How to write Huffman coding algorithm using C#
How to use C# to write Huffman coding algorithm
Introduction:
The Huffman coding algorithm is a lossless algorithm used for data compression. During data transmission or storage, data is effectively compressed by using shorter codes for more frequent characters and longer codes for less frequent characters. This article will introduce how to use C# to write the Huffman coding algorithm and provide specific code examples.
- Basic principles of Huffman coding algorithm
The core idea of Huffman coding algorithm is to construct a Huffman tree. First, by counting the frequency of character occurrences, each character is treated as a node, and a letter tree is constructed based on the frequency. Then, by combining the two nodes with lower frequency into a new node with the frequency being the sum of the frequencies of the two nodes, and inserting the new node into the alphabet tree. Finally, the process is repeated until only one root node remains, building a complete Huffman tree. Next, each character is encoded according to the Huffman tree, with more frequent characters using shorter encodings and less frequent characters using longer encodings. Data compression can be achieved by converting the encoded character sequence into binary data. -
C#Steps to implement Huffman coding algorithm
Step 1: Count character frequency
Traverse the data to be compressed and count the frequency of each character. You can use a dictionary or array to save the correspondence between characters and frequencies.Step 2: Construct a Huffman tree
Based on the statistical results of character frequency, construct a Huffman tree. Construction can be assisted by a priority queue (such as a priority queue or a heap).Step 3: Generate Huffman code
Recursively traverse the Huffman tree and generate the Huffman code corresponding to each character. A dictionary can be used to store the correspondence between characters and corresponding encodings.Step 4: Compress and decompress
Use the encoding generated in step 3 to compress the original data, and write the encoded binary data into the compressed file. During decompression, the compressed file is read and decoded according to Huffman coding to restore the original data. - C# Code Example
// 步骤1:统计字符频率 Dictionary<char, int> frequencies = new Dictionary<char, int>(); string data = "Hello, World!"; foreach (char c in data) { if (frequencies.ContainsKey(c)) { frequencies[c]++; } else { frequencies[c] = 1; } } // 步骤2:构建霍夫曼树 var pq = new PriorityQueue<HuffmanNode>(); foreach (var entry in frequencies) { pq.Enqueue(new HuffmanNode(entry.Key, entry.Value), entry.Value); } while (pq.Count > 1) { var left = pq.Dequeue(); var right = pq.Dequeue(); pq.Enqueue(new HuffmanNode(left, right), left.Frequency + right.Frequency); } HuffmanNode root = pq.Dequeue(); // 步骤3:生成霍夫曼编码 var codes = new Dictionary<char, string>(); GenerateCodes(root, "", codes); void GenerateCodes(HuffmanNode node, string code, Dictionary<char, string> codes) { if (node.IsLeaf()) { codes[node.Character] = code; } else { GenerateCodes(node.Left, code + '0', codes); GenerateCodes(node.Right, code + '1', codes); } } // 步骤4:压缩和解压缩 string compressedData = Compress(data, codes); string decompressedData = Decompress(compressedData, root); string Compress(string data, Dictionary<char, string> codes) { StringBuilder compressed = new StringBuilder(); foreach (char c in data) { compressed.Append(codes[c]); } return compressed.ToString(); } string Decompress(string compressedData, HuffmanNode root) { StringBuilder decompressed = new StringBuilder(); HuffmanNode current = root; foreach (char c in compressedData) { if (c == '0') { current = current.Left; } else if (c == '1') { current = current.Right; } if (current.IsLeaf()) { decompressed.Append(current.Character); current = root; } } return decompressed.ToString(); }
Conclusion:
This article introduces how to write the Huffman coding algorithm using C# and provides detailed code examples. By using the Huffman coding algorithm, data can be effectively compressed, thereby reducing storage and transmission overhead. Readers can further study and apply the Huffman coding algorithm based on the sample code provided in this article.
The above is the detailed content of How to write Huffman coding algorithm using C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


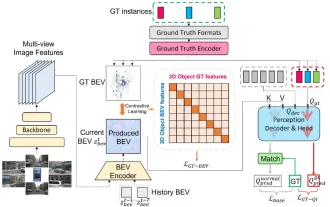
Written above & the author’s personal understanding: At present, in the entire autonomous driving system, the perception module plays a vital role. The autonomous vehicle driving on the road can only obtain accurate perception results through the perception module. The downstream regulation and control module in the autonomous driving system makes timely and correct judgments and behavioral decisions. Currently, cars with autonomous driving functions are usually equipped with a variety of data information sensors including surround-view camera sensors, lidar sensors, and millimeter-wave radar sensors to collect information in different modalities to achieve accurate perception tasks. The BEV perception algorithm based on pure vision is favored by the industry because of its low hardware cost and easy deployment, and its output results can be easily applied to various downstream tasks.
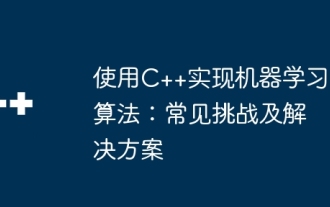
Common challenges faced by machine learning algorithms in C++ include memory management, multi-threading, performance optimization, and maintainability. Solutions include using smart pointers, modern threading libraries, SIMD instructions and third-party libraries, as well as following coding style guidelines and using automation tools. Practical cases show how to use the Eigen library to implement linear regression algorithms, effectively manage memory and use high-performance matrix operations.
