PHP development: How to implement image compression function
PHP development: How to implement image compression function
Abstract: In web development, it is often necessary to process a large number of images. In order to improve web page loading speed and save bandwidth, it is necessary to Compression of images is essential. This article will introduce how to use PHP to implement image compression function and provide specific code examples.
Introduction:
With the rapid development of the Internet, pictures play an increasingly important role in web design. However, a large number of high-resolution images will take longer to load the web page and give users a bad experience. In order to solve this problem, we can use PHP to compress the image. The following will introduce how to use PHP to implement image compression function.
Step 1: Get the image file
First, we need to get the image file from the image uploaded by the user. You can use the $_FILES
global variable to obtain uploaded file information. For example, the following code can get the size, type and temporary storage location of the uploaded file:
$fileSize = $_FILES['image']['size']; $fileType = $_FILES['image']['type']; $tmpFilePath = $_FILES['image']['tmp_name'];
Step 2: Create an image object
Next, we need to create an image object using the PHP GD library. The GD library is an image processing extension for PHP that provides a series of image processing functions. First, we need to create the corresponding image object according to the image type. The following code can create an uploaded image file as an image object:
$sourceImage = null; // 根据图像类型创建图像对象 if ($fileType == 'image/jpeg' || $fileType == 'image/pjpeg') { $sourceImage = imagecreatefromjpeg($tmpFilePath); } elseif ($fileType == 'image/png') { $sourceImage = imagecreatefrompng($tmpFilePath); } elseif ($fileType == 'image/gif') { $sourceImage = imagecreatefromgif($tmpFilePath); }
Step 3: Set the target image size
Before performing image compression, we need to set the size of the target image. You can set it here according to your needs. For example, you can compress the image size to a specific width or height, or reduce it proportionally. The following code can set the size of the target image:
// 目标图片的宽度和高度 $destWidth = 800; $destHeight = 600; // 创建目标图片对象 $destImage = imagecreatetruecolor($destWidth, $destHeight);
Step 4: Image compression
After we have the image object and target size, we can compress the image. You can use the imagecopyresampled
function to scale the source image onto the target image. The following code can compress the source image to the target image:
// 进行图片压缩 imagecopyresampled($destImage, $sourceImage, 0, 0, 0, 0, $destWidth, $destHeight, imagesx($sourceImage), imagesy($sourceImage));
Step 5: Save the compressed image
Finally, we save the compressed image to a specific location on the server for subsequent use . Image objects can be saved as JPEG picture files using the imagejpeg
function. The following code can save the compressed image to the specified location:
// 保存压缩后的图片 $destPath = 'compressed_image.jpg'; imagejpeg($destImage, $destPath, 80);
Summary:
Through the above steps, we can use PHP to implement the image compression function. First get the uploaded image file, then create an image object, set the target image size, perform image compression, and finally save the compressed image to the server. This can improve image loading speed and save bandwidth in web development, providing users with a better experience.
