Java development: How to refactor code and apply design patterns
Java development: How to perform code refactoring and application of design patterns
In software development, code refactoring and application of design patterns are important to improve code quality and maintainability important means of sex. Code refactoring refers to improving the structure, design, and performance of existing code, while design patterns are proven templates for solving problems. This article will introduce how to refactor code and apply design patterns, and give specific code examples.
1. Code Refactoring
- Extract Method
The extraction method is to extract a repeated piece of code into an independent method. Used to increase code readability and maintainability. For example, the following code has repeated logic in multiple places:
public void printUserInfo(User user) { System.out.println("Name: " + user.getName()); System.out.println("Age: " + user.getAge()); System.out.println("Email: " + user.getEmail()); } public void printOrderInfo(Order order) { System.out.println("Order ID: " + order.getId()); System.out.println("Order Date: " + order.getDate()); System.out.println("Order Total: " + order.getTotal()); }
We can extract this repeated code into an independent method:
public void printInfo(String label, Printable printable) { System.out.println(label + printable.getInfo()); } public interface Printable { String getInfo(); } public class User implements Printable { // ... public String getInfo() { return "Name: " + name + " Age: " + age + " Email: " + email; } } public class Order implements Printable { // ... public String getInfo() { return "Order ID: " + id + " Order Date: " + date + " Order Total: " + total; } }
By extracting the method, we can Reduce code duplication and better encapsulate and reuse logic.
- Merge duplicate conditions (Consolidate Conditional Expression)
When we find that there is repeated logic in multiple conditional expressions, we can merge them into a simpler one , more readable expressions. For example, the following code has repeated judgment logic:
public double calculateDiscount(double price, int quantity) { double discount = 0.0; if (price > 100 && quantity > 5) { discount = price * 0.1; } else if (price > 200 && quantity > 10) { discount = price * 0.2; } else if (price > 300 && quantity > 15) { discount = price * 0.3; } return discount; }
We can merge this repeated condition into a simpler expression:
public double calculateDiscount(double price, int quantity) { double discount = 0.0; if (price > 300 && quantity > 15) { discount = price * 0.3; } else if (price > 200 && quantity > 10) { discount = price * 0.2; } else if (price > 100 && quantity > 5) { discount = price * 0.1; } return discount; }
By merging the repeated conditions, we can Improve code readability and maintainability.
2. Design pattern application
- Singleton mode
The singleton mode is a method that ensures that a class has only one instance and provides Design pattern for global access points. In actual development, the singleton mode is often used to manage shared resources, configuration information, etc. The following is a simple example of the singleton pattern:
public class Singleton { private static Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } }
By using the double-checked locking mechanism, we can ensure that a unique instance is created in a multi-threaded environment.
- Factory Pattern (Factory)
Factory pattern is a design pattern that creates different objects based on different parameters. In actual development, the factory pattern is often used to hide the creation logic of objects and provide a consistent interface to create objects. The following is a simple factory pattern example:
public interface Shape { void draw(); } public class Circle implements Shape { public void draw() { System.out.println("Drawing a circle."); } } public class Rectangle implements Shape { public void draw() { System.out.println("Drawing a rectangle."); } } public class ShapeFactory { public static Shape createShape(String type) { if (type.equals("circle")) { return new Circle(); } else if (type.equals("rectangle")) { return new Rectangle(); } return null; } }
By using the factory pattern, we can decouple the creation and use of objects, increasing the flexibility and maintainability of the code.
Summary:
Code refactoring and the application of design patterns are important means to improve code quality and maintainability. By properly refactoring code and applying design patterns, we can reduce code duplication and improve code readability and maintainability. In actual development, we also need to choose appropriate refactoring techniques and design patterns to optimize the code based on the actual situation and design requirements of the project.
