


How to use PHP microservices to implement distributed machine learning and intelligent recommendations
How to use PHP microservices to implement distributed machine learning and intelligent recommendations
Overview:
With the rapid development of the Internet, the explosive growth of data volume has made traditional The machine learning algorithm cannot meet the needs of big data analysis and intelligent recommendation. To address this challenge, distributed machine learning and intelligent recommendation technologies emerged. This article will introduce how to use PHP microservices to implement distributed machine learning and intelligent recommendations, and provide relevant code examples.
- System architecture design
When designing distributed machine learning and intelligent recommendation systems, the following aspects need to be considered: - Data storage: use a distributed storage system (such as Hadoop, Cassandra, etc.) to store massive data.
- Data preprocessing: Use a distributed computing framework (such as Spark) to preprocess data, such as data cleaning, feature extraction, etc.
- Model training: Use distributed machine learning algorithms (such as TensorFlow, XGBoost, etc.) to train the preprocessed data and generate models.
- Model inference: Use a distributed computing framework to deploy models to multiple servers to achieve intelligent recommendations.
- Use PHP microservices to implement distributed machine learning and intelligent recommendations
Since the PHP language is widely used in web development, using PHP microservices to implement distributed machine learning and intelligent recommendations has high flexibility and scalability.
2.1 Data Storage
In PHP microservices, NoSQL databases (such as MongoDB) can be used as distributed storage systems to store massive data. The following is a sample code for using MongoDB to store data:
<?php // 连接MongoDB $mongo = new MongoDBClient("mongodb://localhost:27017"); // 选择数据库 $db = $mongo->mydb; // 选择集合 $collection = $db->mycollection; // 插入数据 $data = array("name" => "John", "age" => 25); $collection->insertOne($data); // 查询数据 $result = $collection->findOne(array("name" => "John")); print_r($result); ?>
2.2 Data preprocessing
Data preprocessing is a very critical step in machine learning. You can use PHP microservices and distributed computing frameworks (such as Apache Spark ) are combined to achieve this. The following is a sample code for data preprocessing using Spark:
<?php // 创建SparkSession $spark = SparkSparkSession::builder() ->appName("Data Preprocessing") ->getOrCreate(); // 读取数据 $data = $spark->read()->format("csv") ->option("header", "true") ->load("data.csv"); // 数据清洗 $data = $data->filter($data["age"] > 18); // 特征提取 $vectorAssembler = new SparkFeatureVectorAssembler(); $vectorAssembler->setInputCols(["age"]) ->setOutputCol("features"); $data = $vectorAssembler->transform($data); // 打印数据 $data->show(); ?>
2.3 Model training
Model training is the core part of distributed machine learning, which can use PHP microservices and distributed machine learning frameworks (such as TensorFlow , XGBoost, etc.). The following is a sample code for model training using TensorFlow:
<?php // 加载TensorFlow库 require_once "tensorflow.php"; // 创建TensorFlow会话 $session = new TensorFlowSession(); // 定义模型 $input = new TensorFlowTensor(TensorFlowDataType::FLOAT, [2, 2]); $const = TensorFlowMath::add($input, TensorFlowMath::scalar(TensorFlowDataType::FLOAT, 2.0)); $output = $session->run([$const], [$input->initWithValue([[1.0, 2.0], [3.0, 4.0]])]); // 打印结果 print_r($output); ?>
2.4 Model Inference
Model inference is the core part of intelligent recommendation. You can use PHP microservices and distributed computing framework to deploy the model and make recommendations The results are returned to the client. The following is a sample code for model inference using PHP microservices:
<?php // 加载模型 $model = new MyModel(); // 接收客户端请求 $input = $_POST["input"]; // 调用模型推断 $output = $model->predict($input); // 返回推荐结果给客户端 echo $output; ?>
Summary:
This article introduces how to use PHP microservices to implement distributed machine learning and intelligent recommendations. By combining distributed storage systems, distributed computing frameworks, and distributed machine learning algorithms, big data can be effectively processed and intelligent recommendations can be achieved. Through the demonstration of sample code, readers can further understand and practice related technologies, and explore the application prospects of PHP in the field of big data.
The above is the detailed content of How to use PHP microservices to implement distributed machine learning and intelligent recommendations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


