Golang Development: Building Scalable Distributed Systems
Golang development: Building scalable distributed systems requires specific code examples
With the development of the Internet and the advancement of technology, distributed systems are becoming cloud computing and core technologies in the field of big data. Distributed systems have the advantages of flexibility, scalability, and high availability, and can meet today's needs for high concurrency and large-scale data processing. In the development of distributed systems, choosing an appropriate programming language is crucial. As a fast, efficient and safe programming language, Golang is increasingly favored by developers. This article will introduce how to use Golang to build a scalable distributed system and provide specific code examples.
The key to building distributed systems in Golang is to use message passing and concurrent programming. Golang provides a wealth of libraries and tools that allow developers to easily build distributed systems, handle large numbers of requests concurrently, and achieve high availability. The following is a code example for building a scalable distributed system using Golang:
package main import ( "fmt" "sync" ) type Counter struct { mu sync.Mutex count int } func (c *Counter) Increment() { c.mu.Lock() defer c.mu.Unlock() c.count++ } func (c *Counter) GetCount() int { c.mu.Lock() defer c.mu.Unlock() return c.count } func main() { counter := Counter{ count: 0, } var wg sync.WaitGroup max := 10000 wg.Add(max) for i := 0; i < max; i++ { go func() { defer wg.Done() counter.Increment() }() } wg.Wait() fmt.Println("Final count:", counter.GetCount()) }
In the above example, we define a Counter
structure that contains a mutex lock and a counter. Increment
The method uses a mutex lock to ensure that only one Goroutine can increment the counter at the same time. The GetCount
method also uses a mutex lock to ensure thread safety when reading the counter.
In the main
function, we create a Counter
instance and use sync.WaitGroup
to wait for all Goroutines to complete execution. Then, we started a series of Goroutines to call the Increment
method concurrently, thus achieving the effect of concurrently incrementing the counter. Finally, we get the final count value by calling the GetCount
method and print it to the console.
The above example demonstrates how to use Golang to build a counter component in a scalable distributed system. In practical applications, we can build more complex distributed systems based on this example, such as distributed caches, message queues, etc. By properly designing and using Golang's concurrency mechanism, we can achieve an efficient and scalable distributed system.
In short, Golang is an ideal programming language for building scalable distributed systems, which is fast, efficient, and safe. By rationally utilizing Golang's concurrency mechanism and message passing mechanism, we can implement a flexible and highly available distributed system. I hope the examples in this article will help you understand how to use Golang to build scalable distributed systems.
The above is the detailed content of Golang Development: Building Scalable Distributed Systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
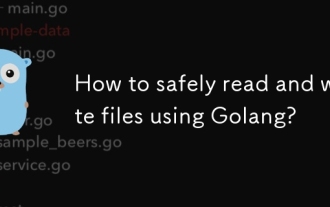
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
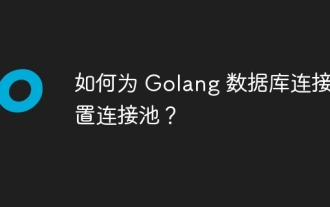
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
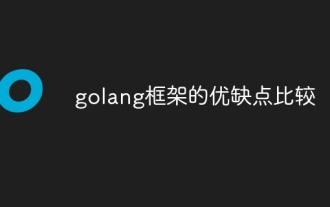
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
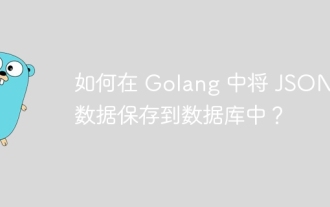
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
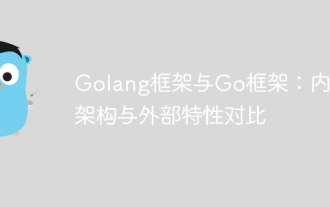
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
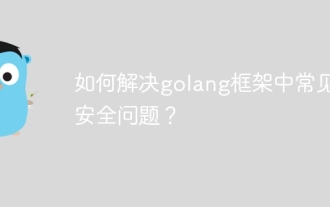
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
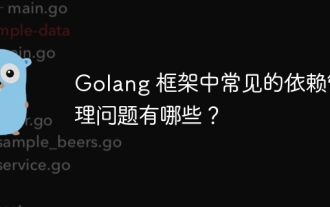
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
