


How to use PHP and Vue to implement the automatic shipping function of warehouse management
How to use PHP and Vue to implement the automatic shipping function of warehouse management
With the rapid development of e-commerce, warehouse management has become a must for many e-commerce companies. One of the links. The automatic shipping function can reduce manual intervention and improve work efficiency, so it is favored by many companies. This article will introduce how to use PHP and Vue to implement the automatic shipping function of warehouse management, and give specific code examples.
1. Demand analysis
Before implementing the automatic shipping function, we need to conduct demand analysis first. The specific requirements are as follows:
- The warehouse management system needs to be able to obtain order information from the order system.
- According to the order information, automatically select the appropriate warehouse and logistics channel for delivery.
- After shipment, the inventory information needs to be updated in real time and the shipment status is sent to the order system.
2. Back-end implementation (PHP)
In the process of PHP back-end implementation, we need to use the following technologies and tools:
- PHP framework: You can choose Laravel, CodeIgniter, etc.
- Database: Choose a suitable relational database, such as MySQL.
- HTTP request: Use PHP's curl library to make HTTP requests.
- Order system interface: According to the specific situation, interface with the developer of the order system.
The specific implementation steps are as follows:
- Create a controller named OrderController, and write a method named getOrders in it to obtain orders from the order system information.
- In the getOrders method, send a request to the order system through an HTTP request and obtain the JSON data of the order information.
- Parse the returned JSON data and save it to the local database.
- In the delivery logic of the warehouse management system, based on the order information, select the appropriate warehouse and logistics channel for delivery.
- After successful delivery, update the inventory information and send the delivery status to the order system.
The following is a simple PHP code example that demonstrates how to implement the function of obtaining order information from the order system:
class OrderController extends Controller { public function getOrders() { $url = 'http://order-system.com/api/orders'; // 订单系统接口地址 $apiKey = 'YOUR_API_KEY'; // 订单系统的API密钥 $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_HTTPHEADER, [ 'Authorization: Bearer ' . $apiKey, 'Content-Type: application/json' ]); $response = curl_exec($curl); curl_close($curl); $orders = json_decode($response, true); // 将订单信息保存到数据库中 foreach ($orders as $order) { Order::create([ 'order_id' => $order['order_id'], 'customer_name' => $order['customer_name'], 'total_amount' => $order['total_amount'], // 其他字段 ]); } return response()->json(['message' => 'Orders imported successfully']); } }
3. Front-end implementation (Vue)
In Vue In the process of front-end implementation, we need to use the following technologies and tools:
- Vue CLI: a scaffolding used to quickly build Vue projects.
- Vue Router: used to implement front-end routing functions.
- Axios: used to make HTTP requests.
The specific implementation steps are as follows:
- Create a new Vue project using Vue CLI.
- Set routing and add a router-view in App.vue to display different pages.
- Create a component named OrderList.vue to display the imported order list.
- In the OrderList component, use Axios to send a request to the backend to obtain the imported order list.
- Render the obtained order list to the page.
The following is a simple Vue component example that demonstrates how to implement the function of displaying the imported order list:
<template> <div> <h2 id="Order-List">Order List</h2> <table> <thead> <tr> <th>Order ID</th> <th>Customer Name</th> <th>Total Amount</th> </tr> </thead> <tbody> <tr v-for="order in orders" :key="order.order_id"> <td>{{ order.order_id }}</td> <td>{{ order.customer_name }}</td> <td>{{ order.total_amount }}</td> </tr> </tbody> </table> </div> </template> <script> import axios from 'axios'; export default { data() { return { orders: [] }; }, mounted() { this.getOrders(); }, methods: { getOrders() { axios.get('/api/orders') .then(response => { this.orders = response.data; }) .catch(error => { console.error(error); }); } } }; </script>
4. Summary
This article introduces how to use PHP and Vue to implement the automatic shipping function of the warehouse management system, and specific code examples are given. In actual project development, the choice of technology stack may differ based on specific needs, but the basic ideas and implementation methods are similar. Through reasonable planning and development, the efficiency of warehouse management can be improved while improving the user experience. I hope this article will be helpful in implementing the automatic shipping function of warehouse management.
The above is the detailed content of How to use PHP and Vue to implement the automatic shipping function of warehouse management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
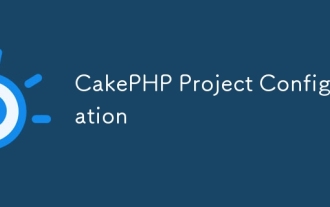
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
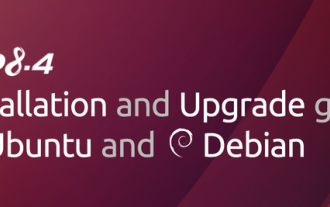
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
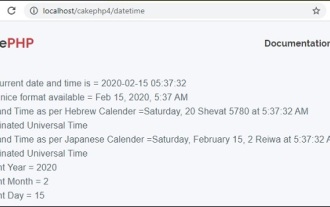
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
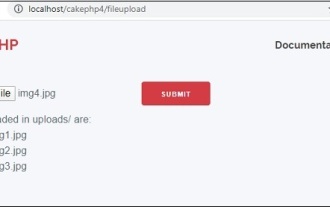
To work on file upload we are going to use the form helper. Here, is an example for file upload.
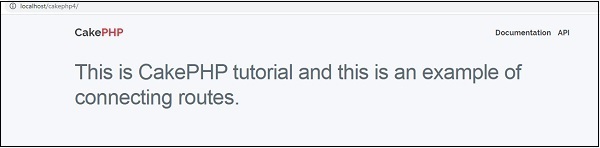
In this chapter, we are going to learn the following topics related to routing ?
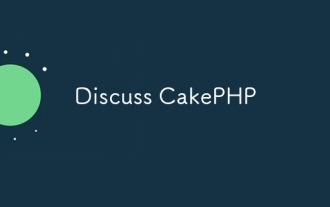
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
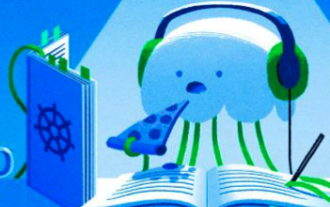
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
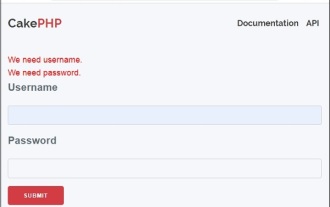
Validator can be created by adding the following two lines in the controller.
