How to use Vue to implement Zhihu-like comment effects
How to use Vue to implement Zhihu-like comment effects
In recent years, the number of Zhihu users has been growing, and many people like to communicate with others on Zhihu. Share and discuss. Among them, the comment function is a very important part. The implementation of special effects for Zhihu comments is crucial to improving user experience and website attractiveness. In this article, we will introduce how to use Vue to implement Zhihu-like comment effects and provide specific code examples.
- Basic Vue component structure
To implement Zhihu-like comment effects, you first need to establish a basic Vue component structure. Assume that our component is named Comment and has the following structure:
<template> <div class="comment"> <!-- 评论内容展示区域 --> <div class="comment-content">{{ comment }}</div> <!-- 评论回复区域 --> <div class="comment-reply"> <textarea v-model="reply"></textarea> <button @click="addReply">回复</button> </div> <!-- 子评论列表区域 --> <ul class="sub-comments"> <li v-for="subComment in subComments"> <Comment :comment="subComment"></Comment> </li> </ul> </div> </template> <script> export default { name: 'Comment', props: { comment: String // 评论内容 }, data() { return { reply: '', // 回复内容 subComments: [] // 子评论列表 } }, methods: { addReply() { // 添加回复到子评论列表 this.subComments.push(this.reply); this.reply = ''; // 清空回复内容 } } } </script> <style scoped> /* 样式省略 */ </style>
In the above code, we create a component named Comment through Vue's component definition method. This component includes a comment content display area, a comment reply area and a sub-comment list area. In the reply area, we use Vue's v-model directive to implement two-way data binding in order to obtain the reply content entered by the user in real time. In the sub-comment list area, we achieve infinite levels of sub-comment display by using recursive calls to the Comment component itself.
- Using the Comment component in the parent component
Before using the Comment component, we need to create a parent component first, and call and render the Comment component through the parent component. Assume that our parent component is named App. The code example is as follows:
<template> <div class="app"> <!-- 评论列表 --> <ul class="comments"> <li v-for="comment in comments"> <Comment :comment="comment"></Comment> </li> </ul> </div> </template> <script> import Comment from './components/Comment.vue'; export default { name: 'App', components: { Comment }, data() { return { comments: [] // 评论列表 } }, created() { // 初始化评论列表数据 this.comments = [ '这是一条评论1', '这是一条评论2' ]; } } </script> <style scoped> /* 样式省略 */ </style>
In the above code, we create a parent component named App through Vue's component definition method. We initialize an initial comment list data in the created hook function and pass it as props of the Comment component. By using the v-for directive, we can render this comment data list into multiple Comment components to achieve Zhihu-like comment effects.
Conclusion
Through the above code examples, we can see how to use Vue to implement Zhihu-like comment effects. Vue's componentization features and two-way data binding mechanism allow us to easily implement complex interface interaction effects. Of course, in actual projects, more functional expansion and detail optimization may be required according to needs, but the code introduced in this article has provided you with a basic framework for operation, which can be used as the basis for further development. I hope this article can be helpful to students who are learning Vue.
The above is the detailed content of How to use Vue to implement Zhihu-like comment effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


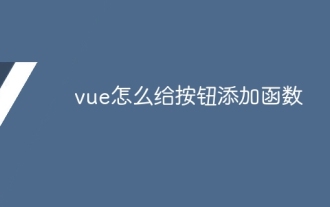
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
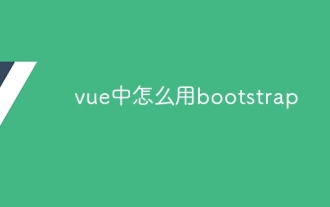
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
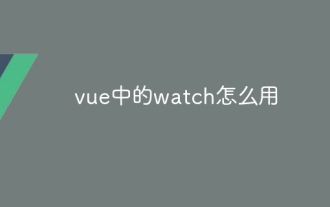
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
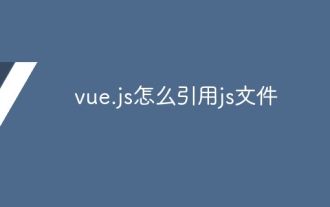
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
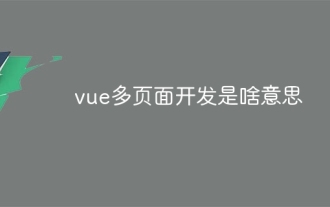
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
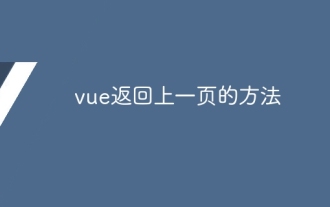
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