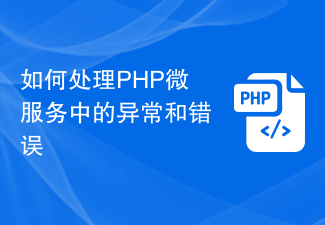
How to handle exceptions and errors in PHP microservices Introduction: With the popularity of microservice architecture, more and more developers choose to use PHP to implement microservices. However, due to the complexity of microservices, exception and error handling have become an essential topic. This article will introduce how to correctly handle exceptions and errors in PHP microservices and demonstrate it through specific code examples. 1. Exception handling In PHP microservices, exception handling is essential. Exceptions are unexpected situations encountered by the program during operation, such as database connection failure, A
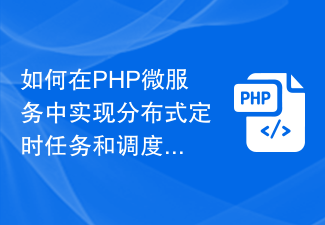
How to implement distributed scheduled tasks and scheduling in PHP microservices In modern microservice architecture, distributed scheduled tasks and scheduling are very important components. They can help developers easily manage, schedule and execute scheduled tasks in multiple microservices, improving system reliability and scalability. This article will introduce how to use PHP to implement distributed timing tasks and scheduling, and provide code examples for reference. Using a queue system In order to implement distributed scheduled tasks and scheduling, you first need to use a reliable queue system. Queuing systems can
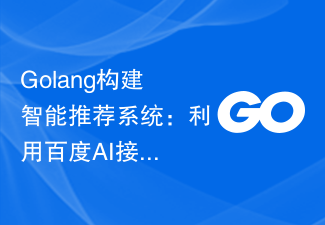
Golang builds an intelligent recommendation system: using Baidu AI interface to implement product recommendation Introduction: With the development of the Internet, people's shopping habits have also changed. More and more users choose to purchase goods online, citing convenience, speed and rich choices as the main reasons. However, finding the product that suits you among the vast amount of products can be a challenge. In order to solve this problem, the recommendation system has become an indispensable part of the e-commerce platform. In this article, we will introduce how to use Golang and Baidu AI interface to build an intelligent recommendation
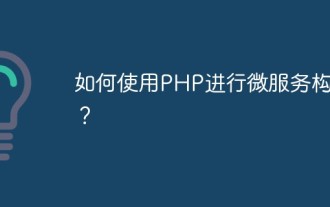
With the continuous development of the Internet and the continuous advancement of computer technology, microservice architecture has gradually become a hot topic in recent years. Different from the traditional monolithic application architecture, the microservice architecture decomposes a complex software application into multiple independent service units. Each service unit can be deployed, run and updated independently. The advantage of this architecture is that it improves the flexibility, scalability, and maintainability of the system. As an open source, Web-based programming language, PHP also plays a very important role in the microservice architecture.

How to use PHP microservices to achieve distributed transaction management and processing. With the rapid development of the Internet, it is increasingly difficult for single applications to meet user needs, and distributed architecture has become mainstream. In a distributed architecture, distributed transaction management and processing has become an important issue. This article will introduce how to use PHP microservices to implement distributed transaction management and processing, and give specific code examples. 1. What is distributed transaction management? Distributed transaction means that a business operation involves multiple independent data sources, and these data sources are required to be consistent.
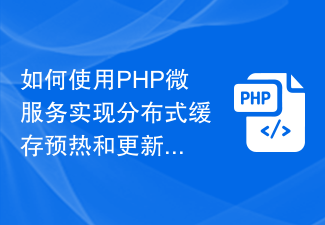
How to use PHP microservices to implement distributed cache warm-up and update Introduction: In modern web applications, caching is one of the important technical means to improve performance and reduce database load. The distributed cache can further improve the scalability and pressure resistance of the system. This article will introduce how to use PHP microservices to implement distributed cache warm-up and update, and provide some specific code examples. Requirements analysis: Our goal is to achieve two key functions through microservices: Cache warm-up: when the system starts, obtain data from the database and store it
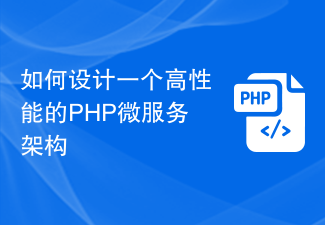
How to design a high-performance PHP microservice architecture. With the rapid development of the Internet, microservice architecture has become the first choice for many enterprises to build high-performance applications. As a lightweight, modular architectural style, microservices can split complex applications into smaller, independent service units, providing better scalability, reliability and maintainability through mutual cooperation. This article will introduce how to design a high-performance PHP microservice architecture and provide specific code examples. 1. Split microservices Before designing a high-performance PHP microservice architecture,
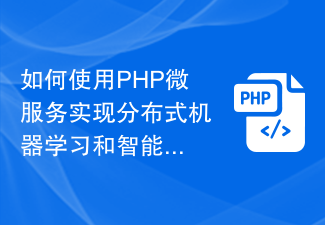
Overview of how to use PHP microservices to implement distributed machine learning and intelligent recommendations: With the rapid development of the Internet, the explosive growth of data volume makes traditional machine learning algorithms unable to meet the needs of big data analysis and intelligent recommendations. To address this challenge, distributed machine learning and intelligent recommendation technologies emerged. This article will introduce how to use PHP microservices to implement distributed machine learning and intelligent recommendations, and provide relevant code examples. System Architecture Design When designing distributed machine learning and intelligent recommendation systems, the following aspects need to be considered:
