Beginner's Guide to Java Development: From Getting Started to Giving Up
Java is a programming language widely used in software development. Its concise syntax and powerful functions make it the first choice for many developers. However, for beginners, learning Java may feel a little difficult. This article will provide a guide for Java development beginners to help them from getting started to giving up.
- Learn basic syntax
The basic syntax of Java includes variables, data types, operators, conditional statements, loop statements, etc. Beginners should start with these basic concepts and write simple code examples to deepen their understanding. The following is a sample code that calculates the sum of two numbers:
public class HelloWorld { public static void main(String[] args) { int a = 10; int b = 20; int sum = a + b; System.out.println("Sum: " + sum); } }
- Understanding object-oriented programming
Java is an object-oriented programming language. Beginners should learn classes and objects. , inheritance, encapsulation and other concepts, and write corresponding code examples to practice. The following is a simple sample code for a student class:
public class Student { private String name; private int age; public Student(String name, int age) { this.name = name; this.age = age; } public void introduce() { System.out.println("Name: " + name); System.out.println("Age: " + age); } public static void main(String[] args) { Student student = new Student("John", 20); student.introduce(); } }
- Master common APIs
Java provides many commonly used APIs, such as string processing, file operations, collections, etc. Beginners should have a deep understanding of these APIs and write relevant code examples to deepen their memory and understanding. The following is a sample code using a collection:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); for (String name : names) { System.out.println(name); } } }
- Learn debugging skills
During the development process, debugging is a very important skill. Beginners should learn to use debugging tools to find errors in their code and fix them. Here is a sample code that uses debugging skills to find errors:
public class DebugExample { public static void main(String[] args) { int sum = 0; for (int i = 1; i <= 10; i++) { sum += i; } System.out.println("Sum: " + sum); } }
- Learn common programming practices
Good programming practices are about writing clear and readable code The essential. Beginners should learn and abide by common programming conventions, such as naming conventions, indentation conventions, comment conventions, etc. The following is a sample code that complies with programming standards:
public class CodeExample { private static final int MAX_COUNT = 100; public static void main(String[] args) { int sum = 0; for (int i = 1; i <= MAX_COUNT; i++) { sum += i; } System.out.println("Sum: " + sum); } }
Through the above-mentioned aspects, beginners can gradually master the basic knowledge and skills of Java programming. Of course, learning programming is not an overnight process and requires continuous practice and accumulation of experience. I hope this article can provide some guidance for beginners and help them from getting started to giving up. However, remember that giving up is not the answer to the problem; perseverance is the key to success.
The above is the detailed content of Beginner's Guide to Java Development: From Getting Started to Giving Up. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



With the development of artificial intelligence technology, Natural Language Processing (NLP) has become a very important technology. NLP can help us better understand and analyze human language to achieve some automated tasks, such as intelligent customer service, sentiment analysis, machine translation, etc. In this article, we will cover the basics and tools for natural language processing using PHP. What is natural language processing? Natural language processing is a method that uses artificial intelligence technology to process

As the Internet continues to develop, the demand for front-end and back-end technologies is also increasing. As a back-end developer, mastering PHP is essential. In PHP development, we often need to process requests and responses. This article will discuss the PATCH request and response, providing a practical guide for PHP beginners. 1. PATCH request The PATCH request is an HTTP request method, which is used to update existing resources. In the HTTP protocol, there is a way to use a PUT request to
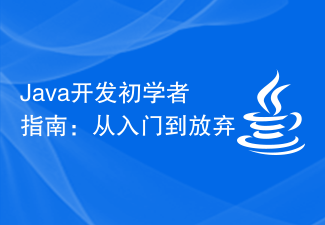
Java is a programming language widely used in software development. Its concise syntax and powerful functions make it the first choice for many developers. However, for beginners, learning Java may feel a little difficult. This article will provide a guide for Java development beginners to help them from getting started to giving up. Learn basic syntax. The basic syntax of Java includes variables, data types, operators, conditional statements, loop statements, etc. Beginners should start with these basic concepts and write simple code examples to deepen their understanding.

PHP is a popular server-side scripting language commonly used for web development, while YAML is a lightweight data serialization format used for configuration files and data exchange. In this article, we'll explore how PHP works with YAML and how to get started. PHP and YAML When developing web applications, developers need to deal with a large amount of data and configuration. These data and configurations can be stored in a database or using text files. Text files usually use XML, JSON or YA
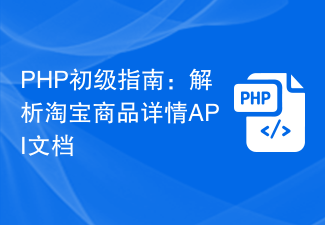
Introduction to PHP Technology: Taobao Product Details API Document Interpretation Introduction: PHP, as a programming language widely used in Web development, has a large user group and a rich extension library. Among them, using PHP to develop Taobao product details API is a very practical and common requirement. This article will provide a detailed interpretation of the Taobao product details API document to provide an introductory guide for beginners. 1. What is Taobao Product Details API? Taobao Product Details API is an interface provided by Taobao open platform.

Starting from scratch: PHP WebSocket development introductory guide and function implementation tutorial 1. Introduction With the development of the Internet, the demand for real-time communication is increasing. As a new real-time communication protocol, WebSocket has gradually attracted the attention and use of developers. This article will use PHP as the development language to introduce the basic concepts of WebSocket, and provide an introductory development guide suitable for beginners to help readers implement WebSocket functions from scratch. 2. WebSocket
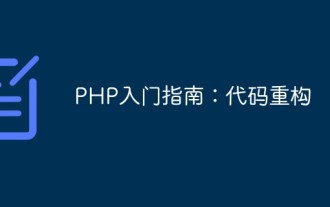
Refactoring is a very important process when writing PHP code. As an application grows, the code base becomes larger and harder to read and maintain. Refactoring is to solve this problem and make the code more modular and better organized and extensible. When we refactor the code, we need to consider the following aspects: Code style Code style is a very important point. Keeping your coding style consistent will make your code easier to read and maintain. Please follow PHP coding standards and be consistent. Try using a code style checking tool such as PHP
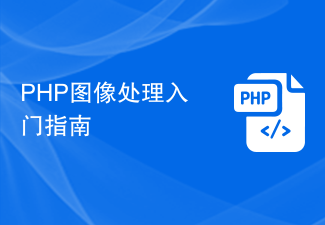
PHP is a very popular server-side programming language and it is widely used for web development. In web development, image processing is a very common requirement, and it is also very simple to implement image processing in PHP. This article will briefly introduce the introductory guide to PHP image processing. 1. Environmental requirements To use PHP image processing, you first need the support of the PHPGD library. The library provides functionality for writing images to files or outputting them to a browser, cropping and scaling images, adding text, and making images grayscale or inverting. therefore
