Python program to find all subsets of a string
In Python, a subset of a string is a sequence of characters that is part of the original string. We can find all subsets of a string using the itertools module in Python. In this article, we will see how to generate all subsets of a string by making all possible combinations of the characters in the string.
grammar
itertools.combination(string,r)
The combination() function of the itertools module accepts a string and r, where r represents the size of possible different string combinations. It returns all possible character combinations of the string.
algorithm
Initialize an empty list called a combination
Use a for loop and the itertools.combination function to generate all possible character combinations in the string.
Filter out content that is not a subset of the original string
Return subset
Example
is:Example
In the following example, we first import the itertools module to generate all possible character combinations in a string. The find_subsets() function accepts a string as input and returns all possible subsets of the string. The find_subset() method first creates an empty list to store all subsets. Then with the help of for loop and itertools.combination() function, it generates all possible subsets of the string and stores them in combination list. After all the combinations have been generated and stored, we need to filter out strings that are not a subset of the original string and store such subsets in a list called subset. This subset is then returned by the function as all possible subsets of the string.
import itertools def find_subsets(string): # Get all possible combinations of characters in the string combinations = [] for i in range(len(string) + 1): combinations += itertools.combinations(string, i) # Filter out the ones that are not subsets of the original string subsets = [] for c in combinations: subset = ''.join(c) if subset != '': subsets.append(subset) return subsets # Test the function string = 'abc' subsets = find_subsets(string) print(subsets)
Output
['a', 'b', 'c', 'ab', 'ac', 'bc', 'abc']
in conclusion
In this article, we discussed how to generate all possible subsets of a string using the itertools module in Python. Once we have generated all possible combinations of characters in a string, we need to filter out strings that are not a subset of the original string. As a result, we get all possible subsets of the string.
The above is the detailed content of Python program to find all subsets of a string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
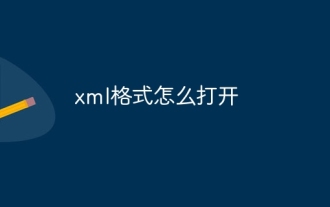
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
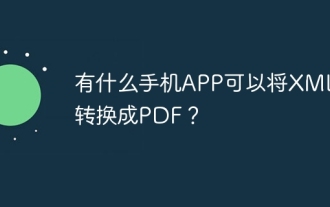
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
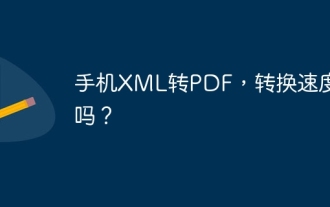
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
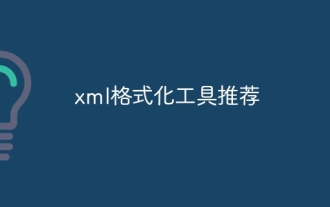
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
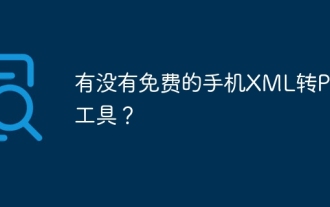
There is no simple and direct free XML to PDF tool on mobile. The required data visualization process involves complex data understanding and rendering, and most of the so-called "free" tools on the market have poor experience. It is recommended to use computer-side tools or use cloud services, or develop apps yourself to obtain more reliable conversion effects.
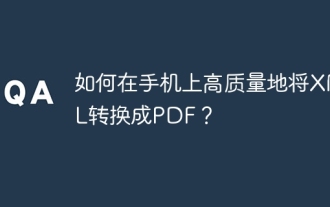
Convert XML to PDF with high quality on your mobile phone requires: parsing XML in the cloud and generating PDFs using a serverless computing platform. Choose efficient XML parser and PDF generation library. Handle errors correctly. Make full use of cloud computing power to avoid heavy tasks on your phone. Adjust complexity according to requirements, including processing complex XML structures, generating multi-page PDFs, and adding images. Print log information to help debug. Optimize performance, select efficient parsers and PDF libraries, and may use asynchronous programming or preprocessing XML data. Ensure good code quality and maintainability.
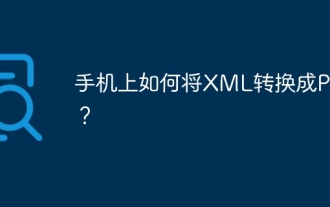
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
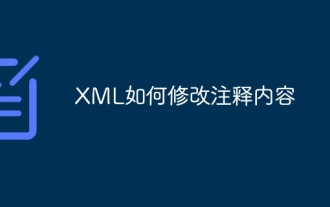
For small XML files, you can directly replace the annotation content with a text editor; for large files, it is recommended to use the XML parser to modify it to ensure efficiency and accuracy. Be careful when deleting XML comments, keeping comments usually helps code understanding and maintenance. Advanced tips provide Python sample code to modify comments using XML parser, but the specific implementation needs to be adjusted according to the XML library used. Pay attention to encoding issues when modifying XML files. It is recommended to use UTF-8 encoding and specify the encoding format.
