How to use Redis and Kotlin to develop asynchronous task queue functions
How to use Redis and Kotlin to develop asynchronous task queue function
Introduction:
With the development of the Internet, the processing of asynchronous tasks has become more and more important. During the development process, we often encounter some time-consuming tasks, such as sending emails, processing big data, etc. In order to improve the performance and scalability of the system, we can use asynchronous task queues to process these tasks. This article will introduce how to use Redis and Kotlin to develop a simple asynchronous task queue, and provide specific code examples.
1. What is an asynchronous task queue?
Asynchronous task queue is a mechanism that puts long-term tasks into a queue for asynchronous execution. By placing the task in the queue, the system can return it to the user immediately without waiting for the task's execution to complete. Asynchronous task queues usually adopt a producer-consumer model, that is, one or more producers add tasks to the queue, and one or more consumers take tasks from the queue and execute them.
2. Advantages of Redis
Redis is a high-performance key-value storage system that supports a variety of data structures, such as strings, lists, sets, hash tables, etc. Redis's high performance and flexibility make it an ideal choice for developing asynchronous task queues. In Redis, we can use the list data structure as a task queue and the publish-subscribe (Pub/Sub) mode to achieve task distribution.
3. Steps to implement asynchronous task queue using Redis and Kotlin
-
Add Redis dependency
First, add the Redis client in the build.gradle file of the Kotlin project Side dependencies:dependencies { implementation 'redis.clients:jedis:3.7.0' }
Copy after login Create a producer
Create a Producer class responsible for adding tasks to the Redis task queue:import redis.clients.jedis.Jedis import redis.clients.jedis.JedisPool class Producer { private val redisHost = "localhost" // Redis的主机地址 private val redisPort = 6379 // Redis的端口号 private val jedisPool = JedisPool(redisHost, redisPort) fun addTask(task: String) { val jedis = jedisPool.resource jedis.rpush("task_queue", task) // 将任务添加到任务队列中 jedis.close() } }
Copy after loginCreate Consumer
Create a Consumer class, responsible for taking out tasks from the Redis task queue and executing:import redis.clients.jedis.Jedis import redis.clients.jedis.JedisPool class Consumer { private val redisHost = "localhost" // Redis的主机地址 private val redisPort = 6379 // Redis的端口号 private val jedisPool = JedisPool(redisHost, redisPort) fun start() { val jedis = jedisPool.resource while (true) { val task = jedis.blpop(0, "task_queue")[1] // 从任务队列中取出任务 executeTask(task) // 执行任务 } jedis.close() } private fun executeTask(task: String) { // 执行任务的具体代码逻辑 println("执行任务:$task") } }
Copy after loginTest
Create a Producer object in the main function, Add the task to the task queue. Then create a Consumer object and start the consumer's start function. This completes the implementation of a simple asynchronous task queue:fun main() { val producer = Producer() producer.addTask("task1") producer.addTask("task2") val consumer = Consumer() consumer.start() }
Copy after login
IV. Summary
By using Redis and Kotlin, we can easily develop a simple asynchronous task queue . Redis provides high-performance key-value storage and publish-subscribe functionality, while Kotlin provides a concise and elegant way of writing code. By putting time-consuming tasks into the task queue for asynchronous execution, we can improve the performance and scalability of the system and improve the user experience.
The above are the specific steps and code examples for using Redis and Kotlin to develop asynchronous task queue functions. I hope this article is helpful to everyone, thank you for reading!
The above is the detailed content of How to use Redis and Kotlin to develop asynchronous task queue functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
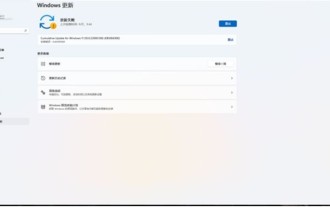
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
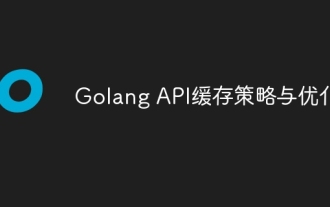
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
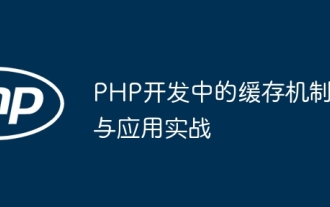
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.

The difference between Java and Kotlin functions: Syntax: Java functions need to specify parameter types and names, while Kotlin can omit the type and use lambda expressions; Parameters: Kotlin can omit parameter types using more concise syntax; Return value: Kotlin can omit the return value Type, the default is Unit; extension function: Kotlin can add new functions to existing classes, while Java needs to implement similar functions through inheritance; instance method call: Kotlin can omit the object name and use a more concise syntax.
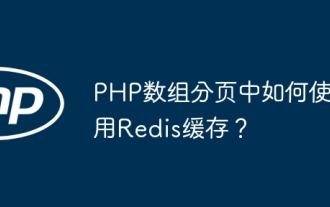
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.
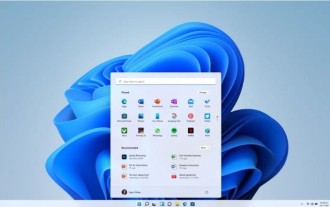
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.

Yes, Navicat can connect to Redis, which allows users to manage keys, view values, execute commands, monitor activity, and diagnose problems. To connect to Redis, select the "Redis" connection type in Navicat and enter the server details.
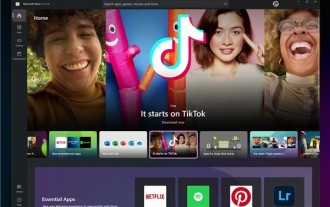
1. First, double-click the [This PC] icon on the desktop to open it. 2. Then double-click the left mouse button to enter [C drive]. System files will generally be automatically stored in C drive. 3. Then find the [windows] folder in the C drive and double-click to enter. 4. After entering the [windows] folder, find the [SoftwareDistribution] folder. 5. After entering, find the [download] folder, which contains all win11 download and update files. 6. If we want to delete these files, just delete them directly in this folder.
