


PHP development skills: How to implement data import and export functions
PHP development skills: How to implement data import and export functions
Importing and exporting data is one of the commonly used functions in web development. In many projects, we need to import data from external data sources into the database, or export data in the database to files in different formats. This article will introduce how to use PHP to implement data import and export functions, and give specific code examples.
1. Database import function
The database import function is usually used to import data from external data sources into the database. The following is a sample code that uses PHP to implement the database import function:
<?php // 导入数据库配置文件 require_once 'config.php'; // 连接数据库 $conn = new mysqli(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); // 检测数据库连接是否成功 if ($conn->connect_error) { die("数据库连接失败:" . $conn->connect_error); } // 读取外部数据源文件,例如CSV文件 $csvFile = 'data.csv'; $handle = fopen($csvFile, 'r'); // 逐行读取CSV文件内容,并插入数据库中 while (($data = fgetcsv($handle, 1000, ',')) !== false) { $sql = "INSERT INTO `tablename` (`column1`, `column2`, `column3`) VALUES ('".$data[0]."', '".$data[1]."', '".$data[2]."')"; if ($conn->query($sql) === false) { echo "数据导入失败:" . $conn->error; } } // 关闭文件句柄 fclose($handle); // 关闭数据库连接 $conn->close(); ?>
In the above code, we first introduce the database configuration file and then establish the database connection. Next, we use the fopen function to open the external data source file and the fgetcsv function to read the CSV file line by line. The process of inserting the read data into the database is implemented by assembling SQL statements, and finally closing the file handle and database connection.
2. Database export function
The database export function is usually used to export data in the database to files in different formats, such as CSV, Excel, etc. The following is a sample code that uses PHP to implement the database export function:
<?php // 导入数据库配置文件 require_once 'config.php'; // 连接数据库 $conn = new mysqli(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME); // 检测数据库连接是否成功 if ($conn->connect_error) { die("数据库连接失败:" . $conn->connect_error); } // 查询数据库中的数据 $sql = "SELECT * FROM `tablename`"; $result = $conn->query($sql); if ($result->num_rows > 0) { // 创建CSV文件并写入表头 $filename = 'data.csv'; $handle = fopen($filename, 'w'); fputcsv($handle, array('Column 1', 'Column 2', 'Column 3')); // 写入数据行 while ($row = $result->fetch_assoc()) { fputcsv($handle, array($row['column1'], $row['column2'], $row['column3'])); } // 关闭文件句柄 fclose($handle); echo "数据导出成功!"; } else { echo "数据库中无数据!"; } // 关闭数据库连接 $conn->close(); ?>
In the above code, we also introduce the database configuration file and establish a database connection. Then, we use the SELECT statement to query the data in the database, and use the fputcsv function to write the query results to a CSV file. Finally, close the file handle and database connection.
Summary:
This article introduces how to use PHP to implement data import and export functions, and gives specific code examples. By using PHP's file operation and database operation related functions, we can easily implement data import and export functions. I hope this article can help you develop data import and export functions in actual projects.
The above is the detailed content of PHP development skills: How to implement data import and export functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
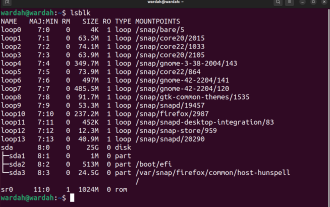
DDREASE is a tool for recovering data from file or block devices such as hard drives, SSDs, RAM disks, CDs, DVDs and USB storage devices. It copies data from one block device to another, leaving corrupted data blocks behind and moving only good data blocks. ddreasue is a powerful recovery tool that is fully automated as it does not require any interference during recovery operations. Additionally, thanks to the ddasue map file, it can be stopped and resumed at any time. Other key features of DDREASE are as follows: It does not overwrite recovered data but fills the gaps in case of iterative recovery. However, it can be truncated if the tool is instructed to do so explicitly. Recover data from multiple files or blocks to a single
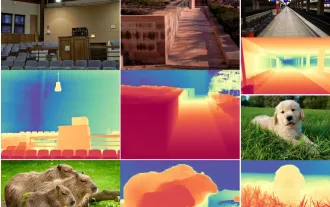
0.What does this article do? We propose DepthFM: a versatile and fast state-of-the-art generative monocular depth estimation model. In addition to traditional depth estimation tasks, DepthFM also demonstrates state-of-the-art capabilities in downstream tasks such as depth inpainting. DepthFM is efficient and can synthesize depth maps within a few inference steps. Let’s read about this work together ~ 1. Paper information title: DepthFM: FastMonocularDepthEstimationwithFlowMatching Author: MingGui, JohannesS.Fischer, UlrichPrestel, PingchuanMa, Dmytr
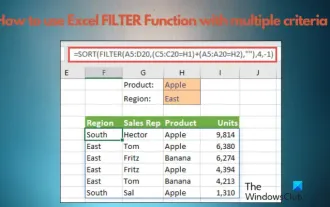
