


How to use PHP to develop simple online code debugging tools and error logging functions
How to use PHP to develop simple online code debugging tools and error logging functions
Introduction:
In the process of developing and debugging code, we often Encountered various errors and bugs. In order to locate and solve these problems more conveniently, we can develop a simple online code debugging tool and add an error logging function to facilitate subsequent troubleshooting. This article will introduce how to develop this tool using PHP language and provide specific code examples.
1. Create a simple Web application
First, we need to create a simple Web application. We can use PHP's built-in web server to create an index.php file, which will serve as the entrance to our web application.
In the index.php file, we can add some basic HTML code and CSS styles to make our debugging tool interface more friendly and beautiful. At the same time, we need to add a text box for entering the PHP code to be debugged, and add a button. After clicking the button, the code will be sent to the server for execution and debugging.
The following is the sample code of the index.php file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
2. Create a PHP script for execution and debugging code
Next, we need to create a PHP script for execution and debugging Code PHP script. We name this script debug.php.
In the debug.php file, we need to obtain the code POST from the index.php page, and then use the eval() function to execute this code and capture errors during the execution. At the same time, we also need to record the error information into the log file so that we can locate and solve the problem later.
The following is the sample code of the debug.php file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
3. Add the error logging function
We have added the error logging function to the debug.php file. When an error occurs during code execution, the error information is logged to a log file named error.log. We can call the error_get_last() function anywhere in the script to get the latest error information.
In order to make the recorded error information more detailed and useful, we can record the time when the error occurred, the error message, the file and line number where the error is located, and other information. At the same time, we can also use the file_put_contents() function to write error information to the log file.
4. Run the debugging tool
To run the debugging tool, we need to switch to the directory where index.php is located on the command line, and execute the following command to start the PHP built-in web server:
1 |
|
Then, we can access http://localhost:8000 in the browser to open our debugging tool interface.
In the interface, we can enter the PHP code to be debugged and click the "Debug" button to send the code to the server for execution and debugging. At the same time, the error information will be recorded in the error.log file for our subsequent viewing and analysis.
Summary:
By using PHP to develop a simple online code debugging tool and error logging function, we can more easily locate and solve errors and problems in the code. In actual development, we can expand and optimize debugging tools according to needs to improve development efficiency and code quality.
The above is the detailed content of How to use PHP to develop simple online code debugging tools and error logging functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


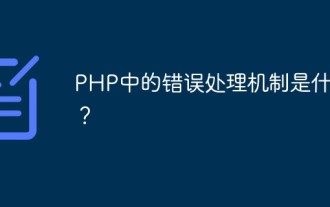
PHP is a popular and powerful server-side programming language that can be used to develop various web applications. Just like other programming languages, PHP is prone to errors and exceptions. These errors and exceptions may be caused by various reasons, such as program errors, server errors, user input errors, etc. In order to ensure the running stability and reliability of the program, PHP provides a complete set of error handling mechanisms. The basic idea of PHP error handling mechanism is: when an error occurs, the program will stop execution and output an error message. we can
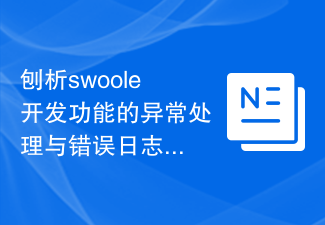
Analyzing the exception handling and error logging mechanism of swoole development function Introduction: Swoole is a high-performance PHP extension that provides powerful asynchronous and concurrent processing capabilities and is widely used in high-performance web development, microservices, game development, etc. field. In development, exception handling and error log recording are very important, which can help us find and solve problems in time and improve the stability and maintainability of the application. This article will delve into the mechanism of exception handling and error logging in swoole development.
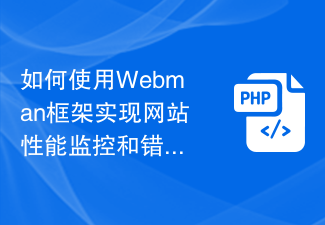
How to use the Webman framework to implement website performance monitoring and error logging? Webman is a powerful and easy-to-use PHP framework that provides a series of powerful tools and components to help us build high-performance and reliable websites. Among them, website performance monitoring and error logging are very important functions, which can help us find and solve problems in time and improve user experience. Below we will introduce how to use the Webman framework to implement these two functions. First, we need to create
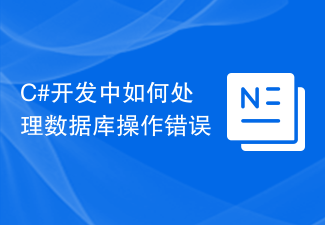
How to handle database operation errors in C# development In C# development, database operation is a common task. However, when performing database operations, you may encounter various errors, such as connection failure, query failure, update failure, etc. In order to ensure the robustness and stability of the program, we need to take corresponding strategies and measures when dealing with database operation errors. Here are some suggestions and specific code examples for handling errors in database operations: Exception Handling In C#, you can use the exception handling mechanism to catch and handle errors in database operations. exist
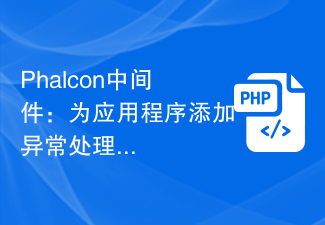
Phalcon middleware: Adding exception handling and error logging functions to applications In recent years, with the rapid development of web applications, how to ensure the stability and reliability of the program has become a focus of developers. Common problems such as how to handle exceptions thrown by applications, recording error messages, and managing logs all require us to have a good solution. The middleware mechanism of the Phalcon framework provides us with an effective way to add exception handling and error logging functionality. This article will introduce
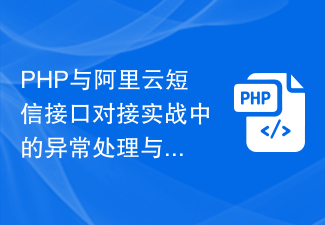
Exception handling and error logging methods in actual docking of PHP and Alibaba Cloud SMS interface Introduction: With the continuous development of the Internet, SMS services are increasingly used in various scenarios. As the leading company in domestic SMS services, Alibaba Cloud SMS Service provides a simple and easy-to-use SMS interface and is favored by many developers. In practical applications, docking with the Alibaba Cloud SMS interface is often an issue that must be considered. This article will introduce the practical experience of docking PHP and Alibaba Cloud SMS interface, and focus on how to handle exceptions and record errors.
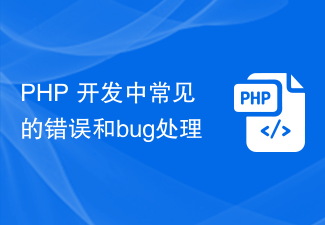
PHP is a scripting language widely used in server-side development. Its simplicity and ease of learning make it the first choice for many developers. However, like any programming language, PHP development faces some common mistakes and bugs. This article will introduce some common PHP development errors and corresponding solutions. 1. Grammar Errors Grammar errors are one of the most common errors. Since PHP is a scripting language, syntax errors are especially likely to occur. For example, missing semicolons, brackets not matching correctly, etc. The way to solve this kind of error is to carefully check

Using debugging tools in PHP programming can help developers better understand and debug code, and improve development efficiency and code quality. This article will introduce how to use common PHP debugging tools. XdebugXdebug is one of the most popular tools in PHP debugging. It can help developers debug and perform performance analysis of PHP code. Its main functions include: Debugging: You can set breakpoints in PHP code and conduct line-by-line debugging. Variable tracking: You can track variable assignments and usage. Stack trace: you can view the code execution