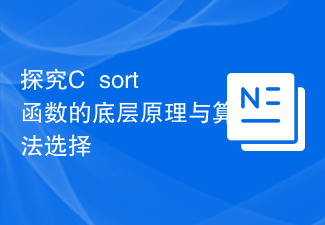
The bottom layer of the C++sort function uses merge sort, its complexity is O(nlogn), and provides different sorting algorithm choices, including quick sort, heap sort and stable sort.
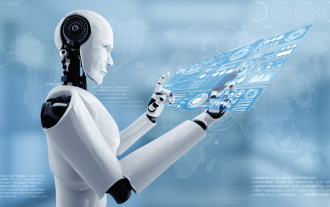
The convergence of artificial intelligence (AI) and law enforcement opens up new possibilities for crime prevention and detection. The predictive capabilities of artificial intelligence are widely used in systems such as CrimeGPT (Crime Prediction Technology) to predict criminal activities. This article explores the potential of artificial intelligence in crime prediction, its current applications, the challenges it faces, and the possible ethical implications of the technology. Artificial Intelligence and Crime Prediction: The Basics CrimeGPT uses machine learning algorithms to analyze large data sets, identifying patterns that can predict where and when crimes are likely to occur. These data sets include historical crime statistics, demographic information, economic indicators, weather patterns, and more. By identifying trends that human analysts might miss, artificial intelligence can empower law enforcement agencies

01 Outlook Summary Currently, it is difficult to achieve an appropriate balance between detection efficiency and detection results. We have developed an enhanced YOLOv5 algorithm for target detection in high-resolution optical remote sensing images, using multi-layer feature pyramids, multi-detection head strategies and hybrid attention modules to improve the effect of the target detection network in optical remote sensing images. According to the SIMD data set, the mAP of the new algorithm is 2.2% better than YOLOv5 and 8.48% better than YOLOX, achieving a better balance between detection results and speed. 02 Background & Motivation With the rapid development of remote sensing technology, high-resolution optical remote sensing images have been used to describe many objects on the earth’s surface, including aircraft, cars, buildings, etc. Object detection in the interpretation of remote sensing images
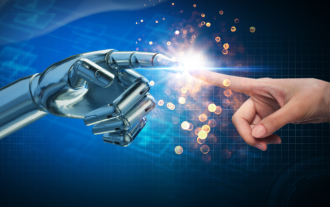
1. The historical development of multi-modal large models. The photo above is the first artificial intelligence workshop held at Dartmouth College in the United States in 1956. This conference is also considered to have kicked off the development of artificial intelligence. Participants Mainly the pioneers of symbolic logic (except for the neurobiologist Peter Milner in the middle of the front row). However, this symbolic logic theory could not be realized for a long time, and even ushered in the first AI winter in the 1980s and 1990s. It was not until the recent implementation of large language models that we discovered that neural networks really carry this logical thinking. The work of neurobiologist Peter Milner inspired the subsequent development of artificial neural networks, and it was for this reason that he was invited to participate in this project.
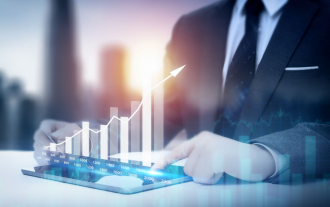
1. Background of the Construction of 58 Portraits Platform First of all, I would like to share with you the background of the construction of the 58 Portrait Platform. 1. The traditional thinking of the traditional profiling platform is no longer enough. Building a user profiling platform relies on data warehouse modeling capabilities to integrate data from multiple business lines to build accurate user portraits; it also requires data mining to understand user behavior, interests and needs, and provide algorithms. side capabilities; finally, it also needs to have data platform capabilities to efficiently store, query and share user profile data and provide profile services. The main difference between a self-built business profiling platform and a middle-office profiling platform is that the self-built profiling platform serves a single business line and can be customized on demand; the mid-office platform serves multiple business lines, has complex modeling, and provides more general capabilities. 2.58 User portraits of the background of Zhongtai portrait construction
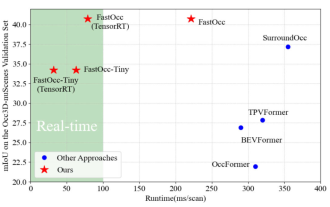
Written above & The author’s personal understanding is that in the autonomous driving system, the perception task is a crucial component of the entire autonomous driving system. The main goal of the perception task is to enable autonomous vehicles to understand and perceive surrounding environmental elements, such as vehicles driving on the road, pedestrians on the roadside, obstacles encountered during driving, traffic signs on the road, etc., thereby helping downstream modules Make correct and reasonable decisions and actions. A vehicle with self-driving capabilities is usually equipped with different types of information collection sensors, such as surround-view camera sensors, lidar sensors, millimeter-wave radar sensors, etc., to ensure that the self-driving vehicle can accurately perceive and understand surrounding environment elements. , enabling autonomous vehicles to make correct decisions during autonomous driving. Head
