How to write custom stored procedures and functions in MySQL using C#
How to write custom stored procedures and functions in MySQL using C
#Introduction:
MySQL is a widely used open source database management system, and C# is A commonly used object-oriented programming language. During the development process, we often need to use database stored procedures and functions to improve code reusability and performance. This article will introduce how to use C# to write custom stored procedures and functions in a MySQL database, and provide specific code examples.
1. Stored procedures
A stored procedure is a set of SQL statements that perform specific tasks. The following will introduce in detail how to write custom stored procedures in MySQL using C#.
1.1 Create a stored procedure
First, create a new database in MySQL and create a data table named "TestDB". The table structure is as follows:
CREATE TABLE TestTable
(
id
int(11) NOT NULL AUTO_INCREMENT,
name
varchar(100) DEFAULT NULL,
age
int(11) DEFAULT NULL,
PRIMARY KEY (id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Next, create a new C# console application in Visual Studio. Connect to the database via the MySQL connection string, and then execute the following code to create a stored procedure named "GetAllData":
using MySql.Data.MySqlClient;
namespace MySQLExample
{
class Program { static void Main(string[] args) { string connectionString = "server=localhost;username=root;password=password;database=TestDB"; MySqlConnection connection = new MySqlConnection(connectionString); MySqlCommand cmd = new MySqlCommand(); cmd.Connection = connection; cmd.CommandText = "CREATE PROCEDURE GetAllData() " + "BEGIN " + "SELECT * FROM TestTable; " + "END"; try { connection.Open(); cmd.ExecuteNonQuery(); Console.WriteLine("存储过程创建成功!"); } catch (Exception ex) { Console.WriteLine(ex.Message); } finally { connection.Close(); } Console.ReadKey(); } }
}
The above code creates a stored procedure named "GetAllData" by executing the CREATE PROCEDURE statement. This stored procedure can be called directly in the database and will return all the data in the TestTable table.
1.2 Calling the stored procedure
Next, let’s demonstrate how to call the just created stored procedure in C#.
Based on the previous step, we can write the following code to call the stored procedure and get the returned data:
using MySql.Data.MySqlClient;
namespace MySQLExample
{
class Program { static void Main(string[] args) { string connectionString = "server=localhost;username=root;password=password;database=TestDB"; MySqlConnection connection = new MySqlConnection(connectionString); MySqlCommand cmd = new MySqlCommand(); cmd.Connection = connection; cmd.CommandText = "GetAllData"; cmd.CommandType = CommandType.StoredProcedure; try { connection.Open(); MySqlDataReader reader = cmd.ExecuteReader(); while (reader.Read()) { Console.WriteLine(reader["id"].ToString() + " " + reader["name"].ToString() + " " + reader["age"].ToString()); } reader.Close(); } catch (Exception ex) { Console.WriteLine(ex.Message); } finally { connection.Close(); } Console.ReadKey(); } }
}
The above code obtains all the data in the TestTable table by executing the "GetAllData" stored procedure, and outputs the results to the console.
2. Function
The function is a reusable SQL code block that calculates the value of the input parameter and returns a value. The following will introduce in detail how to write custom functions in MySQL using C#.
2.1 Create function
Create a function named "GetAverageAge" in MySQL, which will calculate the average age of all people in the TestTable table and return it.
The code to create the function is as follows:
CREATE FUNCTION GetAverageAge
() RETURNS INT(11)
BEGIN
DECLARE avg_age INT(11);
SELECT AVG(age) INTO avg_age FROM TestTable;
RETURN avg_age;
END
2.2 Call the function
Use the following code to call the function just created and get the returned value:
using MySql.Data.MySqlClient;
namespace MySQLExample
{
class Program { static void Main(string[] args) { string connectionString = "server=localhost;username=root;password=password;database=TestDB"; MySqlConnection connection = new MySqlConnection(connectionString); try { connection.Open(); MySqlCommand cmd = new MySqlCommand("SELECT GetAverageAge()", connection); int averageAge = Convert.ToInt32(cmd.ExecuteScalar()); Console.WriteLine("平均年龄:" + averageAge); } catch (Exception ex) { Console.WriteLine(ex.Message); } finally { connection.Close(); } Console.ReadKey(); } }
}
The above code is called by executing the SELECT GetAverageAge() statement "GetAverageAge" function and get the average age returned by the function.
