How to use PHP to develop a simple message board function
Use PHP to develop a simple message board function
The message board is a very common website function, which allows users to post messages, comments and other content on the website. In this article, we will use the PHP programming language to develop a simple message board functionality. First, we need a PHP environment containing a database. Next, we will introduce step by step how to implement the message board function.
- Create database
First, we need to create a database to store the content of messages. You can use a database management system such as MySQL to create a database named "guestbook" and create a data table named "messages", which contains the following fields: - id: auto-increment primary key
- name: user name (varchar type)
- message: message content (text type)
- date: message time (timestamp type)
- Connect to the database
In In the PHP code, we need to connect to the database in order to read and write data. You can use the following code to connect to the database:
<?php $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "guestbook"; // 创建数据库连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } echo "连接成功"; ?>
- Display messages
Next, we need to read the messages from the database and display them on the web page. You can use the following code to read messages from the database:
<?php // SQL查询语句 $sql = "SELECT * FROM messages"; // 执行查询 $result = $conn->query($sql); // 检查查询结果是否为空 if ($result->num_rows > 0) { // 输出每一条留言 while ($row = $result->fetch_assoc()) { echo "用户名:" . $row["name"] . "<br>"; echo "留言内容:" . $row["message"] . "<br>"; echo "留言时间:" . $row["date"] . "<br><br>"; } } else { echo "暂无留言"; } // 关闭数据库连接 $conn->close(); ?>
- Submit messages
In addition to reading messages, we also need to provide a form to allow users to submit messages. You can use the following code to implement the message submission function:
<?php // 检查用户是否提交了留言 if (isset($_POST["submit"])) { // 获取用户输入的用户名和留言内容 $name = $_POST["name"]; $message = $_POST["message"]; // 插入数据到数据库 $sql = "INSERT INTO messages (name, message) VALUES ('$name', '$message')"; if ($conn->query($sql) === TRUE) { echo "留言成功"; } else { echo "留言失败:" . $conn->error; } } // 关闭数据库连接 $conn->close(); ?> <html> <body> <form method="post" action=""> <label for="name">用户名:</label> <input type="text" name="name" id="name"><br> <label for="message">留言内容:</label> <textarea name="message" id="message" rows="5" cols="30"></textarea><br> <input type="submit" name="submit" value="提交留言"> </form> </body> </html>
The above is all the code for using PHP to develop a simple message board function. Through the above steps, we can create a simple message board with message display and message submission functions. The code can be expanded and optimized as needed, such as adding user verification, comment reply and other functions. Hope this article helps you!
The above is the detailed content of How to use PHP to develop a simple message board function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
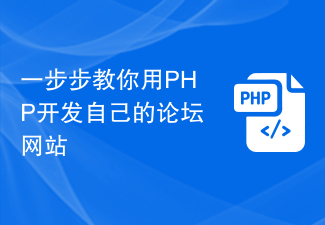
With the rapid development of the Internet and people's increasing demand for information exchange, forum websites have become a common online social platform. Developing a forum website of your own can not only meet your own personalized needs, but also provide a platform for communication and sharing, benefiting more people. This article will teach you step by step how to use PHP to develop your own forum website. I hope it will be helpful to beginners. First, we need to clarify some basic concepts and preparations. PHP (HypertextPreproces
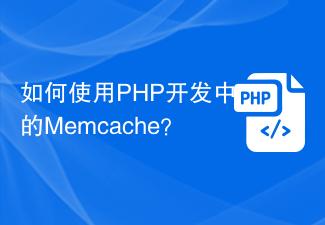
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
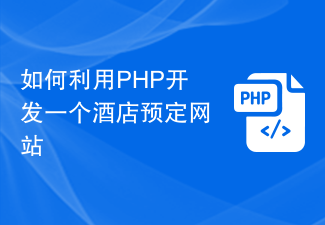
How to use PHP to develop a hotel booking website With the development of the Internet, more and more people are beginning to arrange their travels through online booking. As one of the common online booking services, hotel booking websites provide users with a convenient and fast way to book hotels. This article will introduce how to use PHP to develop a hotel reservation website, allowing you to quickly build and operate your own online hotel reservation platform. 1. System requirements analysis Before starting development, we need to conduct system requirements analysis first to clarify what the website we want to develop needs to have.
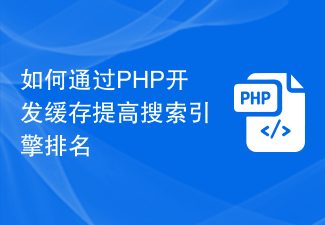
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
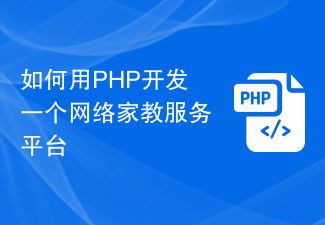
How to use PHP to develop an online tutoring service platform. With the rapid development of the Internet, online tutoring service platforms have attracted more and more people's attention and demand. Parents and students can easily find suitable tutors through such a platform, and tutors can also better demonstrate their teaching abilities and advantages. This article will introduce how to use PHP to develop an online tutoring service platform. First, we need to clarify the functional requirements of the platform. An online tutoring service platform needs to have the following basic functions: Registration and login system: users can
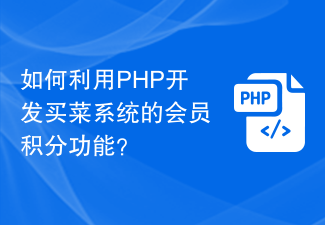
How to use PHP to develop the member points function of the grocery shopping system? With the rise of e-commerce, more and more people choose to purchase daily necessities online, including grocery shopping. The grocery shopping system has become the first choice for many people, and one of its important features is the membership points system. The membership points system can attract users and increase their loyalty, while also providing users with an additional shopping experience. In this article, we will discuss how to use PHP to develop the membership points function of the grocery shopping system. First, we need to create a membership table to store users
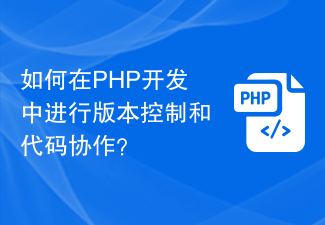
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
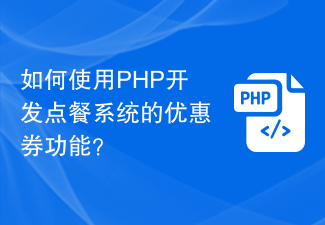
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