Appendix: Complete code example
<?php // 获取图片文件 $fileSize = $_FILES['image']['size']; $fileType = $_FILES['image']['type']; $tmpFilePath = $_FILES['image']['tmp_name']; // 创建图像对象 $sourceImage = null; // 根据图像类型创建图像对象 if ($fileType == 'image/jpeg' || $fileType == 'image/pjpeg') { $sourceImage = imagecreatefromjpeg($tmpFilePath); } elseif ($fileType == 'image/png') { $sourceImage = imagecreatefrompng($tmpFilePath); } elseif ($fileType == 'image/gif') { $sourceImage = imagecreatefromgif($tmpFilePath); } // 目标图片的宽度和高度 $destWidth = 800; $destHeight = 600; // 创建目标图片对象 $destImage = imagecreatetruecolor($destWidth, $destHeight); // 进行图片压缩 imagecopyresampled($destImage, $sourceImage, 0, 0, 0, 0, $destWidth, $destHeight, imagesx($sourceImage), imagesy($sourceImage)); // 保存压缩后的图片 $destPath = 'compressed_image.jpg'; imagejpeg($destImage, $destPath, 80); ?>
The above is the detailed content of PHP development: How to implement image compression function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


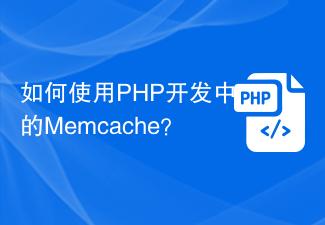
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
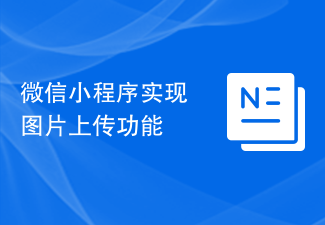
WeChat applet implements picture upload function With the development of mobile Internet, WeChat applet has become an indispensable part of people's lives. WeChat mini programs not only provide a wealth of application scenarios, but also support developer-defined functions, including image upload functions. This article will introduce how to implement the image upload function in the WeChat applet and provide specific code examples. 1. Preparatory work Before starting to write code, we need to download and install the WeChat developer tools and register as a WeChat developer. At the same time, you also need to understand WeChat

How to use Laravel to implement user rights management functions With the development of web applications, user rights management has become more and more important in many projects. Laravel, as a popular PHP framework, provides many powerful tools and functions for handling user rights management. This article will introduce how to use Laravel to implement user rights management functions and provide specific code examples. Database design First, we need to design a database model to store the relationship between users, roles and permissions. To make things easier we will make
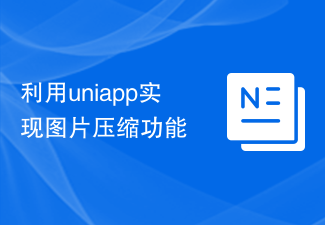
Using uniapp to realize image compression function With the improvement of mobile phone camera functions, we often take a large number of photos in our daily life. However, these high-resolution photos take up storage space on your phone, making it slow and easy to fill up. In order to solve this problem, we can use the relevant technology in uniapp to implement the image compression function, compress the image to a smaller file size, and retain appropriate pixels and image quality. Below we will introduce in detail how to implement the image compression function in uniapp. Step 1: Introduce relevant
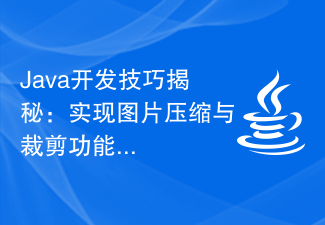
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
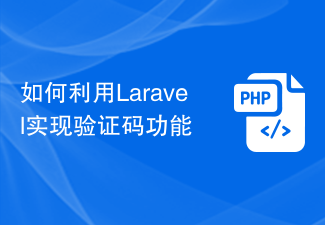
Laravel is a popular PHP web framework that provides many conveniences for web application development. One of the very important functions is the verification code function. CAPTCHA is a mechanism for validating human actions, and it can be used in many web application scenarios. In this article, we will use Laravel as an example to introduce how to implement the verification code function and provide specific code examples. Generate verification code images In Laravel, the way to generate verification code images is usually to use PHP's GD library.
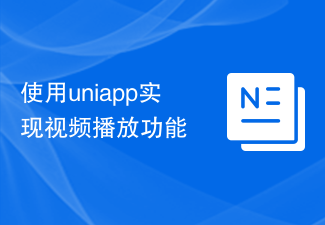
Use uniapp to implement video playback function Uniapp is a cross-platform development framework developed based on Vue.js, which can quickly build multi-terminal applications. If you need to implement the video playback function in Uniapp, you can use the video component of uni-app to implement it. The following will give specific code examples to introduce how to use the video component of uni-app to implement the video playback function in Uniapp. First, you need to create a new page in the pages directory of the uniapp project, such as V
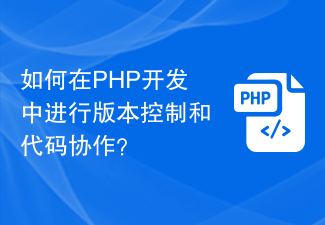
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
