How to use PHP and Vue to implement data filtering function
How to use PHP and Vue to implement data filtering function
In web development, data filtering is a common requirement. This article will introduce how to use PHP and Vue framework to implement a simple data filtering function, and provide specific code examples.
1. HTML structure
First, we need to create an HTML form to enter filter conditions. Suppose we have a table with student information, including the student's name, age, and grades. We can create the following HTML structure:
<div id="app"> <form> <label>姓名:</label> <input type="text" v-model="filter.name"> <br> <label>年龄:</label> <input type="number" v-model.number="filter.age"> <br> <label>成绩:</label> <input type="number" v-model.number="filter.score"> <br> <button type="button" @click="filterStudents">筛选</button> </form> <table> <tr> <th>姓名</th> <th>年龄</th> <th>成绩</th> </tr> <tr v-for="student in filteredStudents"> <td>{{ student.name }}</td> <td>{{ student.age }}</td> <td>{{ student.score }}</td> </tr> </table> </div>
In the above code, we use Vue's two-way binding function (v-model) to bind the input value to the filter object in the Vue instance. When the button is clicked, the filterStudents method will be called to filter and the filter results will be displayed in the table. Note that the v-for instruction is used in the tr tag to traverse the filteredStudents array and render each student's information.
2. Vue instance
Next, we need to create a Vue instance, obtain student information through Ajax requests, and implement the filtering function. We can write the code for the Vue instance in the following way:
new Vue({ el: '#app', data: { students: [], // 学生信息数组 filter: { // 筛选条件 name: '', age: null, score: null } }, computed: { filteredStudents() { // 根据筛选条件过滤学生信息 return this.students.filter(student => { let nameMatch = student.name.toLowerCase().includes(this.filter.name.toLowerCase()); let ageMatch = this.filter.age ? student.age === parseInt(this.filter.age) : true; let scoreMatch = this.filter.score ? student.score === parseFloat(this.filter.score) : true; return nameMatch && ageMatch && scoreMatch; }); } }, methods: { filterStudents() { // 筛选学生信息 // 发送Ajax请求获取学生信息,这里假设数据已经存在 axios.get('/api/students') .then(response => { this.students = response.data; }) .catch(error => { console.error(error); }); } }, mounted() { // 初始化时获取学生信息 this.filterStudents(); } });
In the above code, we use the computed property of Vue to dynamically filter student information when the filter object changes. The computed attribute filteredStudents will filter based on the conditions in the filter object and only display student information that meets the conditions.
The filterStudents method in the methods attribute uses the axios library to send Ajax requests to obtain student information. This assumes that the data already exists and is displayed in the students array.
In the mounted function, we call the filterStudents method to obtain student information during initialization.
3. PHP backend
Finally, we need to process the Ajax request in the PHP backend and return student information. The following is a simple PHP sample code:
<?php // 假设我们已有学生信息的数组 $students = [ ['name' => '张三', 'age' => 18, 'score' => 90], ['name' => '李四', 'age' => 20, 'score' => 85], ['name' => '王五', 'age' => 19, 'score' => 95] ]; // 处理Ajax请求 if ($_SERVER['REQUEST_METHOD'] === 'GET' && $_SERVER['REQUEST_URI'] === '/api/students') { header('Content-Type: application/json'); echo json_encode($students); exit; } ?>
In the above PHP code, we assume that there is already an array $students of student information. When a GET request is received and the request URI is /api/students, we return the JSON format data of the student information.
So far, we have implemented the function of data filtering using PHP and Vue. By using a Vue instance on the front end to set filter conditions, and processing Ajax requests on the back end to return qualified student information, we can simply implement the data filtering function.
Summary
This article introduces how to use PHP and Vue framework to implement data filtering function, and provides specific code examples. During the implementation process, you need to understand the concepts of Vue's two-way binding and computed properties, as well as the way data is processed on the PHP backend through Ajax requests. This method can be applied to various web development scenarios, and I hope it can help readers better understand and apply front-end and back-end data filtering technologies.
The above is the detailed content of How to use PHP and Vue to implement data filtering function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


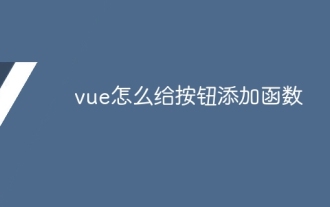
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
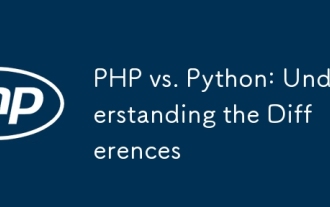
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
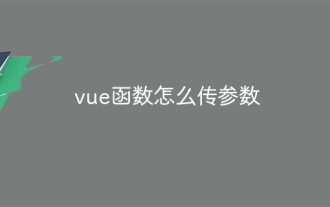
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
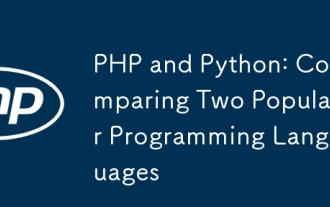
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
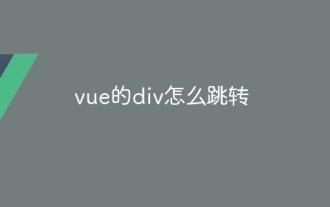
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
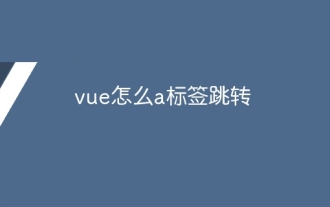
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
