


How to format the output of employee attendance data through PHP?
How to format the output of employee attendance data through PHP?
Attendance is an important part of enterprise management. Monitoring and counting employees' working hours and work conditions can help enterprises improve work efficiency and scientific management. In practical applications, we often need to output employee attendance data in a certain format to facilitate manual analysis and machine processing. This article will introduce how to format the output of employee attendance data through PHP and give corresponding code examples.
1. Preparation
Before starting, we need to prepare the source data of employee attendance data. Generally speaking, attendance data includes the employee's name, department, attendance date and attendance time, which can be stored in the database. Here, we assume that there is already a data table named attendance
, with the following structure:
CREATE TABLE attendance ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50) NOT NULL, department VARCHAR(50) NOT NULL, date DATE NOT NULL, time TIME NOT NULL );
2. Connect to the database
First, we need to connect to the database to obtain attendance data. You can use PHP's built-in PDO extension to connect to the MySQL database. The following is the code to connect to the database:
<?php $dsn = "mysql:host=localhost;dbname=database"; $username = "username"; $password = "password"; try { $pdo = new PDO($dsn, $username, $password); } catch (PDOException $e) { die("数据库连接失败:" . $e->getMessage()); }
In actual application, database
, username
and password
need to be replaced with the correct database name ,user name and password.
3. Query data
After connecting to the database, we can execute the query statement to obtain the attendance data. The following is a code example to obtain data:
<?php $sql = "SELECT * FROM attendance"; $stmt = $pdo->query($sql); $attendanceData = $stmt->fetchAll(PDO::FETCH_ASSOC);
The above code will query all the data in the attendance
table and store it in the attendanceData
array.
4. Formatted output
After obtaining the data, we can format the attendance data and output it according to the needs. The following is a code example that groups attendance data by department and sorts it by date and time:
<?php // 按照部门分组 $departmentData = []; foreach ($attendanceData as $attendance) { $department = $attendance['department']; if (!isset($departmentData[$department])) { $departmentData[$department] = []; } $departmentData[$department][] = $attendance; } // 按照日期和时间排序 foreach ($departmentData as &$department) { usort($department, function ($a, $b) { return strcmp($a['date'] . $a['time'], $b['date'] . $b['time']); }); } // 格式化输出 foreach ($departmentData as $department => $attendances) { echo "$department 考勤数据: "; foreach ($attendances as $attendance) { $name = $attendance['name']; $date = $attendance['date']; $time = $attendance['time']; echo "姓名:$name 日期:$date 时间:$time "; } echo " "; }
The above code groups the attendance data by department and sorts the attendance data of each department by date and time. Sort and then format the output.
Summary
Through the above steps, we can format and output employee attendance data through PHP. In practical applications, the output can be further processed and optimized according to specific needs. I hope this article can help you understand and apply the formatted output of employee attendance data.
The above is the detailed content of How to format the output of employee attendance data through PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


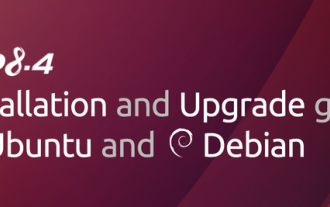
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
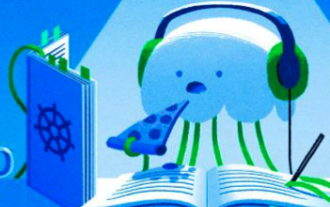
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
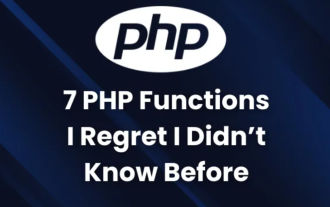
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
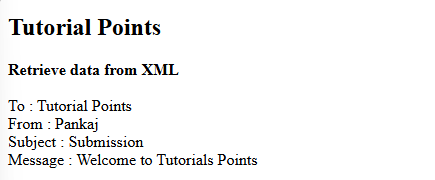
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
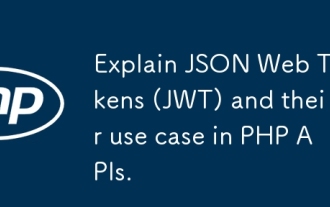
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
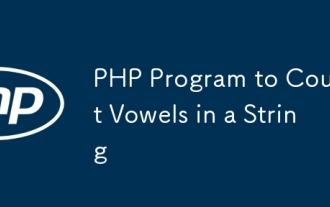
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
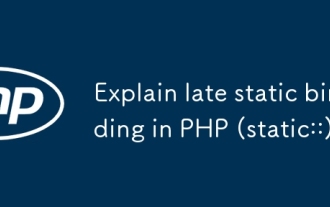
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
