Java development: How to use JPA for database query and persistence
Java development: How to use JPA for database query and persistence
JPA (Java Persistence API) is a set of tools defined in the Java EE specification for managing database persistence. API. It provides an object-oriented way to perform database operations, allowing developers to more easily perform database queries and data persistence. This article will introduce how to use JPA for database query and persistence, and provide detailed code examples.
Use JPA for database query
Before using JPA for database query, we need to define the entity class and the mapping relationship between the entity class and the database table. The following is the code of an example entity class:
@Entity @Table(name = "t_student") public class Student { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @Column(name = "age") private Integer age; // getters and setters }
In the above code, the JPA annotation @Entity is used to indicate that the class is an entity class, and @Table specifies the corresponding database table name, @Id and @ GeneratedValue is used to specify the primary key and automatic generation strategy, and @Column is used to specify the mapped database column.
Next, we can use the EntityManager object to perform database queries. The following is a sample code for querying student information based on ID:
public Student findStudentById(Long id) { EntityManager entityManager = entityManagerFactory.createEntityManager(); return entityManager.find(Student.class, id); }
In the above code, the query operation is performed by calling the find method of the entityManager object and passing in the entity class and ID. The returned result is the queried entity object.
In addition to querying based on id, JPA also provides rich query syntax and methods, which can be queried based on conditions. The following is a sample code for querying student information based on name:
public List<Student> findStudentsByName(String name) { EntityManager entityManager = entityManagerFactory.createEntityManager(); CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder(); CriteriaQuery<Student> criteriaQuery = criteriaBuilder.createQuery(Student.class); Root<Student> root = criteriaQuery.from(Student.class); criteriaQuery.select(root).where(criteriaBuilder.equal(root.get("name"), name)); TypedQuery<Student> query = entityManager.createQuery(criteriaQuery); return query.getResultList(); }
In the above code, by using CriteriaQuery, CriteriaBuilder and other classes, we can construct complex query statements. In the example, we query the student information that meets the conditions through the passed in name parameter.
Use JPA for data persistence
Before using JPA for data persistence, you also need to define the entity class and the mapping relationship between the entity class and the database table. The following is an example of code that persists student information to the database:
public void saveStudent(Student student) { EntityManager entityManager = entityManagerFactory.createEntityManager(); EntityTransaction transaction = entityManager.getTransaction(); try { transaction.begin(); entityManager.persist(student); transaction.commit(); } catch (Exception e) { if (transaction != null) { transaction.rollback(); } e.printStackTrace(); } finally { entityManager.close(); } }
In the above code, we first obtain the transaction object through the getTransaction method of the entityManager object. The entity object is then persisted to the database by calling the persist method. Finally, the transaction commit and rollback operations are performed in the try-catch-finally block.
In addition to insert operations, JPA also provides update and delete operations. The following is an example code for updating student information:
public void updateStudent(Student student) { EntityManager entityManager = entityManagerFactory.createEntityManager(); EntityTransaction transaction = entityManager.getTransaction(); try { transaction.begin(); entityManager.merge(student); transaction.commit(); } catch (Exception e) { if (transaction != null) { transaction.rollback(); } e.printStackTrace(); } finally { entityManager.close(); } }
In the above code, we use the merge method to update student information.
Summary
This article introduces how to use JPA for database query and persistence, and provides detailed code examples. By using JPA, developers can perform database operations more easily and improve development efficiency. Of course, JPA has many other functions and features, and I hope readers can learn more about them and use them flexibly.