If you need to know how to use filtering with multiple criteria in Excel, the following tutorial will guide you through the steps to ensure you can filter and sort your data effectively. Excel's filtering function is very powerful and can help you extract the information you need from large amounts of data. This function can filter data according to the conditions you set and display only the parts that meet the conditions, making data management more efficient. By using the filter function, you can quickly find target data, saving time in finding and organizing data. This function can not only be applied to simple data lists, but can also be filtered based on multiple conditions to help you locate the information you need more accurately. Overall, Excel’s filtering function is a very practical
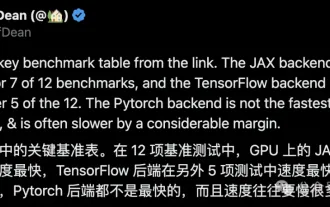
The performance of JAX, promoted by Google, has surpassed that of Pytorch and TensorFlow in recent benchmark tests, ranking first in 7 indicators. And the test was not done on the TPU with the best JAX performance. Although among developers, Pytorch is still more popular than Tensorflow. But in the future, perhaps more large models will be trained and run based on the JAX platform. Models Recently, the Keras team benchmarked three backends (TensorFlow, JAX, PyTorch) with the native PyTorch implementation and Keras2 with TensorFlow. First, they select a set of mainstream
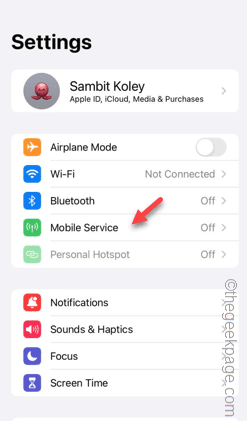
Facing lag, slow mobile data connection on iPhone? Typically, the strength of cellular internet on your phone depends on several factors such as region, cellular network type, roaming type, etc. There are some things you can do to get a faster, more reliable cellular Internet connection. Fix 1 – Force Restart iPhone Sometimes, force restarting your device just resets a lot of things, including the cellular connection. Step 1 – Just press the volume up key once and release. Next, press the Volume Down key and release it again. Step 2 – The next part of the process is to hold the button on the right side. Let the iPhone finish restarting. Enable cellular data and check network speed. Check again Fix 2 – Change data mode While 5G offers better network speeds, it works better when the signal is weaker
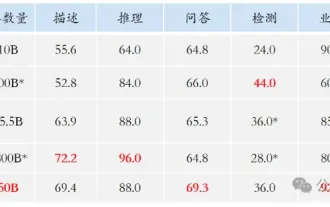
I cry to death. The world is madly building big models. The data on the Internet is not enough. It is not enough at all. The training model looks like "The Hunger Games", and AI researchers around the world are worrying about how to feed these data voracious eaters. This problem is particularly prominent in multi-modal tasks. At a time when nothing could be done, a start-up team from the Department of Renmin University of China used its own new model to become the first in China to make "model-generated data feed itself" a reality. Moreover, it is a two-pronged approach on the understanding side and the generation side. Both sides can generate high-quality, multi-modal new data and provide data feedback to the model itself. What is a model? Awaker 1.0, a large multi-modal model that just appeared on the Zhongguancun Forum. Who is the team? Sophon engine. Founded by Gao Yizhao, a doctoral student at Renmin University’s Hillhouse School of Artificial Intelligence.
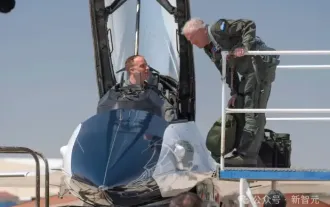
Recently, the military circle has been overwhelmed by the news: US military fighter jets can now complete fully automatic air combat using AI. Yes, just recently, the US military’s AI fighter jet was made public for the first time and the mystery was unveiled. The full name of this fighter is the Variable Stability Simulator Test Aircraft (VISTA). It was personally flown by the Secretary of the US Air Force to simulate a one-on-one air battle. On May 2, U.S. Air Force Secretary Frank Kendall took off in an X-62AVISTA at Edwards Air Force Base. Note that during the one-hour flight, all flight actions were completed autonomously by AI! Kendall said - "For the past few decades, we have been thinking about the unlimited potential of autonomous air-to-air combat, but it has always seemed out of reach." However now,
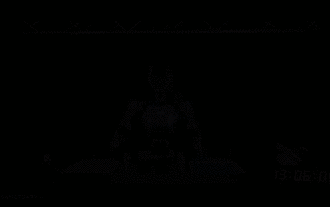
This week, FigureAI, a robotics company invested by OpenAI, Microsoft, Bezos, and Nvidia, announced that it has received nearly $700 million in financing and plans to develop a humanoid robot that can walk independently within the next year. And Tesla’s Optimus Prime has repeatedly received good news. No one doubts that this year will be the year when humanoid robots explode. SanctuaryAI, a Canadian-based robotics company, recently released a new humanoid robot, Phoenix. Officials claim that it can complete many tasks autonomously at the same speed as humans. Pheonix, the world's first robot that can autonomously complete tasks at human speeds, can gently grab, move and elegantly place each object to its left and right sides. It can autonomously identify objects
