce the waste of resources. This article will introduce some commonly used JVM tuning and memory optimization techniques, and provide specific code examples to help developers better perform JVM tuning and memory optimization.
1. JVM tuning skills:
- Set JVM startup parameters: By adjusting the JVM startup parameters, you can perform some basic optimizations on the JVM. The following are some commonly used JVM startup parameter examples:
java -Xms512m -Xmx1024m -XX:PermSize=256m -XX:MaxPermSize=512m -XX:+UseParallelGC -XX:ParallelGCThreads=4 Main
-Xms512m
and-Xmx1024m
set the initial size and maximum size of the Java heap respectively. ;-XX:PermSize=256m
and-XX:MaxPermSize=512m
set the initial size and maximum size of the permanent generation respectively;-XX: UseParallelGC
and-XX:ParallelGCThreads=4
Enable the parallel garbage collector and specify the use of 4 threads.
- Tuning garbage collection performance: Garbage collection is a core function of the JVM. Properly adjusting the parameters of the garbage collector can improve the performance of garbage collection. The following are some examples of commonly used garbage collector parameters:
-XX:+UseSerialGC : 使用串行垃圾回收器 -XX:+UseParallelGC : 使用并行垃圾回收器 -XX:+UseConcMarkSweepGC : 使用并发标记-清除垃圾回收器 -XX:+UseG1GC : 使用G1垃圾回收器
2. Memory optimization skills:
- Use the smallest objects: In order to save memory, try to use smaller objects object. Avoid using unnecessary wrapper classes and use basic types to meet your needs.
int num = 1; // 使用int类型 Integer num = new Integer(1); // 避免使用包装类
- Avoid excessive use of the constant pool: In Java, the constant pool is used to store string constants and basic type constants. When a large number of string constants are used in a program, the memory usage of the constant pool will be too large. The usage of the constant pool can be appropriately reduced.
String str1 = "abc"; // 使用常量 String str2 = new String("abc"); // 避免多余的String对象
- Use cache: For some objects that need to be created frequently, you can use cache to avoid repeated creation and reduce memory usage.
public class ObjectPool { private static final int MAX_SIZE = 100; private static final List<Object> pool = new ArrayList<>(); public static synchronized Object getObject() { if (pool.isEmpty()) { return new Object(); } else { return pool.remove(pool.size() - 1); } } public static synchronized void releaseObject(Object obj) { if (pool.size() < MAX_SIZE) { pool.add(obj); } } }
Using a cache pool can effectively reduce the overhead of frequently creating and destroying objects.
- Release resources in a timely manner: During the running of the program, used objects are released in a timely manner to avoid memory leaks.
public class ResourceHolder { private static final Resource resource = new Resource(); public static Resource getResource() { return resource; } public static void releaseResource() { resource.close(); } }
Release the resource by calling the releaseResource()
method when the resource is no longer needed.
Conclusion:
Through the use of JVM tuning and memory optimization, the performance and stability of Java applications can be improved and the waste of resources can be reduced. This article introduces some common JVM tuning and memory optimization techniques, and provides specific code examples, hoping to be helpful to developers. In short, in the actual development process, it is necessary to select an appropriate optimization strategy based on specific application requirements and system environment, and conduct corresponding testing and adjustments.
The above is the detailed content of Java development: How to use JVM tuning and memory optimization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
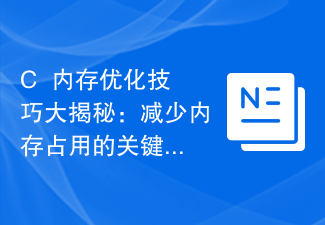
C++ is an efficient and powerful programming language, but when processing large-scale data or running complex programs, memory optimization becomes an issue that developers cannot ignore. Properly managing and reducing memory usage can improve program performance and reliability. This article will reveal some key tips for reducing memory footprint in C++ to help developers build more efficient applications. Use appropriate data types In C++ programming, choosing the appropriate data type is an important step in reducing memory usage. For example, if you only need to represent a small range of integers, you can use
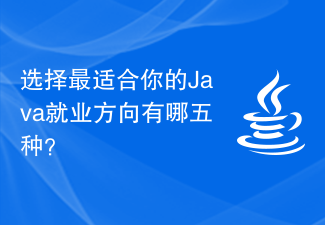
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
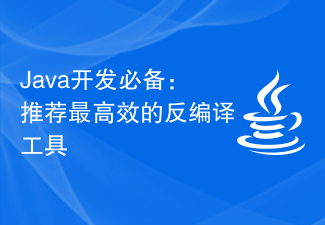
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source

As a very popular programming language, Java has always been favored by everyone. When I first started learning Java development, I once encountered a problem-how to build a message subscription system. In this article, I will share my experience in building a message subscription system from scratch, hoping to be helpful to other Java beginners. Step 1: Choose a suitable message queue To build a message subscription system, you first need to choose a suitable message queue. The more popular message queues currently on the market include ActiveMQ,
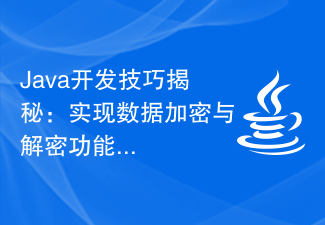
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
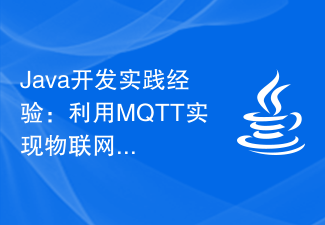
With the development of IoT technology, more and more devices are able to connect to the Internet and communicate and interact through the Internet. In the development of IoT applications, the Message Queuing Telemetry Transport Protocol (MQTT) is widely used as a lightweight communication protocol. This article will introduce how to use Java development practical experience to implement IoT functions through MQTT. 1. What is MQT? QTT is a message transmission protocol based on the publish/subscribe model. It has a simple design and low overhead, and is suitable for application scenarios that quickly transmit small amounts of data.
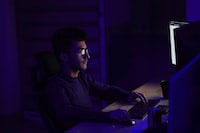
SpringBoot is a popular Java framework known for its ease of use and rapid development. However, as the complexity of the application increases, performance issues can become a bottleneck. In order to help you create a springBoot application as fast as the wind, this article will share some practical performance optimization tips. Optimize startup time Application startup time is one of the key factors of user experience. SpringBoot provides several ways to optimize startup time, such as using caching, reducing log output, and optimizing classpath scanning. You can do this by setting spring.main.lazy-initialization in the application.properties file
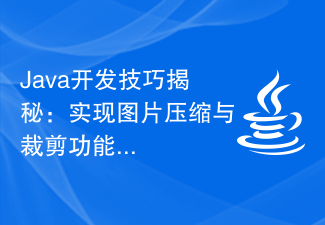
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
