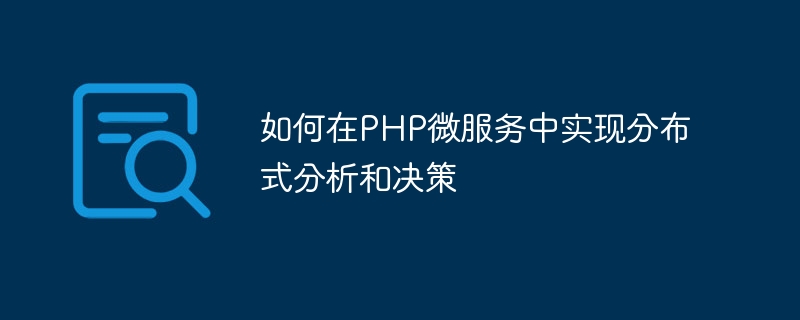
How to implement distributed analysis and decision-making in PHP microservices
Abstract: With the rapid development of the Internet and big data technology, distributed analysis and decision-making are increasingly important in enterprises. are becoming increasingly important. This article will introduce how to implement distributed analysis and decision-making in PHP microservices, and provide specific code examples.
- Introduction
With the rapid development of the Internet, enterprises are faced with more and more data, which require analysis and decision-making to support business development. The traditional single-machine architecture often cannot meet the needs of large-scale data processing. Therefore, distributed analysis and decision-making have become the first choice for enterprises.
- Distributed architecture design
In PHP microservices, realizing distributed analysis and decision-making requires a reasonable design of the distributed architecture. The following is a simple distributed architecture design example:
- A master service node (Master Node): responsible for overall scheduling and coordination, and providing external API interfaces.
- Multiple Worker Nodes: Responsible for specific analysis and decision-making tasks, and task distribution through the main control node.
- Task Distribution and Execution
On the main control service node, we can achieve task distribution through message queues (such as RabbitMQ, Kafka). The specific steps are as follows:
- After receiving the request, the main control service node sends the task information to the message queue.
- The worker node obtains task information from the message queue and begins to perform specific analysis and decision-making tasks.
- After the task execution is completed, the working node returns the results to the master node.
The following is a simple PHP code example:
<?php
// 主控服务节点代码
// 发布任务到消息队列
function sendTaskToQueue($task) {
$queue = new RabbitMQ();
$queue->push($task);
}
// 接收来自工作节点的任务结果
function receiveTaskResult() {
$queue = new RabbitMQ();
$result = $queue->pop();
// 处理结果...
}
// 工作节点代码
// 从消息队列中获取任务
function getTaskFromQueue() {
$queue = new RabbitMQ();
$task = $queue->pop();
return $task;
}
// 执行任务
function executeTask($task) {
// 执行具体的分析和决策任务...
$result = analysisAndDecision($task);
return $result;
}
// 将任务结果返回给主控节点
function sendTaskResult($result) {
$queue = new RabbitMQ();
$queue->push($result);
}
Copy after login
- Distributed data processing
In distributed analysis and decision-making, data processing is an important link . Due to the large amount of data, we need to fragment the data and assign it to different working nodes for processing.
The following is a simple PHP code example:
<?php
// 主控服务节点代码
// 将数据分片后发送到消息队列
function sendShardedDataToQueue($data) {
$queue = new RabbitMQ();
foreach ($data as $shard) {
$queue->push($shard);
}
// 发送完成后,发送一个结束标记
$queue->push('end');
}
// 工作节点代码
// 从消息队列中获取分片数据并处理
function processDataFromQueue() {
$queue = new RabbitMQ();
while (true) {
$shard = $queue->pop();
if ($shard == 'end') {
break;
}
// 处理分片数据...
analysisAndDecision($shard);
}
}
Copy after login
- Summary
By properly designing the distributed architecture and distributing and executing tasks through message queues, we can Implement distributed analysis and decision-making in PHP microservices. In the code example, we use RabbitMQ as the message queue. You can also choose other suitable message queue tools according to actual needs. The choice of middleware will affect the performance and stability of the distributed system, so the performance and throughput of different middleware need to be evaluated and tested.
The above introduction is just a simple example. In actual applications, the security of data transmission, node scalability, and fault handling also need to be taken into consideration. I hope this article can provide you with some reference and help in implementing distributed analysis and decision-making in PHP microservices.
The above is the detailed content of How to implement distributed analysis and decision-making in PHP microservices. For more information, please follow other related articles on the PHP Chinese website!