


How to write custom stored procedures, triggers and functions in MySQL using Python
How to write custom stored procedures, triggers and functions in MySQL using Python
The database's stored procedures, triggers and functions are a powerful tool. It can help us implement some complex operations and logic in the database. In MySQL, we can use Python to write custom stored procedures, triggers and functions. This article explains how to use Python to accomplish these tasks in MySQL and provides specific code examples.
1. Custom stored procedures
A stored procedure is a collection of database operations that can be called by a name, and can pass parameters and return results. The following is an example of a stored procedure written in Python:
import mysql.connector def create_procedure(): conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() sql = """ CREATE PROCEDURE GetProduct(IN pro_id INT) BEGIN SELECT * FROM product WHERE id = pro_id; END""" cursor.execute(sql) conn.commit() print("存储过程创建成功!") cursor.close() conn.close() create_procedure()
The above code uses the MySQL Connector/Python library to connect to the database and uses the CREATE PROCEDURE statement to create a stored procedure named GetProduct, which stores The process accepts an integer parameter pro_id, then queries the data in the product table based on the id, and returns the result.
2. Custom triggers
A trigger is a special object in a database table that automatically performs a series of operations when a specific event occurs. Here is an example of a trigger written in Python:
import mysql.connector def create_trigger(): conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() sql = """ CREATE TRIGGER update_product_count AFTER INSERT ON order_item FOR EACH ROW BEGIN UPDATE product SET count = count - NEW.quantity WHERE id = NEW.product_id; END""" cursor.execute(sql) conn.commit() print("触发器创建成功!") cursor.close() conn.close() create_trigger()
The above code uses the MySQL Connector/Python library to connect to the database and uses the CREATE TRIGGER statement to create a trigger named update_product_count, which triggers The program is automatically executed after inserting a new record into the order_item table, and the inventory is automatically updated by updating the quantity of the corresponding product in the product table.
3. Custom function
The function is a piece of reusable code that receives a certain input, processes the input and returns an output. Here is an example of a function written in Python:
import mysql.connector def create_function(): conn = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="yourdatabase" ) cursor = conn.cursor() sql = """ CREATE FUNCTION GetProductPrice(pro_id INT) RETURNS DECIMAL(10,2) BEGIN DECLARE price DECIMAL(10,2); SELECT price INTO price FROM product WHERE id = pro_id; RETURN price; END""" cursor.execute(sql) conn.commit() print("函数创建成功!") cursor.close() conn.close() create_function()
The above code uses the MySQL Connector/Python library to connect to the database and uses the CREATE FUNCTION statement to create a function called GetProductPrice that accepts a Integer parameter pro_id, then query the price of the corresponding product in the product table based on the id, and return the price.
Summary:
By using Python to write custom stored procedures, triggers and functions, we can implement more flexible and complex operations and logic in the MySQL database. Using the Python programming language allows us to complete these tasks more quickly and efficiently. I hope this article will help you write custom stored procedures, triggers and functions in MySQL using Python.
The above is the detailed content of How to write custom stored procedures, triggers and functions in MySQL using Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


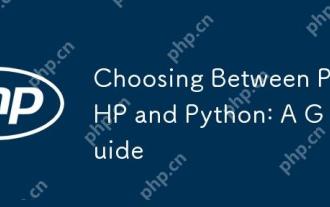
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
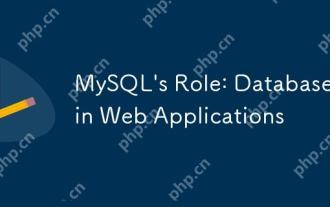
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
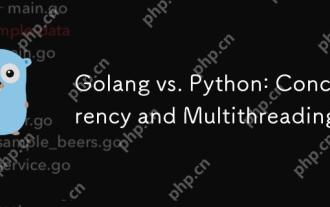
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
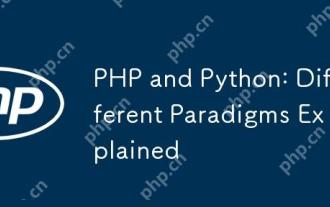
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
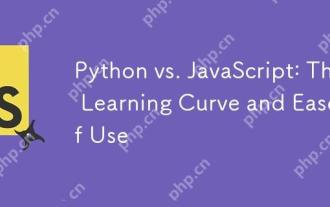
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
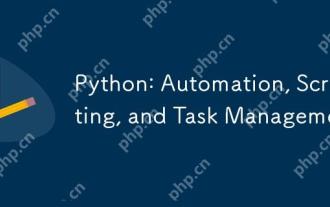
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
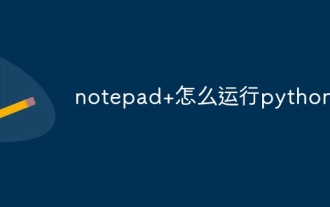
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
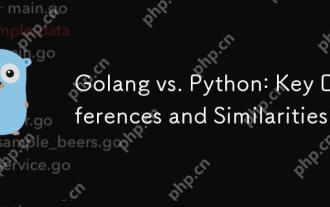
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
