


Golang development: using RPC to achieve cross-process communication
Golang development: Using RPC to achieve cross-process communication requires specific code examples
1. Introduction to RPC
RPC (Remote Procedure Call) is a A remote procedure call protocol that allows the client to call functions or methods of the server program located on the remote computer just like calling a local function. RPC can be implemented using different network protocols, such as TCP, HTTP, etc. In distributed systems, RPC is an important communication mechanism, often used for communication across processes or across network nodes.
2. RPC in Golang
Golang provides a built-in RPC library, allowing developers to easily use RPC to achieve cross-process communication. Through Golang's RPC library, we can register server-side functions as methods that can be called by the client, and pass parameters through Golang's data structure. Golang's RPC library supports multiple network protocols, such as TCP, HTTP, etc., and also supports multiple data encoding formats such as JSON and Gob.
3. Code Example
The following is a simple code example that demonstrates how to use RPC to achieve cross-process communication, which includes server and client parts.
Server code:
package main import ( "errors" "net" "net/http" "net/rpc" ) type Arith struct{} type Args struct { A, B int } type Result struct { Value int Err error } func (t *Arith) Multiply(args *Args, result *Result) error { result.Value = args.A * args.B result.Err = nil return nil } func (t *Arith) Divide(args *Args, result *Result) error { if args.B == 0 { result.Value = 0 result.Err = errors.New("divide by zero") } else { result.Value = args.A / args.B result.Err = nil } return nil } func main() { arith := new(Arith) rpc.Register(arith) rpc.HandleHTTP() l, err := net.Listen("tcp", ":1234") if err != nil { panic(err) } http.Serve(l, nil) }
The above code defines a structure named Arith, which contains two methods: Multiply and Divide, which implement the functions of multiplying and dividing two integers respectively. . The Multiply method multiplies two integers, and the Divide method divides two integers. It should be noted that the case where the divisor is 0 is handled in the division.
Next we look at the client code:
package main import ( "fmt" "net/rpc" ) type Args struct { A, B int } type Result struct { Value int Err error } func main() { client, err := rpc.DialHTTP("tcp", "localhost:1234") if err != nil { panic(err) } args := &Args{4, 5} var result Result err = client.Call("Arith.Multiply", args, &result) if err != nil { panic(err) } fmt.Println("Multiply:", result) args = &Args{10, 2} err = client.Call("Arith.Divide", args, &result) if err != nil { panic(err) } fmt.Println("Divide:", result) }
The client code first establishes a connection with the server through the rpc.DialHTTP function, and then calls the client.Call method to call the server method. In this example, Arith's Multiply method is called first, and then Arith's Divide method is called. By printing the results, you can see that the server-side method was successfully called and returned the correct result.
4. Summary
Through the above code examples, we can see that it is very simple to use Golang's RPC library to achieve cross-process communication. Just define the server-side method and register it in RPC, then establish a connection with the server-side on the client side and call the server-side method. The use of RPC can easily switch the underlying network protocol and supports multiple data encoding formats. In distributed system development, using Golang's RPC library can improve development efficiency and provide good performance for small and medium-sized distributed applications.
The above is the detailed content of Golang development: using RPC to achieve cross-process communication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


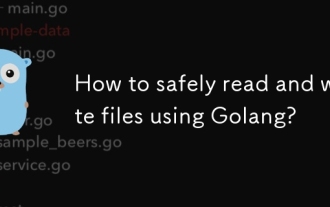
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
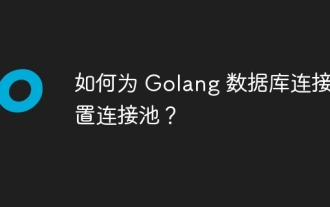
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
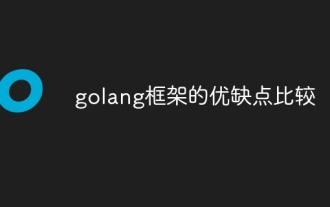
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
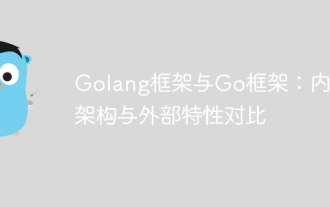
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
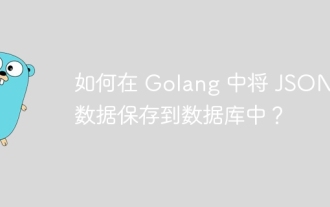
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
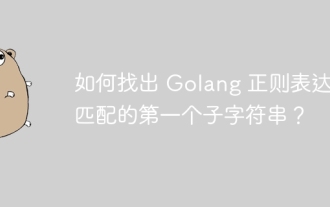
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
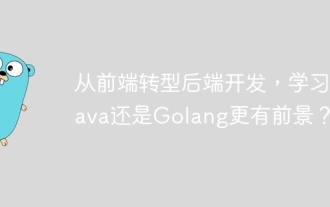
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