The above is the detailed content of Java development: How to refactor code and apply design patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
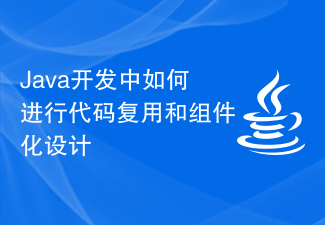
Overview of how to carry out code reuse and componentized design in Java development: In daily Java development, code reuse and componentized design are very important concepts. By rationally utilizing code reuse and component design methods, we can improve the maintainability, scalability and reusability of code, reduce the occurrence of redundant code, and improve development efficiency. 1. Methods of code reuse: 1.1 Inheritance: Inheritance is a commonly used method of code reuse. Code reuse can be achieved by inheriting the properties and methods of the parent class. For example, we have a
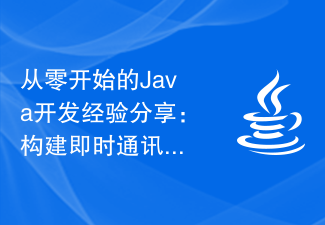
The Java language has become the mainstay of enterprise-level applications and back-end development. For beginners, it is recommended to start with a relatively simple development project, such as building an instant messaging system. Through this project, you can learn core Java concepts and skills, such as object-oriented programming, network programming, multi-threaded programming, and database operations. The following is an experience sharing of building a Java instant messaging system from scratch. Designing the Database Structure First requires designing the database structure, which is the foundation of any application. For instant messaging systems, you need
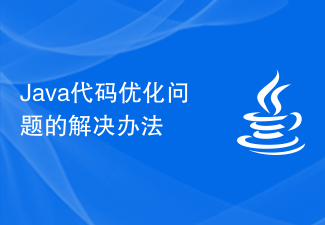
How to solve code optimization problems encountered in Java During the development of Java applications, we often encounter the problem of low code efficiency. These problems may cause the program to run slowly, consume large amounts of resources, or even cause the program to crash. In response to these problems, we need to optimize the code to improve the performance and efficiency of the program. This article will explore some common Java code optimization problems and provide some solution ideas. 1. Loop optimization Loop is a structure we often use, but if the loop is too complex or there are redundant operations
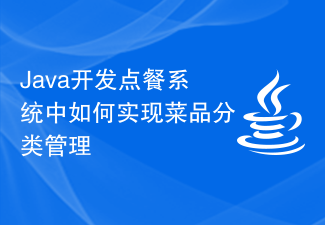
How to implement dish classification management in Java development of ordering systems. In the catering industry, the development of ordering systems has attracted more and more attention, which can greatly improve the efficiency and service quality of restaurants. Among them, dish classification management is an important functional module in the ordering system. This article will introduce how to implement dish classification management in a Java ordering system. 1. Requirements analysis In the dish category management module, we need to implement the following functions: Add dish category: You can add new dish categories to the system, including category names, category icons, etc. Edit dish
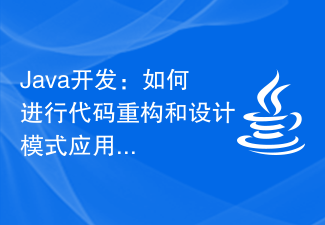
Java development: How to perform code refactoring and application of design patterns. In software development, code refactoring and the application of design patterns are important means to improve code quality and maintainability. Code refactoring refers to improving the structure, design, and performance of existing code, while design patterns are proven templates for solving problems. This article will introduce how to refactor code and apply design patterns, and give specific code examples. 1. Code Refactoring Extraction Method (ExtractMethod) The extraction method is to extract a repeated piece of code
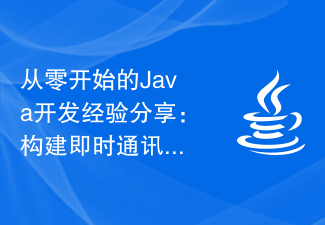
The Java language has become the mainstay of enterprise-level applications and back-end development. For beginners, it is recommended to start with a relatively simple development project, such as building an instant messaging system. Through this project, you can learn core Java concepts and skills, such as object-oriented programming, network programming, multi-threaded programming, and database operations. The following is an experience sharing of building a Java instant messaging system from scratch. Designing the Database Structure First requires designing the database structure, which is the foundation of any application. For instant messaging systems, you need
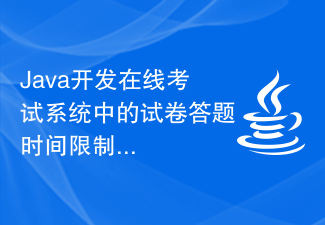
The time limit for answering questions in the Java development online examination system requires specific code examples. When developing an online examination system, limiting the time for answering questions is one of the very important functions. This ensures that students complete their answers within the allotted time and submit their exam papers in a timely manner. This article will introduce how to implement the function of time limit for test paper answering through Java code. First, we need to define a timer to record the time when students start answering questions. You can use System.currentTime in Java
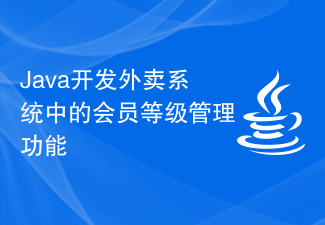
Member level management function in Java development takeout system With the rapid development of the mobile Internet, the takeout industry is gradually rising in the Chinese market. As an important part of people's lives, the increasingly perfect and convenient takeout system has become the goal of pursuing user experience. Among them, the membership level management function is an important part of the takeout system, which can provide users with more discounts and services, and can also effectively promote users' consumption willingness and loyalty. As a mature and powerful programming language, Java plays an important role in the development of takeout systems.
