


How to use PHP to develop simple online ordering and delivery functions
How to use PHP to develop simple online ordering and delivery functions
With the rapid development of the Internet, people's lifestyles have also changed. More and more people are ordering food at home and want the convenience of having food delivered to their doorstep. In this article, I will introduce how to use PHP to develop a simple online ordering and delivery function. At the same time, I will provide specific code examples to help you better understand and practice.
- Create database
First, we need to create a database to store the restaurant's menu and customer order information. In the MySQL database, we can use the following code to create a database named "restaurant":
CREATE DATABASE restaurant;
Next, we can create two tables to store menu and order information, the code example is as follows:
CREATE TABLE menu ( id INT(11) AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, price DECIMAL(10, 2) NOT NULL ); CREATE TABLE orders ( id INT(11) AUTO_INCREMENT PRIMARY KEY, customer_name VARCHAR(255) NOT NULL, customer_phone VARCHAR(15) NOT NULL, items TEXT NOT NULL, total_price DECIMAL(10, 2) NOT NULL, order_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
- Create a web interface
Next, we need to create a web interface for customers to browse the menu and place orders. Here is a simple HTML template example:
<!doctype html> <html> <head> <title>在线点餐</title> </head> <body> <h1 id="菜单">菜单</h1> <ul> <?php // 连接数据库 $conn = mysqli_connect("数据库地址", "用户名", "密码", "restaurant"); // 查询菜单数据 $query = "SELECT * FROM menu"; $result = mysqli_query($conn, $query); // 遍历菜单并显示在页面上 while ($row = mysqli_fetch_assoc($result)) { echo "<li>" . $row['name'] . " - ¥" . $row['price'] . "</li>"; } ?> </ul> <h1 id="下单">下单</h1> <form method="post" action="place_order.php"> <label>姓名:</label> <input type="text" name="customer_name" required><br> <label>电话:</label> <input type="text" name="customer_phone" required><br> <label>订单:</label> <textarea name="items" required></textarea><br> <button type="submit">提交订单</button> </form> </body> </html>
- Processing orders
When a customer submits an order, we need to process the order and save the order information to the database. The following is a sample code for processing orders:
<?php // 连接数据库 $conn = mysqli_connect("数据库地址", "用户名", "密码", "restaurant"); // 获取表单数据 $customer_name = $_POST['customer_name']; $customer_phone = $_POST['customer_phone']; $items = $_POST['items']; // 计算订单总价 $query = "SELECT SUM(price) AS total_price FROM menu WHERE id IN ($items)"; $result = mysqli_query($conn, $query); $row = mysqli_fetch_assoc($result); $total_price = $row['total_price']; // 保存订单信息到数据库 $query = "INSERT INTO orders (customer_name, customer_phone, items, total_price) VALUES ('$customer_name', '$customer_phone', '$items', '$total_price')"; mysqli_query($conn, $query); // 提示用户下单成功 echo "下单成功!"; // 关闭数据库连接 mysqli_close($conn); ?>
- Delivery order
When a new order is placed, we need a background service to process the order and deliver it food. This part can be developed according to specific needs and will not be elaborated here.
Summary:
Through the above steps, we can use PHP to develop a simple online ordering and delivery function. Customers can browse the menu and place their order, and the order information will be saved to the database. We also provide specific code examples to help you better understand and practice. Of course, there are many other functions and details that can be further improved and optimized and need to be developed according to actual needs.
I hope this article will be helpful to you, and I wish you good luck in developing online ordering and delivery functions using PHP!
The above is the detailed content of How to use PHP to develop simple online ordering and delivery functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


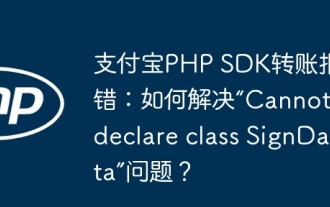
Alipay PHP...
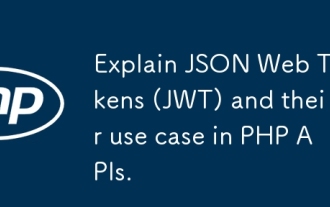
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
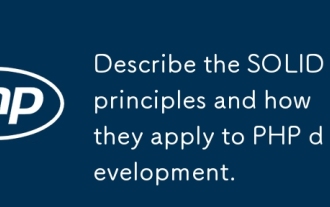
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
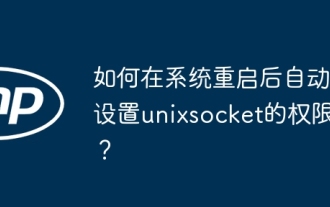
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
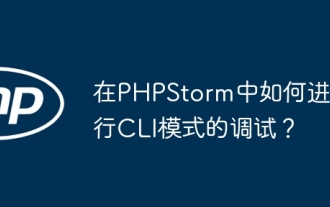
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
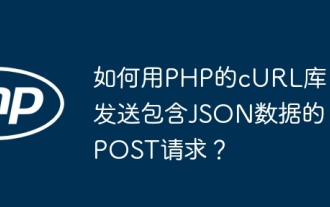
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
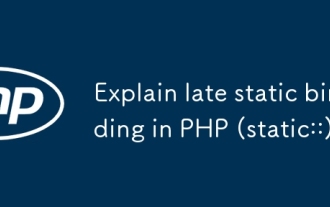
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
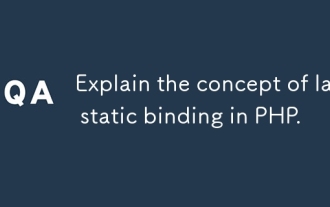
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