The above is the detailed content of Java development: How to use JPA for database query and persistence. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


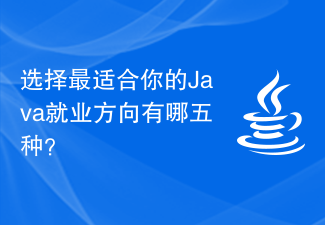
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
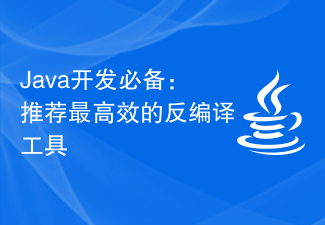
Essential for Java developers: Recommend the best decompilation tool, specific code examples are required Introduction: During the Java development process, we often encounter situations where we need to decompile existing Java classes. Decompilation can help us understand and learn other people's code, or make repairs and optimizations. This article will recommend several of the best Java decompilation tools and provide some specific code examples to help readers better learn and use these tools. 1. JD-GUIJD-GUI is a very popular open source
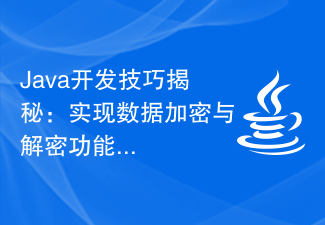
Java development skills revealed: Implementing data encryption and decryption functions In the current information age, data security has become a very important issue. In order to protect the security of sensitive data, many applications use encryption algorithms to encrypt the data. As a very popular programming language, Java also provides a rich library of encryption technologies and tools. This article will reveal some techniques for implementing data encryption and decryption functions in Java development to help developers better protect data security. 1. Selection of data encryption algorithm Java supports many
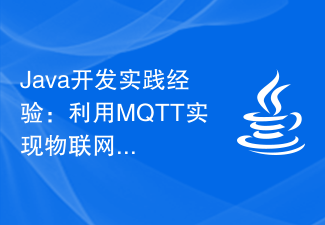
With the development of IoT technology, more and more devices are able to connect to the Internet and communicate and interact through the Internet. In the development of IoT applications, the Message Queuing Telemetry Transport Protocol (MQTT) is widely used as a lightweight communication protocol. This article will introduce how to use Java development practical experience to implement IoT functions through MQTT. 1. What is MQT? QTT is a message transmission protocol based on the publish/subscribe model. It has a simple design and low overhead, and is suitable for application scenarios that quickly transmit small amounts of data.
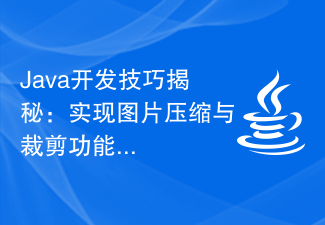
Java is a programming language widely used in the field of software development. Its rich libraries and powerful functions can be used to develop various applications. Image compression and cropping are common requirements in web and mobile application development. In this article, we will reveal some Java development techniques to help developers implement image compression and cropping functions. First, let's discuss the implementation of image compression. In web applications, pictures often need to be transmitted over the network. If the image is too large, it will take longer to load and use more bandwidth. therefore, we
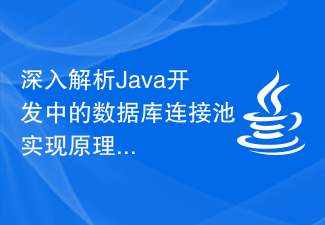
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
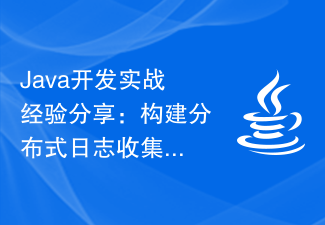
Sharing practical experience in Java development: Building a distributed log collection function Introduction: With the rapid development of the Internet and the emergence of large-scale data, the application of distributed systems is becoming more and more widespread. In distributed systems, log collection and analysis are very important. This article will share the experience of building distributed log collection function in Java development, hoping to be helpful to readers. 1. Background introduction In a distributed system, each node generates a large amount of log information. These log information are useful for system performance monitoring, troubleshooting and data analysis.

As a very popular programming language, Java has always been favored by everyone. When I first started learning Java development, I once encountered a problem-how to build a message subscription system. In this article, I will share my experience in building a message subscription system from scratch, hoping to be helpful to other Java beginners. Step 1: Choose a suitable message queue To build a message subscription system, you first need to choose a suitable message queue. The more popular message queues currently on the market include ActiveMQ,
