How to implement image storage and processing functions of data in MongoDB
How to implement image storage and processing functions of data in MongoDB
Overview:
In the development of modern data applications, image processing and storage is a Common needs. MongoDB, a popular NoSQL database, provides features and tools that enable developers to implement image storage and processing on its platform. This article will introduce how to implement image storage and processing functions of data in MongoDB, and provide specific code examples.
Image storage:
In MongoDB, you can use the GridFS (Grid File System) function to store image files. GridFS makes it possible to store files larger than 16MB by splitting large files into small chunks and then storing these chunks in collections. GridFS stores files as two collections: fs.files is used to save the metadata of the file, and fs.chunks is used to save the chunks of the file. Below is a sample code that shows how to use GridFS to store image files in MongoDB.
from pymongo import MongoClient from gridfs import GridFS # 连接MongoDB client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] fs = GridFS(db) # 读取图像文件 with open('image.jpg', 'rb') as f: data = f.read() # 存储图像文件 file_id = fs.put(data, filename='image.jpg') print('File stored with id:', file_id)
Image processing:
MongoDB provides some built-in operators and functions that can be used for image processing in queries. Here are some examples of commonly used image processing operations:
Resize image
from PIL import Image # 读取图像文件 with open('image.jpg', 'rb') as f: data = f.read() # 调整图像大小 img = Image.open(io.BytesIO(data)) resized_img = img.resize((500, 500)) # 存储调整后的图像文件 resized_img.save('resized_image.jpg')
Copy after loginImage rotation
from PIL import Image # 读取图像文件 with open('image.jpg', 'rb') as f: data = f.read() # 图像旋转 img = Image.open(io.BytesIO(data)) rotated_img = img.rotate(90) # 存储旋转后的图像文件 rotated_img.save('rotated_image.jpg')
Copy after login-
Image filter
from PIL import Image, ImageFilter # 读取图像文件 with open('image.jpg', 'rb') as f: data = f.read() # 图像滤镜 img = Image.open(io.BytesIO(data)) filtered_img = img.filter(ImageFilter.BLUR) # 存储滤镜后的图像文件 filtered_img.save('filtered_image.jpg')
Copy after loginSummary:
By using MongoDB’s GridFS function, we can easily store large image files in MongoDB. At the same time, MongoDB also provides some built-in operators and functions, allowing us to perform some simple image processing operations in queries. The above code example shows how to use GridFS to store image files and use the Pillow library to perform some simple image processing operations. By further learning and using these features, developers can implement more complex image storage and processing functions in MongoDB.The above is the detailed content of How to implement image storage and processing functions of data in MongoDB. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


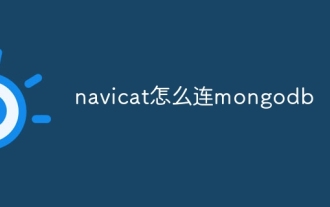
To connect to MongoDB using Navicat, you need to: Install Navicat Create a MongoDB connection: a. Enter the connection name, host address and port b. Enter the authentication information (if required) Add an SSL certificate (if required) Verify the connection Save the connection

.NET 4.0 is used to create a variety of applications and it provides application developers with rich features including: object-oriented programming, flexibility, powerful architecture, cloud computing integration, performance optimization, extensive libraries, security, Scalability, data access, and mobile development support.
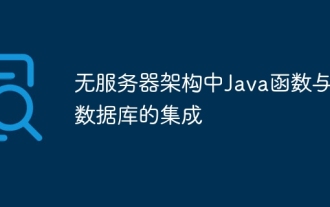
In a serverless architecture, Java functions can be integrated with the database to access and manipulate data in the database. Key steps include: creating Java functions, configuring environment variables, deploying functions, and testing functions. By following these steps, developers can build complex applications that seamlessly access data stored in databases.
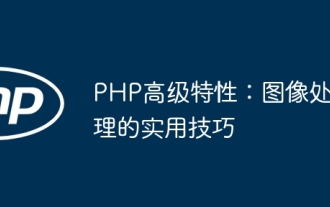
PHP provides advanced image processing techniques, including scaling and cropping, image synthesis, filters, conversions, etc. Practical examples show how to use these techniques to create thumbnails that save loading time and showcase images. By understanding these technologies, you can improve your image processing capabilities and enhance application functionality.

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
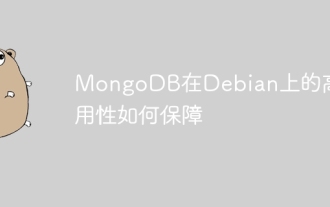
This article describes how to build a highly available MongoDB database on a Debian system. We will explore multiple ways to ensure data security and services continue to operate. Key strategy: ReplicaSet: ReplicaSet: Use replicasets to achieve data redundancy and automatic failover. When a master node fails, the replica set will automatically elect a new master node to ensure the continuous availability of the service. Data backup and recovery: Regularly use the mongodump command to backup the database and formulate effective recovery strategies to deal with the risk of data loss. Monitoring and Alarms: Deploy monitoring tools (such as Prometheus, Grafana) to monitor the running status of MongoDB in real time, and
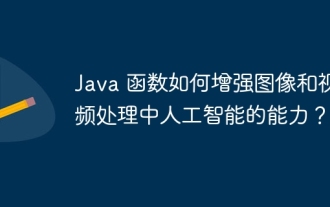
Java functions significantly enhance AI applications in image and video processing by providing: image enhancement (adjustment of brightness, contrast, etc.), target detection, image classification; video transcoding, video analysis, video editing and other capabilities. Using a pre-trained model, such as the Haar cascade classifier, face detection can be deployed in a Java function that detects faces in the incoming image and plots the detection results.
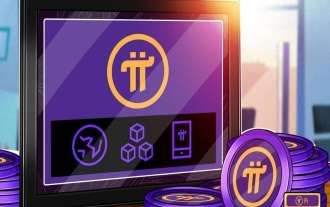
PiNetwork is about to launch PiBank, a revolutionary mobile banking platform! PiNetwork today released a major update on Elmahrosa (Face) PIMISRBank, referred to as PiBank, which perfectly integrates traditional banking services with PiNetwork cryptocurrency functions to realize the atomic exchange of fiat currencies and cryptocurrencies (supports the swap between fiat currencies such as the US dollar, euro, and Indonesian rupiah with cryptocurrencies such as PiCoin, USDT, and USDC). What is the charm of PiBank? Let's find out! PiBank's main functions: One-stop management of bank accounts and cryptocurrency assets. Support real-time transactions and adopt biospecies
