


How to use PHP to develop a simple online reservation function
How to use PHP to develop a simple online appointment function
With the popularity and development of the Internet, more and more companies and institutions need to provide online appointment functions for convenience Users can make reservations anytime and anywhere. PHP is a popular server-side programming language with powerful development capabilities and rich development resources. It is very suitable for developing online reservation functions. This article will introduce how to use PHP to develop a simple online reservation function and provide specific code examples.
1. Set up a development environment
First, we need to set up a PHP development environment. You can choose to use integrated environments such as XAMPP and WAMP, or you can build your own LAMP (Linux Apache MySQL PHP) or WAMP (Windows Apache MySQL PHP) environment. Make sure PHP can run properly.
2. Create a database
The online reservation function usually requires a database to store reservation information. We can use the MySQL database to create the reservation table. The following is an example of a simple appointment form structure:
CREATE TABLE appointments ( id INT(11) PRIMARY KEY AUTO_INCREMENT, name VARCHAR(50) NOT NULL, email VARCHAR(50) NOT NULL, phone VARCHAR(20) NOT NULL, appointment_date DATE NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
3. Create an appointment form
Create an appointment form on the web page to allow users to fill in the appointment information. You can use HTML and CSS to design the form interface, and PHP is responsible for receiving and processing the data submitted by the form. The following is a simple appointment form example:
<form action="submit.php" method="post"> <input type="text" name="name" placeholder="姓名" required> <input type="email" name="email" placeholder="邮箱" required> <input type="tel" name="phone" placeholder="电话" required> <input type="date" name="appointment_date" required> <input type="submit" value="提交"> </form>
4. Processing form submission
When the user clicks the submit button, the PHP code will receive the data submitted by the form and insert it into the appointment table. The following is a sample code for processing form submission:
<?php // 连接到数据库 $conn = new mysqli('localhost', 'username', 'password', 'database'); // 检查数据库连接是否成功 if ($conn->connect_error) { die("数据库连接失败:" . $conn->connect_error); } // 处理表单提交 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $name = $_POST['name']; $email = $_POST['email']; $phone = $_POST['phone']; $appointment_date = $_POST['appointment_date']; // 插入预约信息到数据库 $sql = "INSERT INTO appointments (name, email, phone, appointment_date) VALUES ('$name', '$email', '$phone', '$appointment_date')"; if ($conn->query($sql) === TRUE) { echo "预约成功!"; } else { echo "预约失败:" . $conn->error; } } // 关闭数据库连接 $conn->close(); ?>
5. Display reservation information
You can write PHP code to obtain reservation information from the database and display it on the web page. The following is a sample code that displays reservation information:
<?php // 连接到数据库 $conn = new mysqli('localhost', 'username', 'password', 'database'); // 检查数据库连接是否成功 if ($conn->connect_error) { die("数据库连接失败:" . $conn->connect_error); } // 查询预约信息 $sql = "SELECT * FROM appointments"; $result = $conn->query($sql); // 显示预约信息 if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "姓名:" . $row['name'] . "<br>"; echo "邮箱:" . $row['email'] . "<br>"; echo "电话:" . $row['phone'] . "<br>"; echo "预约日期:" . $row['appointment_date'] . "<br>"; echo "<hr>"; } } else { echo "暂无预约信息"; } // 关闭数据库连接 $conn->close(); ?>
6. Improve functions and optimize code
The above code is just a simple example. In actual development, other functions can be added, such as form verification and verification code. , user login, etc. At the same time, you can also optimize the code, such as using PDO to operate the database, using the PHP framework to simplify the development process, etc.
Summary:
This article introduces how to use PHP to develop a simple online reservation function. By building a development environment, creating a database, creating a reservation form, processing form submission, and displaying reservation information, a simple reservation system can be quickly implemented. At the same time, function expansion and code optimization can also be carried out according to actual needs to improve user experience and development efficiency.
The above is the detailed content of How to use PHP to develop a simple online reservation function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


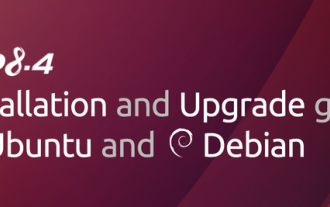
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
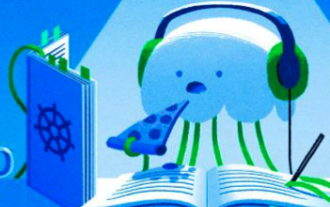
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
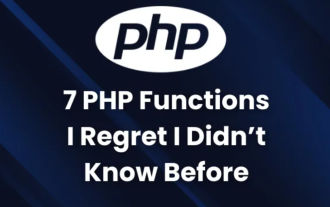
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
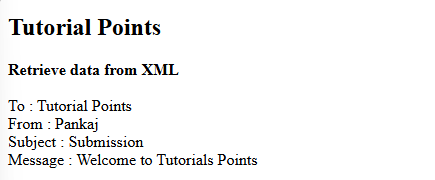
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
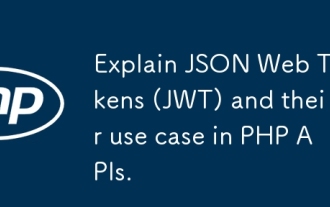
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
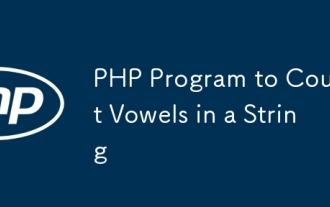
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
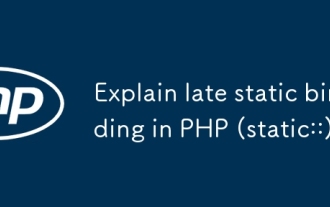
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