Conclusion:
This article introduces how to use C# to write custom stored procedures and functions in MySQL, and provides specific code examples. Stored procedures and functions can improve code reusability and performance and are suitable for various complex data processing needs. By using C# to connect to the MySQL database, we can flexibly write and call stored procedures and functions, thereby giving full play to the advantages of the MySQL database.
The above is the detailed content of How to write custom stored procedures and functions in MySQL using C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


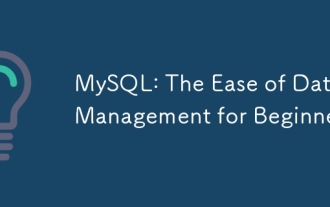
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
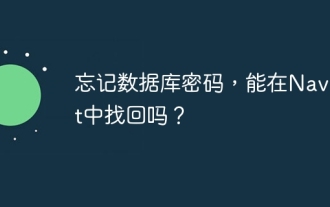
Navicat itself does not store the database password, and can only retrieve the encrypted password. Solution: 1. Check the password manager; 2. Check Navicat's "Remember Password" function; 3. Reset the database password; 4. Contact the database administrator.
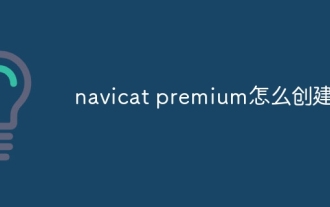
Create a database using Navicat Premium: Connect to the database server and enter the connection parameters. Right-click on the server and select Create Database. Enter the name of the new database and the specified character set and collation. Connect to the new database and create the table in the Object Browser. Right-click on the table and select Insert Data to insert the data.
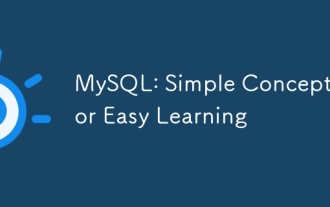
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
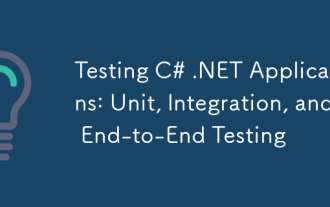
Testing strategies for C#.NET applications include unit testing, integration testing, and end-to-end testing. 1. Unit testing ensures that the minimum unit of the code works independently, using the MSTest, NUnit or xUnit framework. 2. Integrated tests verify the functions of multiple units combined, commonly used simulated data and external services. 3. End-to-end testing simulates the user's complete operation process, and Selenium is usually used for automated testing.
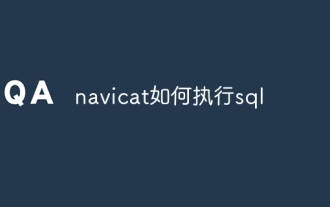
Steps to perform SQL in Navicat: Connect to the database. Create a SQL Editor window. Write SQL queries or scripts. Click the Run button to execute a query or script. View the results (if the query is executed).
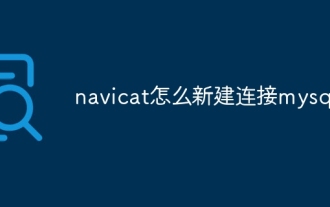
You can create a new MySQL connection in Navicat by following the steps: Open the application and select New Connection (Ctrl N). Select "MySQL" as the connection type. Enter the hostname/IP address, port, username, and password. (Optional) Configure advanced options. Save the connection and enter the connection name.
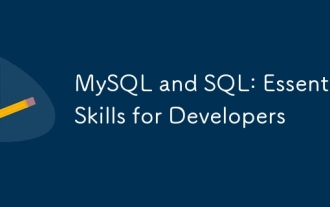
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
